C++ Fundamentals: Understanding the Basics
Understanding 2D Vectors in C++
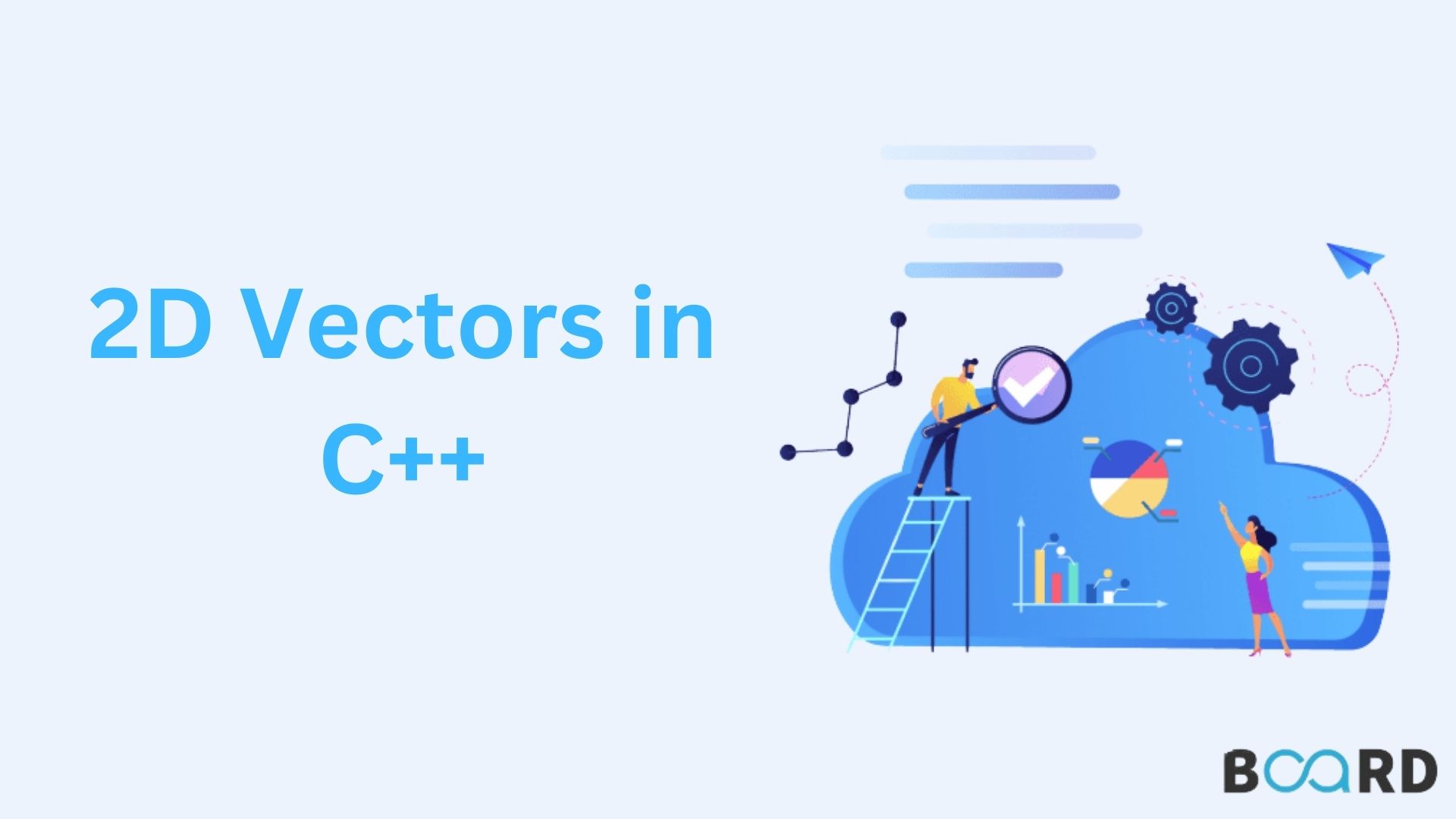
Introduction
Dynamic arrays, or vectors in C++, can dynamically enlarge themselves when an item is added or withdrawn, with the container controlling its storage on an automated basis. A two-dimensional vector, sometimes referred to as a vector of vectors, is a vector with a variable number of rows, each of which is a vector. Each vector index in this case contains a vector that iterators may both traverse and access. In other words, the only difference between a vector of a vector and a vector array is in the dynamic features. We shall go into great detail about C++ 2D Vector in this blog.
Syntax
You must comprehend the fundamental grammar of a programming language while learning a new notion in that language. So let's examine the two-dimensional vector's syntax.
How does a 2D Vector work in C++?
We now understand how a two-dimensional vector is written. Time to see an example of the same, then.
Three rows and four columns are used to initialize the vector vtr in this instance. This will be presented as a vector if we print it using a for loop. We can tell a vector looks like a matrix just by looking at it. However, this is more flexible than the matrix since elements may be added or removed based on our needs. Among the uses for a 2-D vector are:
- Representation and modification of images
- Applications of the 2-D grid
- For representation in dynamic programming
Examples
Here are a few 2-D vector example applications.
Example #1 - Initialization of a two-dimensional vector using CPP.
Code:
A header file for handling the vector is first described in this application. When initializing the vector, the members of the vector are also specified. The vector is then printed using a loop.
Example #2 - Using a one-dimensional vector pushed to the back, the CPP code initializes a two-dimensional vector.
Code
In this code, too, a header file for supporting the vector is specified initially. Additionally, constants R and C are provided to specify the number of rows and columns, respectively. The vector's items are then returned to the first vector by utilizing the pushback () function. Variable el is used to refer to the vector's initial value. A vector is printed once the code has been executed.
Conclusion
A vector with two dimensions has a customizable number of rows, and each row contains a vector. The 2-D vector's many characteristics are thoroughly covered in this article.
Hope this article gave you a clear understanding of the concept. For similar topics visit Board Infinity Blogs and Discussion Forum.