C++ Fundamentals: Understanding the Basics
Understanding Loops in C++
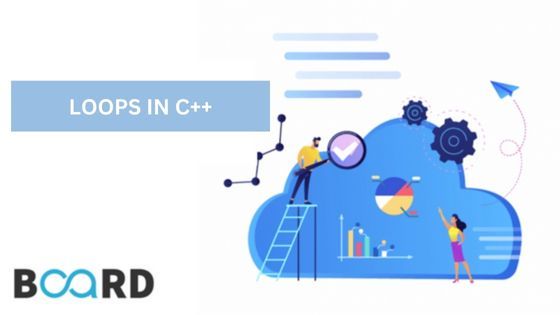
Introduction
There can be instances where you need to run a block of code repeatedly. Generally speaking, statements are carried out in order: the first statement in a function is carried out first, then the second, and so on. Different control structures offered by programming languages enable more intricate execution routes. The typical format of a loop statement in most programming languages is as follows: A loop expression allows us to run a statement or collection of statements several times.
While Loop
As long as a certain condition is true, a while loop statement continually executes a target statement.
Syntax:
Statement groups can be collective/singular. Any expression that is true for the condition is one that does not equal zero. While the condition is true, the loop iterates. The line immediately after the loop receives programme control when the condition is false.
Code
For Loop
Using a for loop, which is a repetition control structure, you can quickly create a loop that has to run a certain number of times.
Syntax
Code
Do While Loop
The do...while loop tests its condition at the bottom of the loop, in contrast to for and while loops that test the iteration condition at the top of the loop. Though a while loop is comparable to a do...while loop, the former is guaranteed to run at least once.
Syntax
Code
The output for all the 3 code snippets above will be the same as shown below
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
Nested Loops
A loop can be nested inside of another loop. C++ allows at least 256 levels of nesting.
Syntax
The following is the C++ syntax for a nested for loop statement:
The following is the C++ syntax for a nested while loop statement:
The following is the C++ syntax for a nested do...while loop statement:
Hope this gave you a clear understanding of loops in C++. To find more information regarding loops and other topics, visit our Discussion Forum.