C++ Fundamentals: Understanding the Basics
Vector erase() in C++
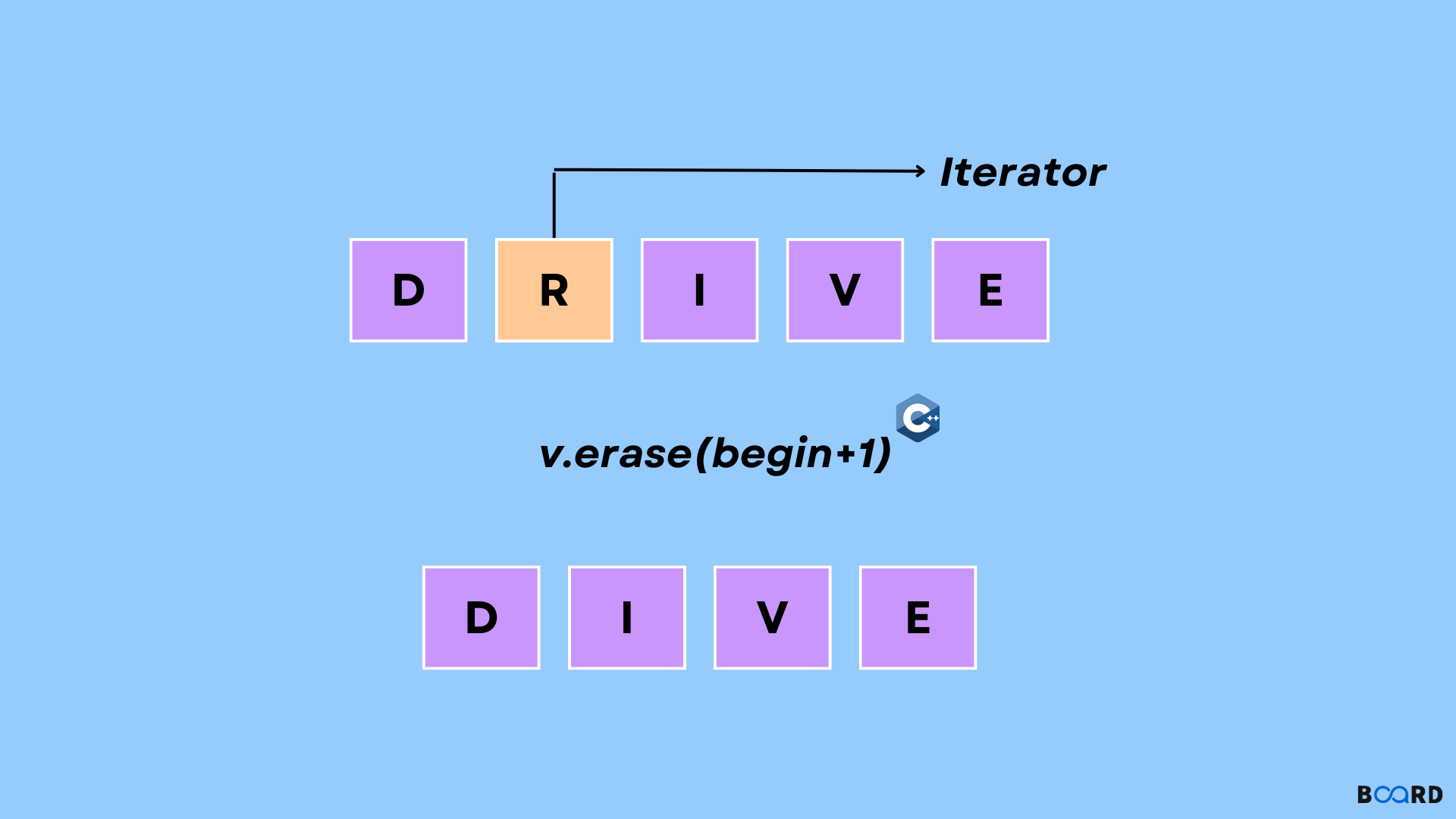
Introduction
A single element at a specific index or a group of items between two start and finish indices can be deleted using the erase() method.
Syntax
The erase() function's syntax is as follows to delete an element at a given index:
Iterator erase(const iterator start, const iterator end) is the syntax for the erase() function, which removes all the items from a particular start index to the end index. Please take note that const iterator must be specified as the position of the element to be eliminated.
Examples
Element from Vector Removed at a Specific Index
In the following C++ program, we initialize a vector name with five elements, and then remove the element at index=3.
Code
Remove the specified range of elements from the vector. [start, end)
In the C++ program that follows, we create a vector named with five entries before removing the elements starting at index=2 and continuing until index=4.
Code
Conclusion
In this C++ lesson, we learnt how to utilize the erase() function's syntax to delete a specific element from a location or a group of items that are present in a certain index range.