Binary Tree Code Implementation
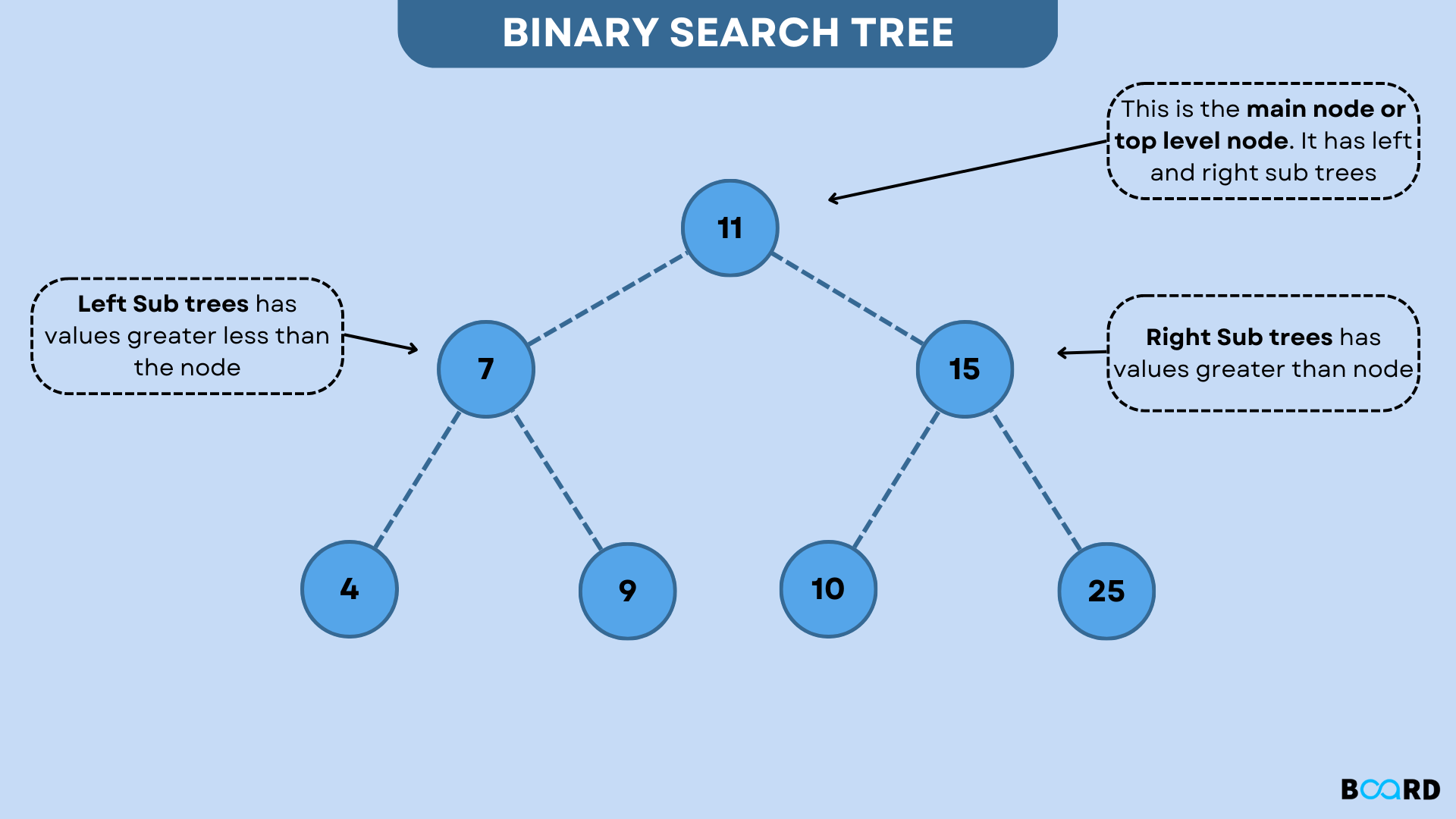
Introduction
Do you know what a binary is? How can we implement binary trees in our favorite programming language? Then don't worry, dear, when Board Infinity is here.
In this article, we will discuss each and everything about the binary tree. We will also look at its implementation and its properties. Let us understand what it is.
What is a Binary Tree?
Each parent node can have a maximum of two children in a binary tree, a type of tree data structure. A binary tree's nodes are made up of these three components:
- Data item
- Address of the left child
- Address of the right child
Let us look at the types of a binary trees.
Types
The types of binary trees are mentioned below:
- Full Binary Tree: An exceptional form of a binary tree called a full binary tree includes either two offspring or none at all for each parent node and internal node.
- Perfectly Binary Tree: A type of binary tree known as a perfect binary tree is one in which every leaf node is at the same level, and every internal node has exactly two child nodes.
- Complete Binary Tree: A complete binary tree is identical to a complete binary tree, with two key exceptions.
- Each level must be fully filled.
- Each component of the leaf must slant to the left.
- A complete binary tree does not necessarily have to be a full binary tree because the final leaf element may not have the right sibling.
- Degenerate or Pathological Tree: A degenerate or pathological tree is one that only has one offspring, either to the left or to the right.
- Skewed Binary Tree: A skewed binary tree is a diseased or degenerative tree in which the right or the left nodes predominate. There are two kinds of skewed binary trees: left-skewed binary trees and right-skewed binary trees.
- Balanced Binary Tree: It is a kind of binary tree in which each node has a height difference between the left and right subtrees that is either 0 or 1.
Let us discuss the applications of binary trees.
Applications
The applications of binary trees are mentioned below:
- In router algorithms,
- For rapid and simple access to data
- To use the syntax tree
- To implement the heap data structure
Now we understand what a binary tree is. So, let us discuss how we can implement the binary tree. Let us look at binary tree code.
Implementation of Binary Tree
Before moving on to the binary tree code, let us look at the representation of the binary tree. A structure that includes two pointers to additional structures of the same type and a data part represents a node in a binary tree.
Here is the implementation of binary tree code.
Output
Conclusion
In this article, we have discussed binary tree code. We have also discussed what a binary tree is and what its applications are. We discussed its several types. Binary trees are an important part of tree data structure and have many real-world applications.