Advanced C++ Programming and Algorithms
A Quick Guide to Encapsulation in C++
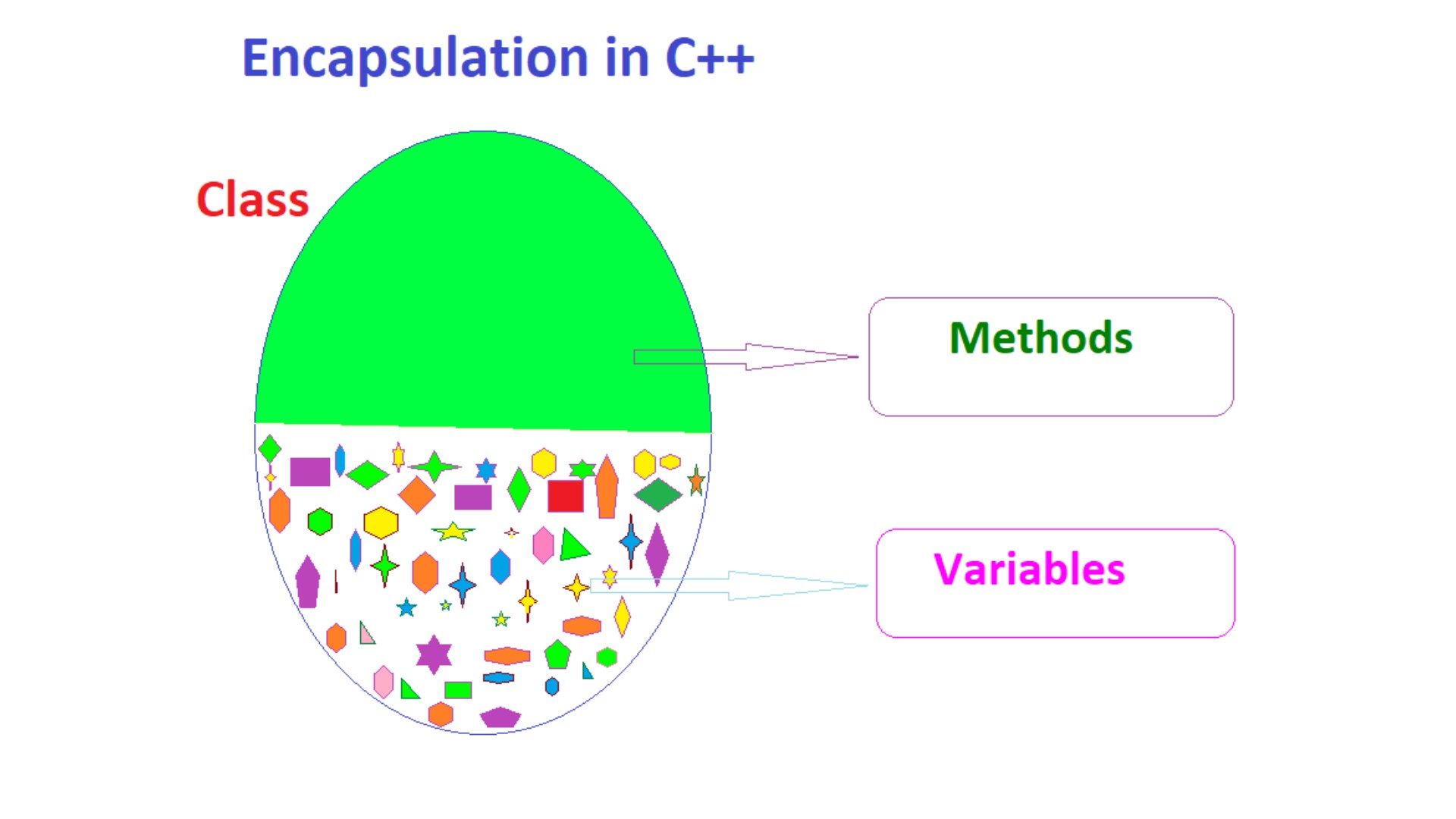
Introduction
Encapsulation is one of the fundamental concepts in OOP that bundles data and associated methods that operate on that data into a single block called CLASS. It is also seen as a pathway for restricting direct access to some data and methods associated with a class (which leads to DATA HIDING). In other words: Encapsulation is about wrapping data and methods into a single class and protecting it from outside intervention.
The word “Encapsulation” can be thought of as a derived form of the word encapsulate where we encapsulate data members (variables) and associated functions (methods) in a class.
“The true value of encapsulation is recognized in an environment prone to change. If our code is well-encapsulated, we can better manage risk in the event of a requirement change. By encapsulating the code, we are less likely to change insignificant parts (for us) of the code.”
ENCAPSULATION IN C++
encapsulation in C++ can be implemented using class and access specifiers.In C++, the implementation of encapsulation has two steps: first, labeling data members as private using the private access specifier, and second, tagging the member function that manipulates data members as public.
EXAMPLE IN C++
ADVANTAGES OF ENCAPSULATION
The main advantage of using Encapsulation is to hide the data from other methods. By making the data private, these data are only used within the class, but these data are not accessible outside the class.
- Protects data from unauthorized users
- This concept is applicable in the marketing and finance sector, where there is a high demand for security and restricted data access to various departments.
- Encapsulation helps us in binding the member functions and data of a class.
- Encapsulation also helps us make code flexible, which is easy to change and maintain.
Conclusion
Encapsulation is a fundamental concept in object-oriented programming. It essentially means binding variables and methods together into a single unit and preventing them from being accessed by other classes.