Advanced C++ Programming and Algorithms
OOPs Abstraction in C++
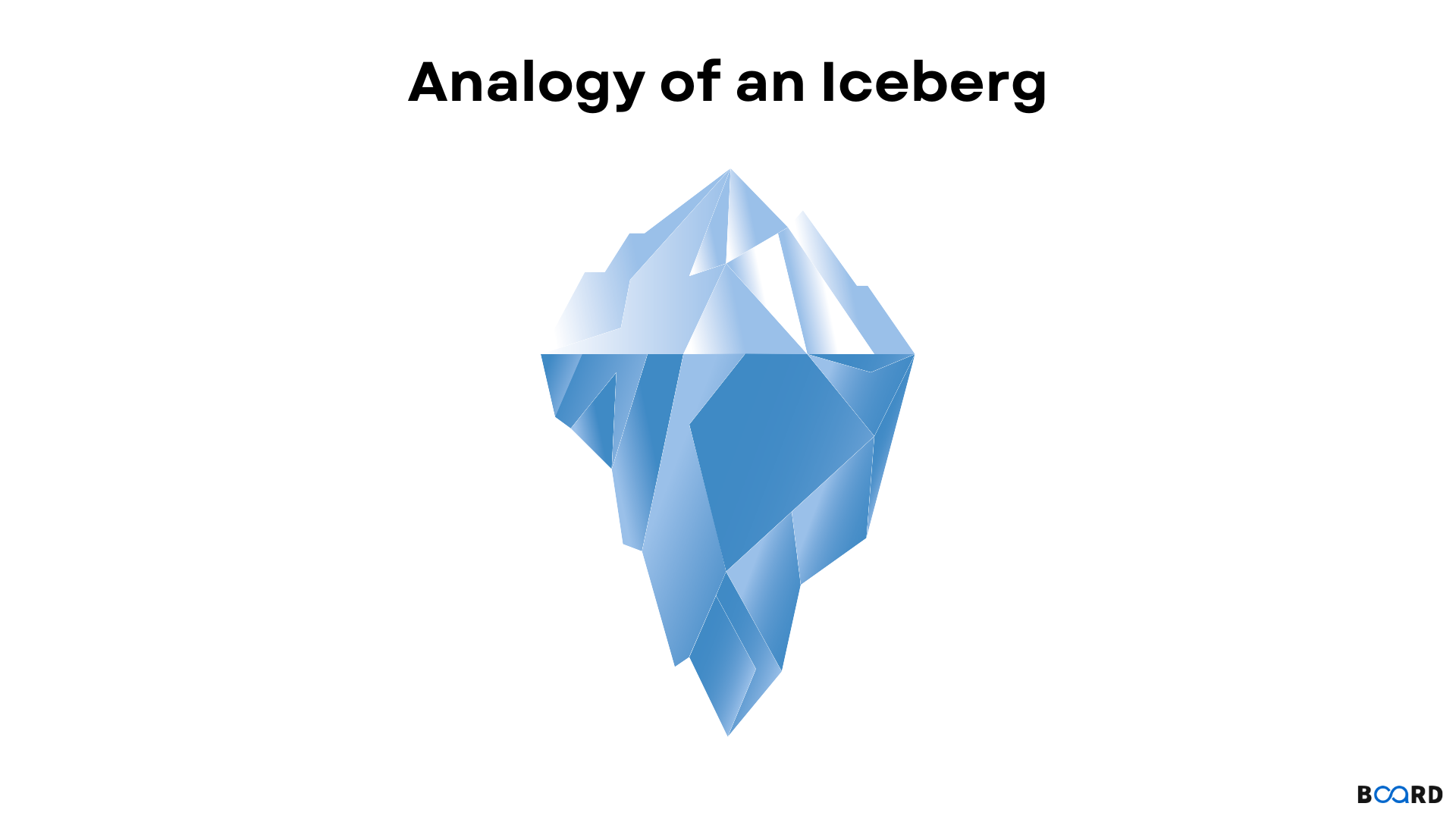
Introduction
Abstraction is one of the most important concepts of object-oriented programming. It refers to showing only relevant information to the outside world. In simple words, we can say it means hiding any background information from the outside world.
Data abstraction refers to showing only relevant information about data to the outside world or hiding the implementation.
Let us consider a real-life example:
When we drive a car and there is another vehicle coming from the front so we apply the brake. Now we are only concerned about applying the brake. We don’t go deep into how the brake mechanism works internally like the brake box, brake shoe, etc. This is what abstraction is.
Types of abstraction
There are two types of abstraction:
1. Data abstraction: This type of abstraction is more concerned with showing the required information regarding the data and hides any irrelevant information from the user’s perspective.
2. Control abstraction: This type of abstraction portrays only relevant information regarding the implementation and hides any irrelevant information.
Abstraction with classes
Abstraction in C++ can be implemented using classes. A class allows us to group properties (data members) and behavior (implementation) with the help of access specifiers. A class is free to choose which data members should be available to the outside world and which should not.
Abstraction with header files
We can also visualize abstraction in header files. For example, we have a max() function present in the <algorithm> header file that compares a set of elements and gives us the maximum of all. It is underlying to note here that we are only concerned about passing a set of values and this function returns us the maximum value out of those. We don’t worry about the functions’ implementation and this is what abstraction is.
Abstraction with access specifiers
Access specifiers are important to implement abstraction in C++. They can be used in order to impose restrictions on the class entities. Let us consider an example below,
- Those data members or member functions that are declared using public access specifier can be accessed from anywhere in the program.
- Those data members or member functions that are declared using private access specifier can be accessed only from within the class. They cannot be accessed from outside the class.
Abstraction can be implemented easily using the methods discussed above. The members that are responsible for the internal implementation can be set to private in a class and that information will be hidden from the outside world. Also, the information which is required to be shared with the outside world can be made using public access specifier.
Let us consider an example,
Example
Outside the class, data1 and data2 are not accessible directly as they are declared as private. This shows the abstraction.
Output:
Conclusion
As you can see, we displayed data1 and data2 using display() method declared under Abstraction class.