Complete Walkthrough of Stacks in Data Structure
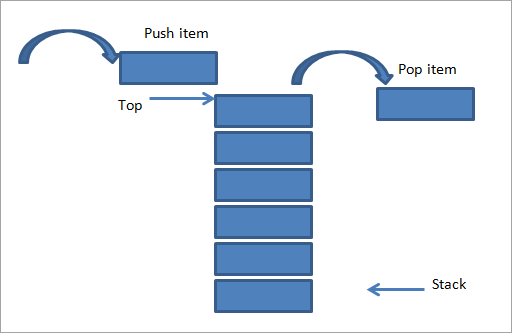
Introduction
You will gain knowledge about the stack data structure in this article, as well as how it is implemented in Python, Java, and C/C++. Stacks are linear data structures that adhere to the Last In First Out rule (LIFO). This implies that the last piece to be added to the stack gets eliminated first. The stack data structure may be compared to a stack of plates stacked one on top of the other.
LIFO Principle of Stack
It basically means that the element that was added last will mandatorily be the element that will have to be removed first and by extension, the element added first will be at the bottom of the stack and will be popped out the last. Programmers use the phrases push and pop to describe the actions of adding and deleting items from stacks.
Stack Primary Operations
We may carry out many activities on a stack using a few fundamental operations.
- Push: Add item to stack's top
- Pop: Delete the item from the stack's top and return it
- IsEmpty: Check if there are elements in the stack
- IsFull: Check if there is any more space in the stack to accommodate more elements
- Peek: Gain access to the top element's value without deleting it
Working of Stack Data Structure
The following is how the processes go:
- The top element on the stack is tracked by a pointer named TOP.
- We initialize the stack with a value of -1 so that TOP == -1 may be used to determine whether the stack is empty.
- When an element is pushed, TOP's value is increased and the new element is positioned in the area that TOP is pointing to.
- When an element is popped, we return the item that TOP referred to and lower its value.
- We determine whether the stack already is full before pushing. We determine whether the stack already is empty before popping.
Code
Time Complexity
The pop and push operations for the array-based version of a stack require constant time, i.e. (1).
Applications of Stack Data Structure
While stack is a straightforward data structure to use, it is incredibly effective. A stack is most frequently used for the following purposes:
- Reverse string - Stack all the letters together, then remove them. You'll receive all letters in reverse due to the stack's LIFO sequence.
- Compiler Design - By transforming the equation to prefix or postfix form, compilers use the stack to figure out the value of equations like 2 + 4 / 5 * (7 - 9)
- Browser History - All of the URLs you've previously viewed are saved in a stack when you use the back button on a browser. A fresh page is put on top of the stack each time you view one. The previous URL is retrieved when you use the back button, which also removes the current URL from the stack.