C++ Fundamentals: Understanding the Basics
Command Line Arguments in C++
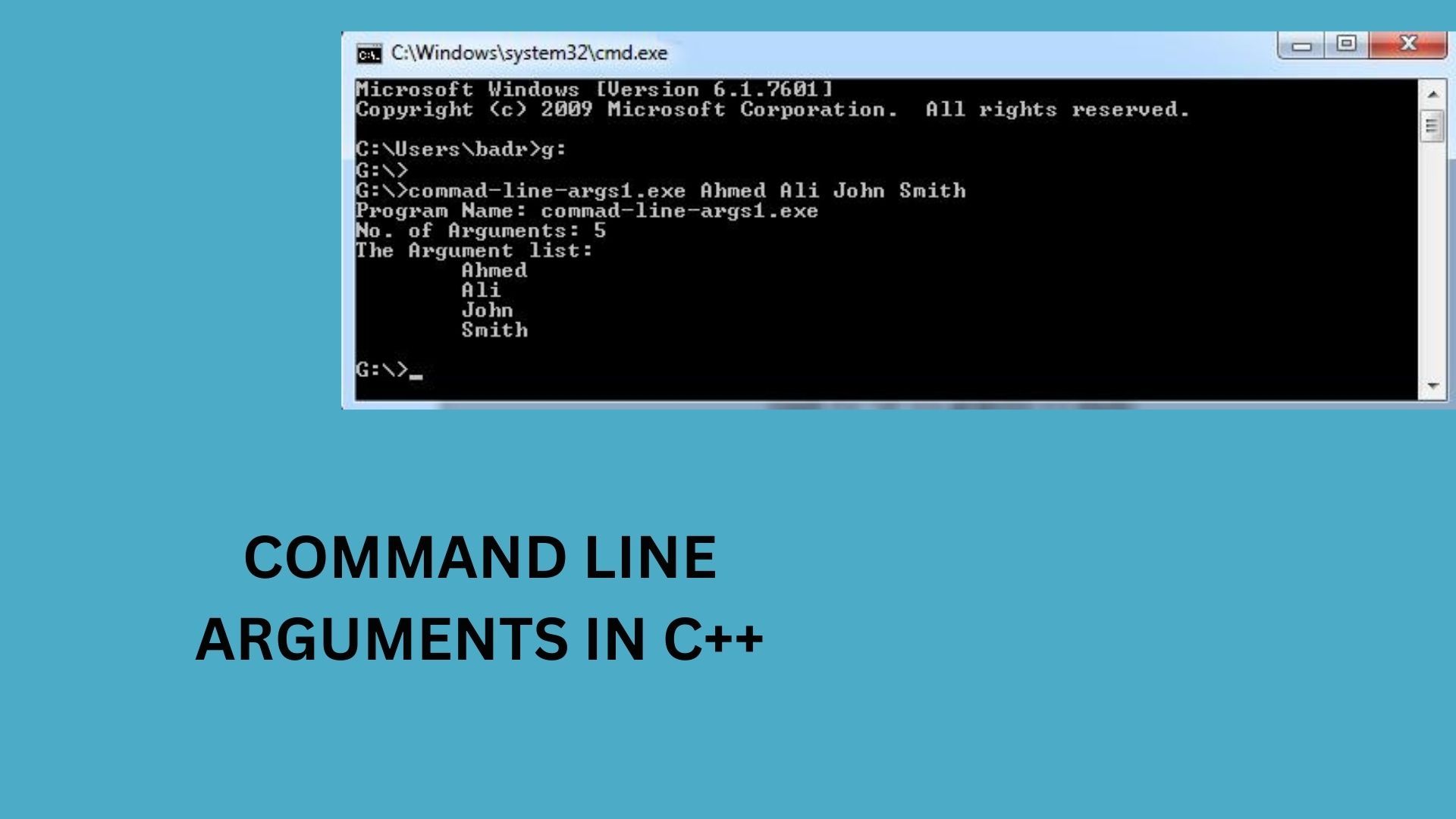
Introduction
Accepting arguments from the command line is feasible in C++. In OS such as DOS or Linux, which are Command Line Interface, program arguments are provided after the code file name and are sent to the application from the operating system. To utilize command line arguments in your code, you must first comprehend the main function's complete declaration, which up until this point has not taken any arguments. In actuality, there are two parameters that main can take: a list of all the command line arguments and the number of command line arguments.
Argc and Argv
Program's main() method will accept two parameters from the command line, namely;
- argc : The entire number of arguments given into the main function is stored in the integer type variable argc. The program name is only one of the many arguments it accepts on the command line.
- argv[]:The actual parameters provided to the main function are included in the char* type variable argv[].
The whole statement of main is as follows:
Argc, an integer, stands for ARGument Count (hence argc). It is the total amount of parameters, including the program name, that were supplied to the program from the command line. The list of all the parameters is contained in the array of character pointers. The program name is included in argv[0], or if the name is not provided, an empty string. After that, a command line argument is any element number less than argc. Each argv member can be used as a string, or argv can be used as a two-dimensional array. The pointer argv[argc] is null.
Code
How would one employ this? This is typically used by programs that want their parameters to be set when they are executed. One such application is to create a function that accepts a file's name and prints all of its text on the screen.
Conclusion
This code is not very complicated. It includes Main in its entirety. The second argument, which may possibly be a file name, is then checked to see if the user added it. The application then tries to open the file to determine whether it is legitimate. This is a routine procedure that works well and is simple. The method involves opening the file if it is legitimate. By this point in the blog, you should have no problem understanding how the code works because plenty of comments are added for the explanation part.