Advanced C++ Programming and Algorithms
How to Perform Insertion Sort in C++?
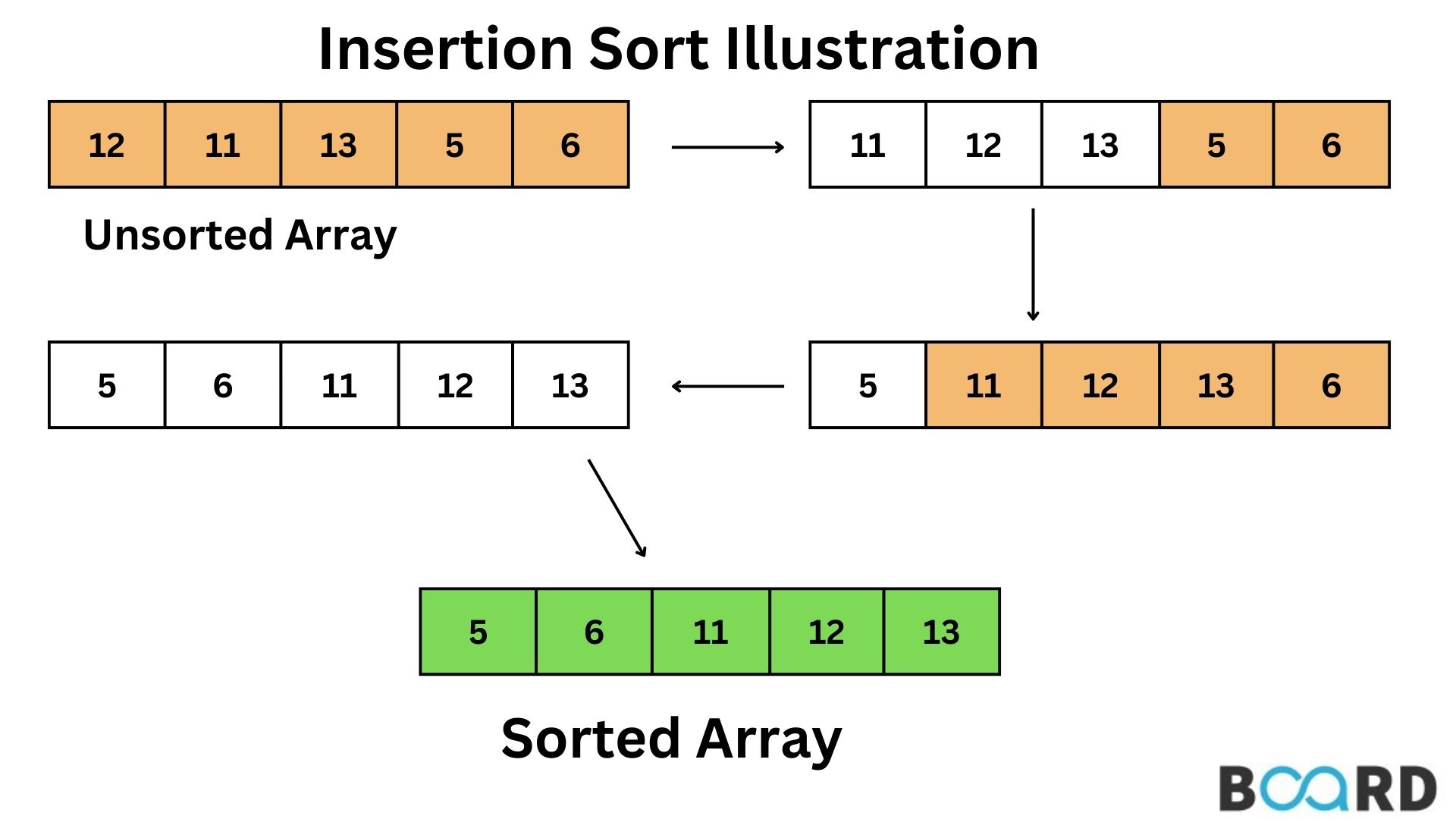
Introduction
In this article, we will learn about Insertion Sort. It places an unsorted element in the proper location after each iteration. Insertion sort functions similarly to how we sort the cards in our hands when playing cards. Assuming the first card is already sorted, we choose an unsorted card next. The unsorted card is positioned on the right if it is larger than the card being held on the left if not. Other unsorted cards are taken in the same manner and placed in the appropriate spot. A similar approach is used by insertion sort.
One of the simplest algorithms, with straightforward implementation, is this one. For small data values, insertion sort is generally effective. Insertion sort is adaptive in nature, so it is suitable for data sets that have already undergone some degree of sorting. Now we will be learning about its working.
In order to rank an array of size N ascendingly
- Over the array, iterate from arr[1] to arr[N].
- Comparing the current (key) element to the previous one.
- Compare the key element to the previous elements to see if it is smaller. In order to make room for the substituted element, move the larger elements up one position.
Implementation of Insertion Sort
Output
Time Complexity
O(N^2), Suppose, an array is in ascending order, and you want to sort it in descending order. In this case, worst case complexity occurs. Each element has to be compared with each of the other elements so, for every nth element, (n-1) number of comparisons are made.
Thus, the total number of comparisons = n*(n-1) ~ n2
Auxiliary Space
O(1), Space complexity is O(1) because an extra variable key is used.
Insertion sort is used when there are few items. It can also be helpful when the input array is nearly sorted and only a small number of the large array's elements are misplaced.
In the case of using Insertion sort for the Linked list, Below is a simple insertion sort algorithm for the linked list.
- Create an empty sorted (or result) list
- Traverse the given list, and do the following for every node.
- Insert the current node in a sorted way in the sorted or result list.
- Change the head of the given linked list to the head of the sorted (or result) list.