A Simple Introduction to Recursion
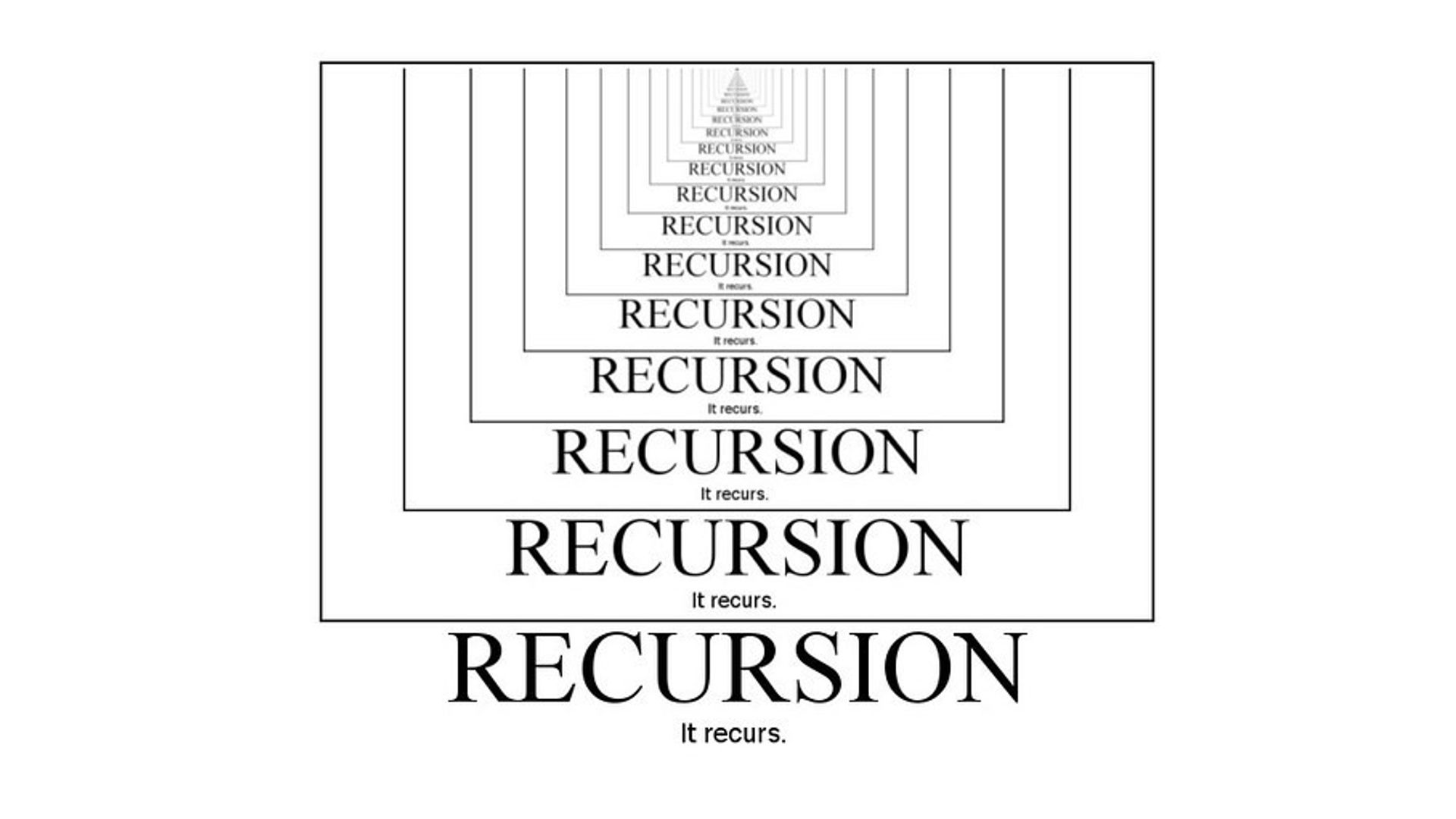
Introduction
Recursion is a C++ method that calls itself repeatedly until a certain condition is met. This technique has a base case and a recursive condition, calling the same function repeatedly. Recursive conditions help repeat code over and over, and base cases help terminate conditions.
Implementation
Recursion can be used to solve almost any problem, and sometimes it really helps. It is generally used when dealing with complex problems or problems that form hierarchical patterns. Solve the original problem through smaller sub-problems.
Developers should pay close attention to recursion. Because it's easy to write functions that never terminate, or consume excessive memory or processing power. However, if written correctly, recursion can be a very efficient and mathematically elegant programming approach.
Recursion consumes more memory because recursive functions add something to the stack for each recursive call and retain the value until the call completes. Recursive functions use a LIFO (LAST IN FIRST OUT) structure as well as a stack data structure.
Example 1: Calculating Factorial
Output:
Enter a non-negative number: 4
Factorial of 4 = 24
Advantages of Recursion
- This makes your code shorter and cleaner.
- Recursion is necessary for problems involving data structures and sophisticated algorithms such as graph and tree traversals.
Disadvantages of Recursion
- It takes up a lot of stack space compared to iterative programs.
- Consume more processor time.
- It can be more challenging to debug compared to equivalent iterative programs.