Advanced C++ Programming and Algorithms
Virtual Base Class in C++
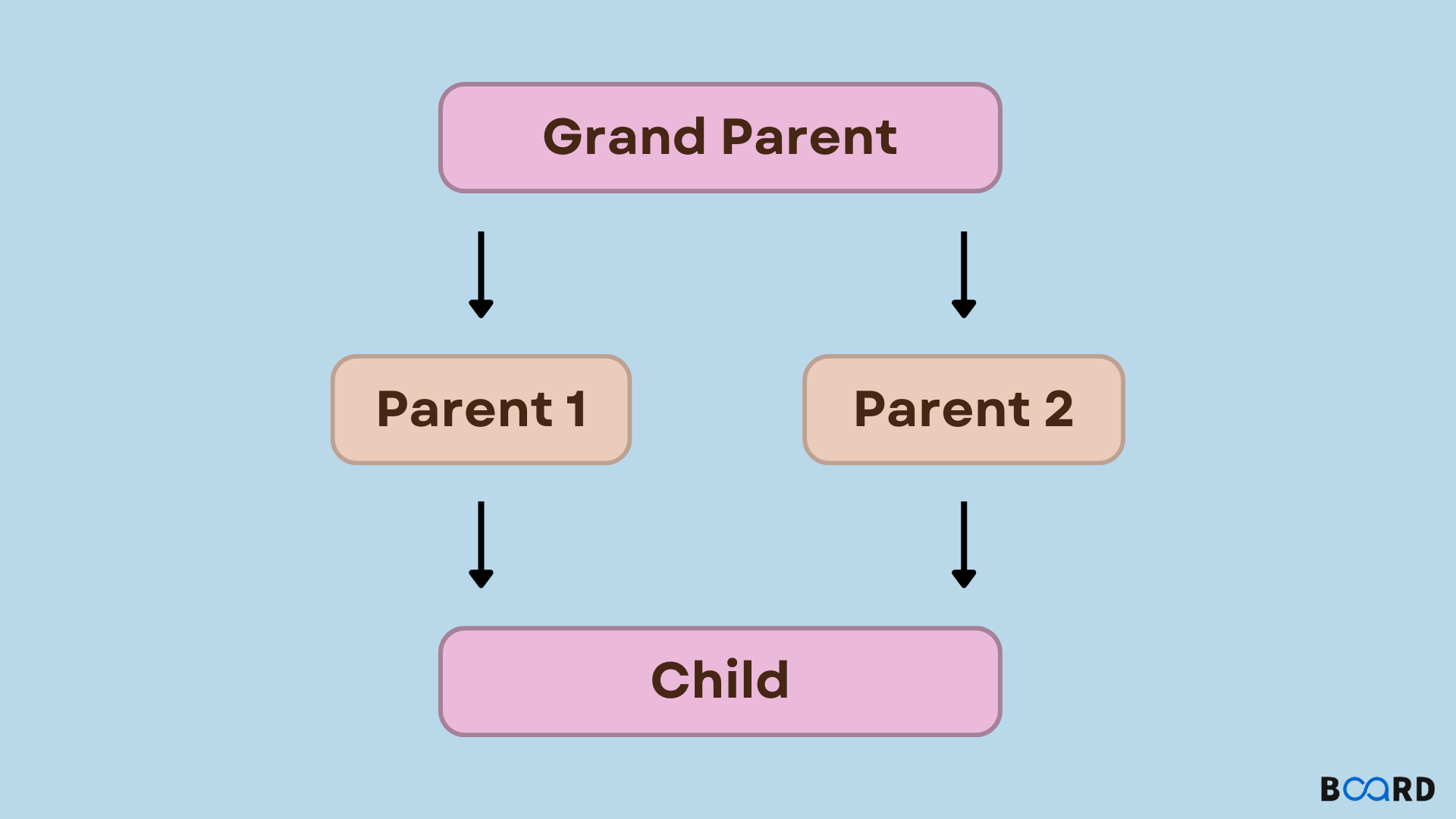
Introduction
Virtual base classes in C++ are used to prevent multiple instances of a given class from appearing in an inheritance hierarchy when using multiple inheritances.
Base classes are the classes from which other classes are derived. The derived(child) classes have access to the variables and methods/functions of a base(parent) class. The entire structure is known as the inheritance hierarchy.
Virtual Class is defined by writing a keyword “virtual” in the derived classes, allowing only one copy of data to be copied to Class B and Class C (referring to the above example). It prevents multiple instances of a class appearing as a parent class in the inheritance hierarchy when multiple inheritances are used.
Need for Virtual Base Class
To prevent the error and let the compiler work efficiently, we’ve to use a virtual base class when multiple inheritances occur. It saves space and avoids ambiguity.
When a class is specified as a virtual base class, it prevents duplication of its data members. Only one copy of its data members is shared by all the base classes that use the virtual base class.
If a virtual base class is not used, all the derived classes will get duplicated data members. In this case, the compiler cannot decide which one to execute.
Syntax
Examples
Example 1
Output:
Example 2
Output: