Advanced C++ Programming and Algorithms
OOPs (Object Oriented Programming) in C++
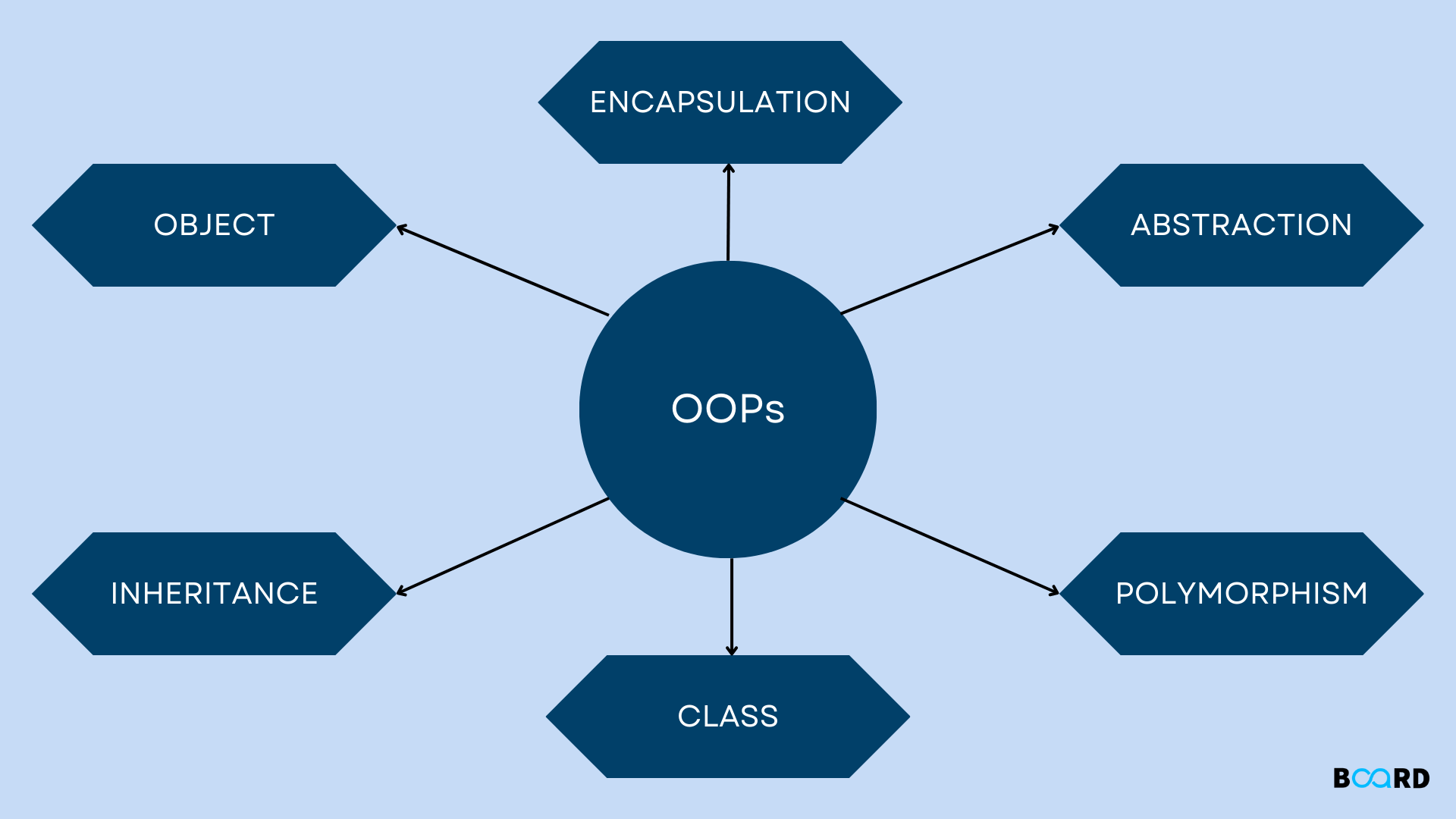
Introduction
In the C language, our program was organised around logic and functions, but in the object-oriented programming language, C++, our program is organised about objects rather than logic and functions. By dividing data into two memory blocks, namely data and functions, this method makes the code modular and versatile. The primary focus of object-oriented programming is on classes and objects. Data is modelled in OOPs as objects with properties and functionalities. We shall discover the many OOPs ideas in C++ in this blog.
In this article we are covering the foundational ideas of OOPs that serve as its building pieces.
Class
You may think of a class as a container for functions and data variables.
A class is an object-only data type that is specified by the user and holds member functions and variables together. It is a template by which objects will be created and they will all showcase the features of the class definition.
Object
The class's instances are objects.
Classes are used to build objects. The same process used to declare variables also applies to declaring objects. A real-world entity that physically exists in the world and has some behaviour and state is referred to as an object. Eg. chair, a table, a dog, a person, a car, etc.
Abstraction
It is a way of shielding the user from unimportant information like the implementation.
Consider a scenario where someone is downloading and uploading files. He is aware of the appropriate alternatives to select. He will click the appropriate button to begin uploading or downloading the material. Your phone will display if the file has been downloaded or uploaded. As it is irrelevant to you, what occurs on the server when you press send is concealed from you. When someone clicks upload or download, just the section the user wants to view is displayed; the background activity that occurs is concealed from him.
Encapsulation
keeping the information and functionality in a single, capsule-like entity.
Through the process of encapsulation, data and function are combined into a single, capsule-like entity. This is done to prevent members outside the class from accessing private information. In order to accomplish encapsulation, we keep all class data members private and construct public methods that allow us to access or modify the values of these data members.
Inheritance
A derived class inherits all the data members and functions from a base class.
The method through which a relationship exists between two base classes and a derived class is known as inheritance. The base class's attributes and characteristics are transferred to the derived class. The class from which the characteristics are derived is known as the base class, and the class from which the features are inherited is known as the derived class. The classes Male and Female, for instance, are derived classes from the basic class HumanBeing.
Polymorphism
Redefining function behaviours or providing alternate function behaviours is Polymorphism
Polymorphism redefines how something functions by either altering how it is carried out or by altering the components used to carry it out. A person is capable of juggling many roles at once. A woman simultaneously serves as a mom, sister, wife, and worker. Polymorphism is the term for this. Two crucial ideas are included in polymorphism.
- Operator Overloading: Operator overloading occurs when an operator behaves differently in various situations.
- Function Overloading: Function overloading refers to when a function exhibits diverse behaviour in various contexts. This implies that a function with the same name in two distinct classes will provide two entirely different results.
As you can see, the program uses the same function sum(), but it takes a different amount of parameters and various types of inputs. Two integers make up the first sum(), two double numbers make up the second sum(), and three integers make up the third sum().
Advantages of OOPs
- OOPs enable code reuse and increase the use of preexisting classes.
- Because classes and objects are used in OOPs, code maintenance is simple and doesn't need any reorganisation.
- Additionally, it aids in data concealment, protecting the data and information from leakage or exposure.
- Implementing object-oriented programming is simple.
You should now have a better understanding of the necessity for OOP development while handling medium-to-large scale projects. In this blog we went over the fundamentals of OOPs, such as polymorphism, inheritance, encapsulation, etc., which are the building blocks for the C++ OOP architecture. You also learnt about polymorphism and inheritance examples, as well as the benefits of C++ OOPs.