C++ Fundamentals: Understanding the Basics
Understanding Templates in C++
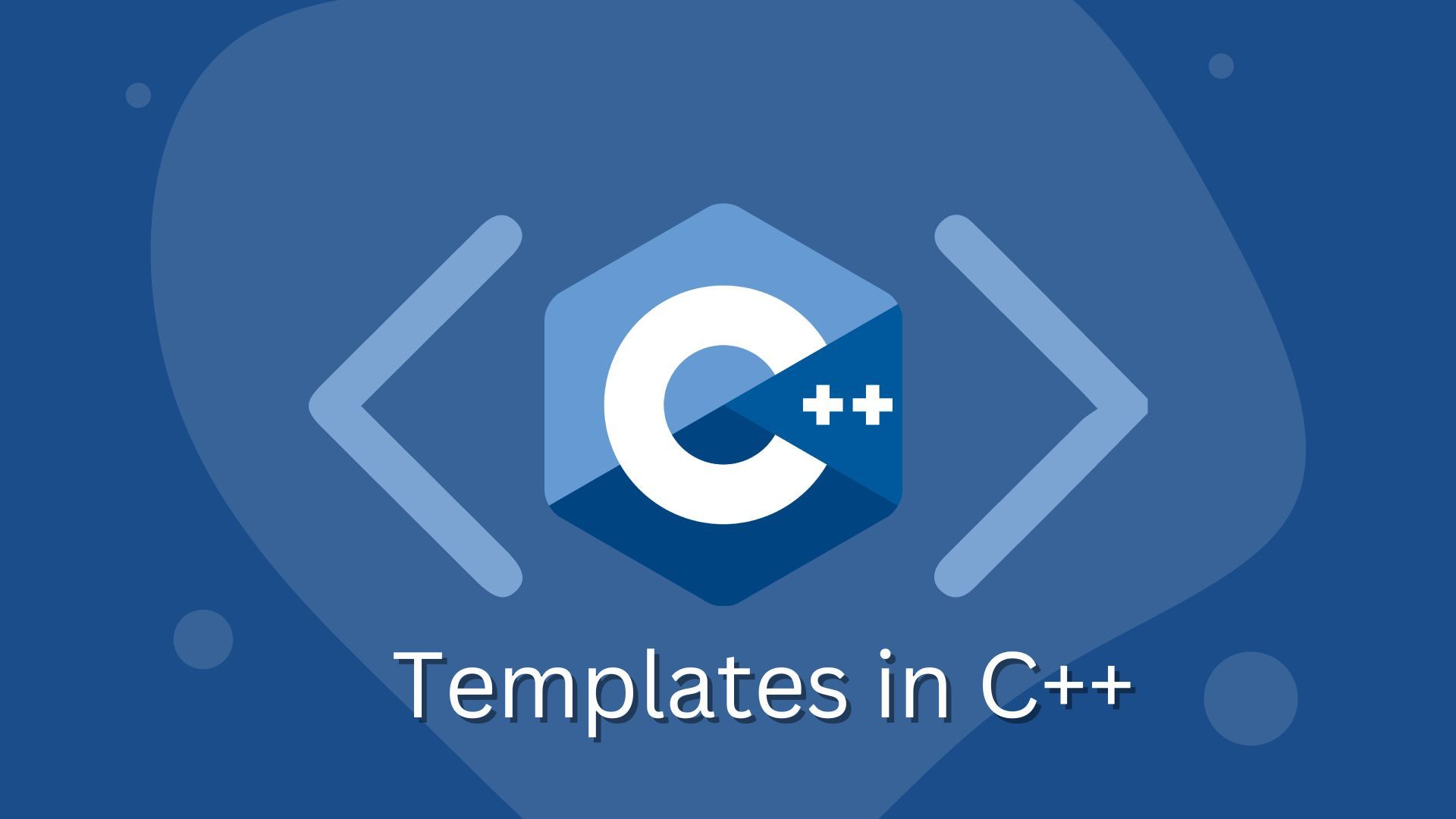
Introduction
With the aid of examples, we will talk about C++ class templates in this article. We can develop generic programmes because of the C++ language's excellent template mechanism. We may use templates in two different ways:
- Class Templates
- Function Templates
Class templates may be used to construct a single class that works with several data types, just like function templates can. Class templates are useful since they can reduce the length and complexity of our code.
Template Declaration
The class declaration comes after the template keyword is followed by any template parameters contained inside a > tag.
In the aforementioned declaration, class is a keyword, and T is the template parameter, a placeholder for the utilized data type. A member variable named var and a member function named functionName() both have the type T inside the class body.
Instantiating Templates
The following syntax allows us to build an object from a class template in the other classes and functions (like the main() method) after we've declared and defined the class template.
Code
Explanation
With the code, we have generated a class template called Number in this application. Note that the function Object() { [native code] } argument n, the variable num, and the method getNum() are all of type T or have a return type of T. They can thus be of any sort, according to that. Each class definition for int and float is created by Numberint> and Numberdouble>, which are then utilized as appropriate. Whenever class template objects are declared, the type must be specified. Otherwise, a compiler error will be generated. We implemented the class template in main() by constructing its instances.
Analysis of Templates
The compiler does not view templates as regular functions or classes. They are "on demand," which means that they wait to compile the logic of a template function until a specific instantiation with a particular set of template arguments is necessary. When an instantiate is necessary at that point, the compiler creates a function from the template, particularly for those parameters. Since no code is created until a template is constructed when necessary, compilers are ready to accept the inclusion of the same template file many times in a project without causing linkage issues.