Advanced C++ Programming and Algorithms
OOPS Polymorphism with C++
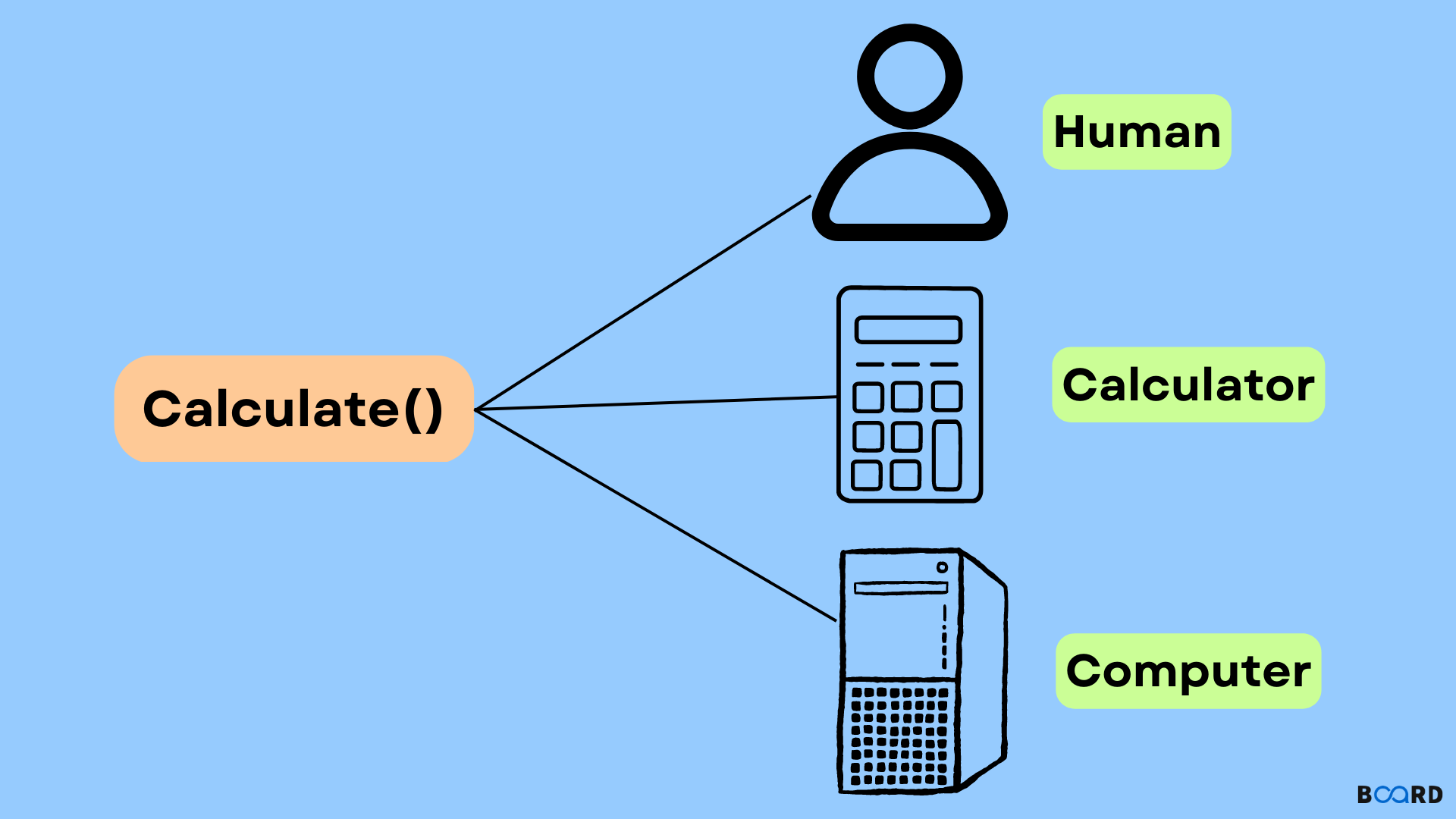
What is Polymorphism in OOPS?
In the real world, it often happens that one person is doing multiple tasks. For example, you work as an employee in a company and at another time, you play the role of a son in your home. However, you don’t change your name every time. You just put different attires for different roles. This concept is not restricted to the Real World, it is also applied to Programming. In this article, we will learn what is Polymorphism in OOPs.
Polymorphism, which means ‘One name and many forms’ is one of the seven principles of Object Oriented Programming. It simply means that one function can be used for multiple operations, without declaring a different function. Since the function's name is the same for all use cases, it’s become crucial to know how to identify the operation of the function in different cases.
As you already know the standard paradigm of Programming includes two stages- Compilation and Execution. So, the function’s behavior may be known during the compilation stage or execution of the code.
This differentiates the type of Polymorphism implemented in your code. Before learning what is Polymorphism in OOPS, just go through the diagram below to understand its classification. Let’s discuss the first type of Polymorphism.
Compile Time Polymorphism
Compile Time Polymorphism means function definitions are distinguished at the time of compilation. It is applied using the concept of Overloading and is further classified as Function Overloading and Operator Overloading.
Function Overloading
In Function Overloading, you can define more than one function with the same name but different arguments. These different arguments help the compiler to identify which function definition should be called.
Let’s understand this with a C++ implementation.
Here the display function has different arguments in all the cases using which compiler knows which function definition to call. The code as following output:
An important point here is you have to use different arguments in the function definitions. If you want to use the same arguments, then you should use the different order of passing arguments as shown below.
Like Function Overloading, Operator Overloading is also an important concept while learning what is Polymorphism in OOPS as Object Oriented Programming allows us to also use operators for this purpose.
Operator Overloading
Operator Overloading allows us to redefine how operators work under normal conditions. Before learning what is Polymorphism in OOPS, you should be aware of the basic operators used in C++. There are three types of Operators:
- Unary Operator
- Binary Operator
- Ternary Operator
However, in Operator Overloading, the following operators cannot be overloaded:
- Scope Operator (::)
- Member Access Operator (.)
- Asterisk (*)
- Ternary Operator (?:)
Now, we will look into the Unary and Binary Operator Overloading under Polymorphism.
For Unary Operator Overloading, suppose you want to redefine the (++) operator and increase the value by 100, instead of 1. Then, you have to define the (++) operator as shown below and then call the operator using the object of the class.
As shown in the below output, the value is incremented by 100.
Similarly, Binary Operator Overloading is done. Normally, the Addition (+) operator is used to add two built-in Data Types. But, using Operator Overloading, can be used to add two numbers of different data types. Below is the C++ implementation of Binary Operator Overloading.
The output of the code is:
Now, to completely understand what is polymorphism in OOPS, we have to understand the second type i.e. Run Time Polymorphism.
Run Time Polymorphism
In this type of Polymorphism, the function definitions are distinguished during the execution of the code. Run Time Polymorphism is achieved by implementing the virtual function. To understand the Virtual Function, you should be familiar with Inheritance in OOPS
A Virtual Function is a member function that is declared in the Parent class and then it is redefined in the Child Class.
At the time of calling the function, instead of an object, a pointer of the base class is used to call the function. Which function is called is decided by which object is referenced by the pointer. This is decided later during run time it is called Run Time Polymorphism. The following code shows Run Time Polymorphism using Virtual Functions.
The output of the code is shown below.
Now, it would be clear to you what is polymorphism in OOPS. Moreover, virtual functions are mainly used to use the base class pointers which can be used to call the derived class methods without knowing the Derived class object.