Working on Queue using STL
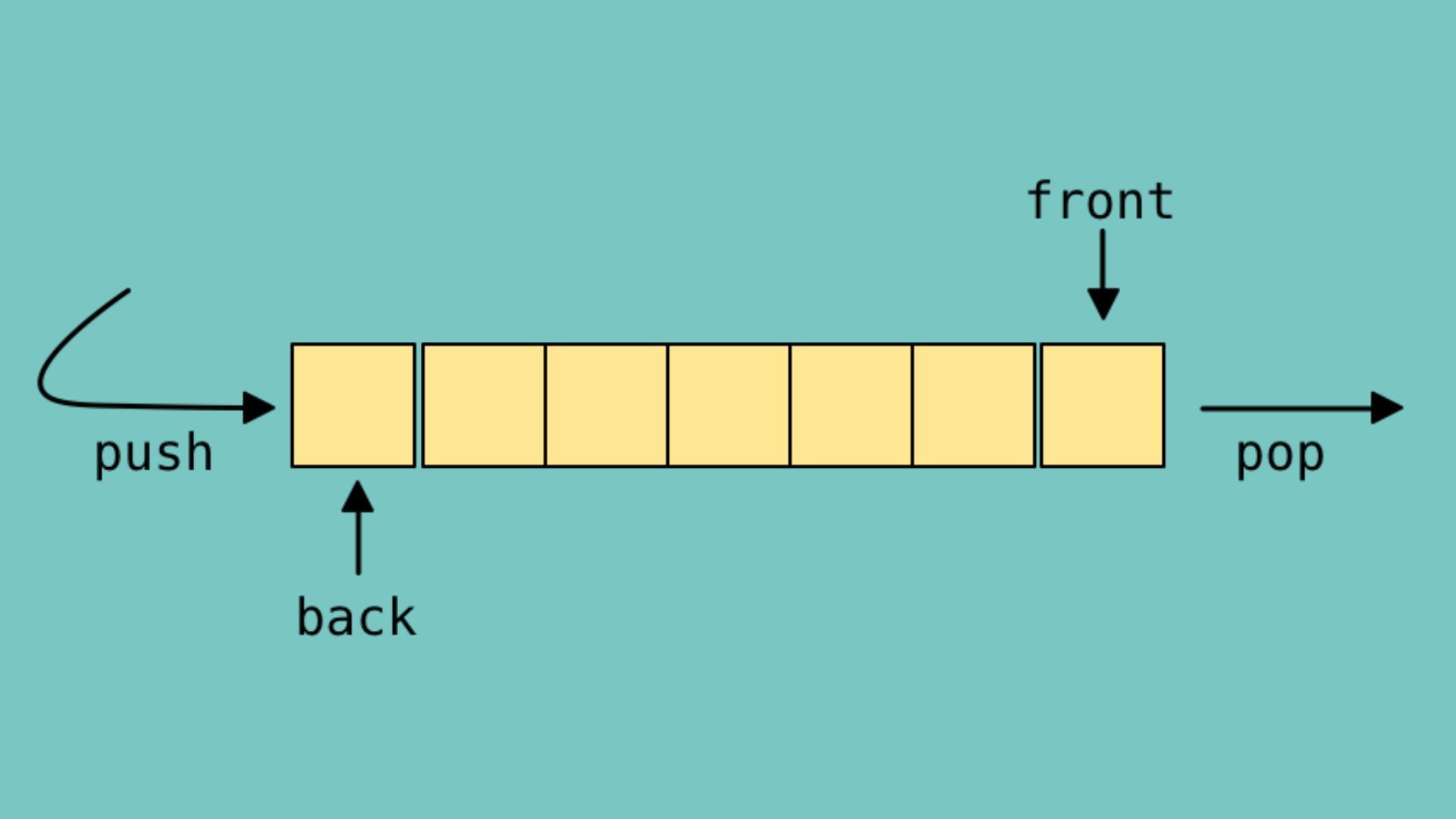
Introduction
In C++, the replication of a queue is done using a queue container; insertion always happens at the end of the queue, and deletion always happens at the beginning. Here is how to define a queue in syntax:
The preceding line will produce an object type queue with the name queue_name.
Member Functions of Queue Container
Some of the Queue Container's frequently utilized STL features include the following:
push function
The element is added to the queue using push(). The queue's back or rear is where the element is inserted.
pop function
By taking one item out of the queue's front position, this technique decreases the queue's size by one. The piece that was entered first is the one that was eliminated. Nothing is returned by pop().
front and back functions
In contrast to back(), front() returns the element at the front of the queue. Notably, unlike pop, both restore the element rather than removing it ().
size and empty functions
While empty() determines if the queue is empty or not, size() returns the number of components that are currently in the queue. If the queue is empty, empty returns true; otherwise, false is returned.
Swap function
The two queue elements are switched via the method swap().
Application of Queue in Data Structure
In situations when the FIFO technique (First In First Out) needs to be applied, a queue data structure is typically employed. Some of the most popular uses for the queue in data structures are the ones listed below:
- Scheduling requests for a single shared resource, such as disc and CPU
- Interruptions from real-time or hardware systems
- Dealing with website traffic switches
- Routers in Networking
- Media player and music queues
Conclusion
We hope this post was helpful to you. You can enhance your ability to work on projects that need knowledge of queue data structures by developing a thorough understanding of the crucial queue data structure idea, its kinds, implementation, and applications.