10 Common Data Structures Every Programmer Must Know
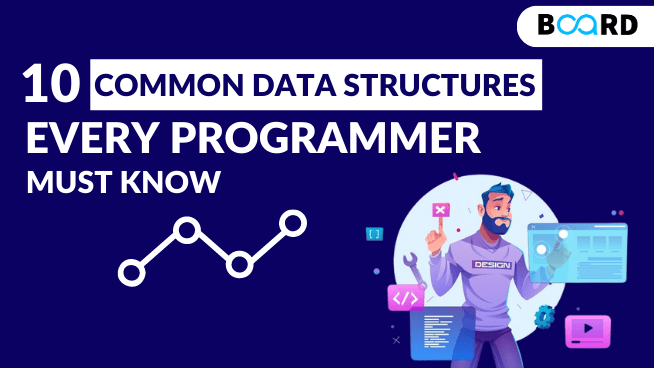
Introduction
Data structures have become an essential topic to ace in every interview. Every aspirant who wants to grasp a good-paying job needs to be well versed in this concept. Data structures help you to learn and implement various algorithms to solve different coding problems. You need to grasp the most prevalent data structures and fill your resumé with in-demand abilities. Algorithms and data structures are the most important disciplines for programmers.
Employers and recruiters look for both runtime and resource efficiency while tackling real-world coding difficulties. Knowing which data structure is optimal for the present solution will improve program performance and cut development time. As a result, most top organizations need a solid comprehension of data structures and put them to the test during the coding interview.
What is Data Structure?
Data structures are code frameworks for storing and organizing data that make it easier to manipulate, navigate, and access data. The way data is gathered, the functionality we may implement, and the relationships between data, are all determined by data structures.
Data structures are employed in practically every computer science and programming aspect, from operating systems to front-end development to machine learning.
Applications of Data Structure
Data structures are tried-and-tested technologies that provide a simple framework for organizing your programs. Data structures can be applied for:
- Large datasets may be managed and used in a variety of ways.
- Search a database quickly for specific information.
- Connect data items in a clear hierarchical/relational manner.
- Streamline and accelerate data processing.
Lists, dictionaries, tuples, and sets are the four built-in data structures in Python. These pre-built data structures provide default methods and make them simple to use. Let's take a look at types of data structures.
10 Data Structures Types to Master
Some of the most significant data structures to be aware of are listed below. This isn't a complete list, and you may play around with other data structures to come up with your own. However, these are the fundamentals that will enable you to pursue a programming and data analysis career.
1. Arrays
An array is a collection of things that are stored consecutively and is one of the most basic data structures. An array includes values or variables of the same data type (known as 'elements') and has a fixed size, therefore, you can't modify the size of an array. The index of each item in an array begins with 0.
Consider an array in the same manner as a weekly pill organiser. It consists of a series of miniature containers, each with its own set of components. Arrays are frequently used as building blocks for more complex data structures. Algorithms for sorting are also based on them.
2. Linked Lists
A linked list is a collection of objects connected in a linear order. This means that data must be accessed in a specific order; random data access is not feasible. Each element is referred to as a 'node', and each node has a key and a reference in a linked list. The pointer points to the next node, which is labeled 'next'. The sequence begins with a 'head', which takes you to the list's first entry. The 'tail' refers to the last item on the list.
You may make a singly linked list, which allows you to traverse each item from the head to the tail in a forward way. A doubly-linked list, on the other hand, may be traversed both forward and backward. Finally, you may make a circular linked list where the tail's next pointer points to the head, and vice versa, and completes a circle. When moving between applications with Alt + Tab, linked lists are utilised to maintain symbol tables (on a PC).
3. Stacks
The function of a stack is roughly identical to what it sounds like. It's similar to stacking items in a tall container.
LIFO (Last In First Out) structures are referred to as stacks. This indicates that the piece that was inserted last is the first to be accessible. You may either 'push' a new element to the top of the stack or 'pop', which deletes the element that was put previously and is now at the top. Stacks are often used in recursion programming to parse and evaluate mathematical statements and implement function calls.
4. Queues
A queue is similar to a stack. However, it is a FIFO (First In First Out) structure rather than a LIFO (Last In First Out) structure. A line of people waiting to enter a building is the easiest way to visualize a queue. The person at the front of the line will be the first to enter the building, while the back will be the last.
In this structure, you may enqueue an element, which means adding it to the end of the queue. You may also dequeue an element, which means removing it from the queue's beginning. Queues are commonly used to manage threads in multithreading and construct priority queuing systems (unsurprisingly).
5. Hash Tables
Each value is associated with a key in a hash table structure, which then stores them. This makes it simple to lookup values using a key quickly. It's a quick and straightforward approach to insert and search for data, regardless of its size, because it makes it simple to distinguish between a group of related things.
If you attend college, for example, you may be given a unique student ID number. This ID number serves as a key to retrieving information about you and your academic record. A hash table maps a data set of arbitrary size to one of a defined size—the hash table—using a 'hash function'. 'Hash values' are the values returned by a hash function.
Hash tables are often used to build database indexes, associative arrays, and a 'set', among other things.
6. Trees
A tree is a non-linear hierarchical data structure, unlike arrays, stacks, queues, and linked lists, which are all linear data structures. Consider it as a firm, with the CEO at the top and department heads at the bottom. Those department leaders have authority over those who report to them, resulting in more nodes. Each parent-child node relationship is represented by one edge (or link) in a tree. From a root to any given node, there must be only one viable path.
Trees are a more complicated data structure frequently employed in artificial intelligence and other complex problem-solving systems. Binary trees, binary search trees, AVL trees, balanced trees, red-black trees, 2-3 trees, and N-ary trees are among the several forms of trees. The binary tree and binary search tree are the most common.
7. Graphs
A tree is essentially a graph in its most basic form. The difference between a graph and a tree is that no rules dictate how nodes are connected with a graph. A network may be seen as a graph. Graphs in coding, like graphs in mathematics, have vertices. Although nodes appear as points on a graph, they are frequently linked to others, forming a web-like pattern. Undirected graphs and directed graphs are two different types of graphs. An adjacency matrix or an adjacency list can be used to represent them.
8. Tries
If you've ever used a search engine and received auto recommendations, you've seen a trie data structure in action. String-related problems can be solved using tries. Because they are prefixes of longer pathways, they are also known as 'prefix trees'.
9. Heaps
A heap is a binary tree in which the parent nodes are compared to their offspring. As a result, the values within the nodes can be structured as needed. Trees can represent heaps; however, binary arrays can also be used. There are two different kinds of piles. The parent's key is less than or equal to the children's keys in a min-heap. The parent's key is larger than or equal to the children's keys in a max heap.
Algorithms that employ heaps to generate priority queues and locate the lowest or highest value in an array are common.
10. Binary Search Tree
A binary search tree is a node-based binary tree data structure. The following properties are used to order the nodes:
- The values in the left subnode are always smaller than those in the parent node.
- Values larger than the parent node are always found in the right subnode.
- Binary search trees will be used in both subnodes.
Many search apps employ binary search trees, and they're also used to figure out which objects in a 3D game need to be drawn. Because hierarchical data is so ubiquitous, this data format is commonly employed in engineering projects.
Types of Data Structures are an essential concept to learn for a data scientist.
Conclusion
Almost every data structure has a variety of applications and purposes. Having a rudimentary grasp of data structures is a crucial first step toward becoming a skilled programmer. For a deep understanding of data structures, enroll for the data structures and algorithms with the C++ course and learn the most powerful programming languages and data structures to solve programming challenges. You'll be fully capable of analyzing and implementing algorithms, as well as be able to select the best algorithm for various situations.
You'll find it easier to learn other programming languages once you've mastered the types of data structure since you'll have a firm foundation to build on. Understanding the most popular data structures and how they function is the initial step, whether you're developing data structures in Java or Python or studying for interview questions.