C++ Fundamentals: Understanding the Basics
Understanding Vectors in C++
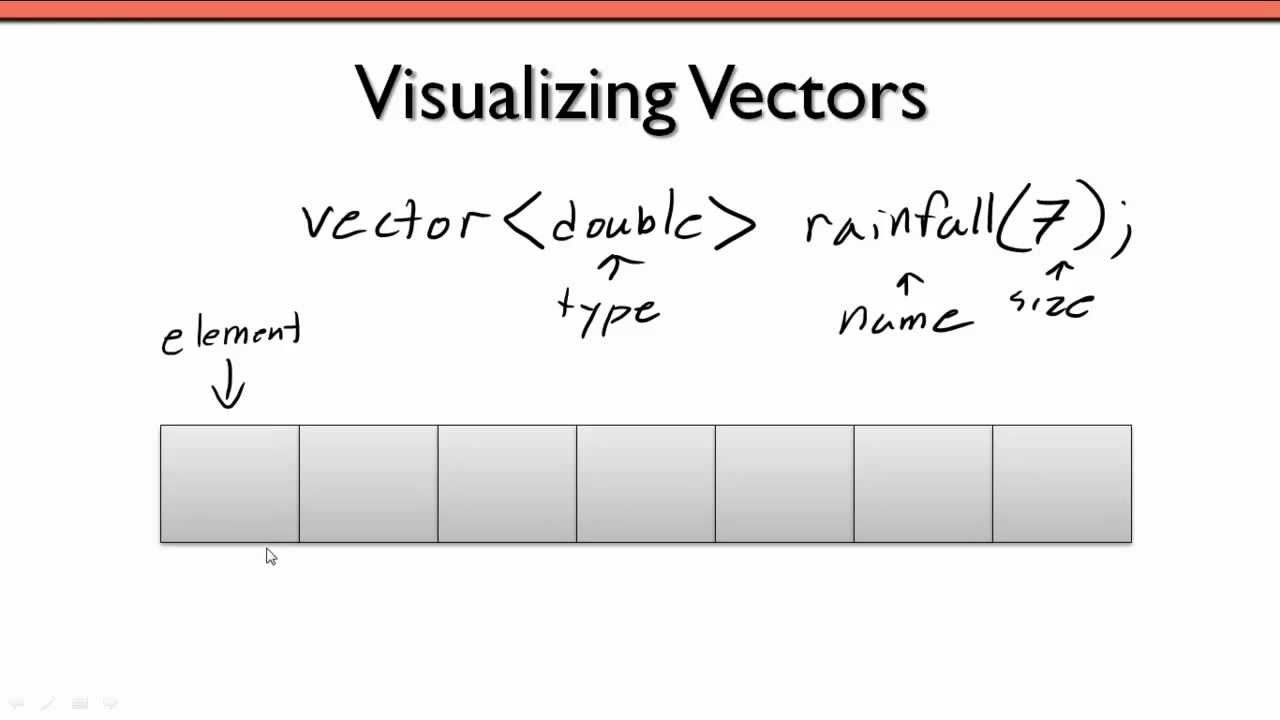
Introduction
The STL, a collection of general-purpose classes and methods used mostly for storing and processing data, includes vectors. In C++, vectors are run-time sequence containers that represent arrays with variable size. Their elements may also be accessed utilizing offsets on conventional pointers to their elements since they employ contiguous storage spaces for their elements just as effectively as in arrays. Data is stored in dynamic arrays called vectors. Vectors provide the programme greater freedom than arrays, which are static in nature and only hold sequential data. When an element is added to or removed from a vector, the vector's size can be adjusted automatically.
Properties of Vectors
- In C++, vectors are not ordered.
- Iterators make it simple to retrieve and navigate through vector items that are stored in nearby storage.
- Data is added at the end of vectors when the push back() method is used.
- As there may occasionally be a need to expand the vector, adding an element to the end of the vector requires differential time, but adding an element to the beginning or center of the vector just requires linear time.
- Because there is no scaling involved, removing the final element just requires constant time.
Before utilizing vectors in C++, the #include <vector> header directive must be written.
Syntax for Vector declaration
vector< object_type > vector_variable_name;
Initialization
- Pushing each item in a vector one at a time with push_back()
- The push back() function is used to sequentially push back each element that has to be put in the vector.
- Syntax:
vector_name.push_back(element_value);
Overloading the constructor:
- This technique is used to insert the same value into a vector many times.
- Syntax:
vector<object_type> vector_name (number_of_repetition,element_value);
Passing an Array:
- When using this approach, the vector function Object() { [native code] } is handed an array as an argument.
- Syntax:
vector<object_type> vector_name {val1,val2,val3,....,valn};
Using ready-made vector:
- This technique produces a new vector that has the same values as an existing vector.
- The begin() and end() of an initialised vector are sent by this method.
Syntax:
vector<object_type> vector_name_1{val1,val2,…,valn};
vector<object_type> vector_name_2(vector_name_1.begin(),vector_name_1.end())
Functions associated with Vectors
Modifiers
- assign() – gives the vector's current elements a new value.
- push_back() – the element from the vector's rear is pushed.
- pop_back() – removes components from the vector's back.
- insert() – adds a new element to the vector before the one that is supplied.
- erase() – is used to delete elements from the vector's given element or range.
- swap() – swaps the information in two vector of the same datatype. The dimensions of vectors may vary.
- clear() – The vector's whole contents are removed using it.
Iterators
- begin() – returns an iterator that points to the vector's first element.
- end() –returns an iterator that points to the vector's final element.
- rbegin() – returns a reverse iterator that points to the vector's final element.
- rend() – returns a reverse iterator pointing to the vector's element before the first. essentially seen as the opposite end.
- cbegin() – returns a consistent iterator referring to the vector's top-most component.
- cend() – returns a fixed iterator pointing to the vector's subsequent element after the last element.
- crbegin() – returns a const reverse iterator pointing at the vector's final component.
- crend() – returns a const reverse iterator pointing to the vector's first-preceding-element element.
Capacity
- size() – returns the number of items the vector presently contains.
- max_size() – returns the maximum number of components a vector can accommodate.
- capacity() – returns the presently allotted storage space.
- resize(n) – resizes the storage space for n components.
- empty() – checks if container is empty
Element access
- reference_operator[g]:returns a reference to the vector's "g" element.
- at(g): Returns a reference of the element in the vector at point 'g'.
- front(): Returns a pointer to the vector's topmost element.
- back(): Returns a pointer to the vector's last element.
- data(): Returns direct reference to the memory array that the vector internally uses to hold the elements it owns.
Code
Output
Advantages of C++ Vectors
- Its nature is dynamic.
- It is simple to insert and remove elements.
- It is possible to store several items.
- Vectors may be easily copied from one to another by utilizing the assignment operator.
Disadvantages of C++ Vectors
- Memory use has increased.
- No index exists for it.
- Contiguous RAM is not utilized.
As we have learned more about C++ vectors, it is evident that this type of data structure not only serves as a dynamic array but also makes sure that elements related to that vector may be accessed quickly and randomly. Now, you can efficiently manage computer memory when adding, deleting, traversing, and changing items in vectors. Now we know where in a program to use vectors and where to use arrays. Every C++ expert has to understand vectors. This brings us to the conclusion of our discussion of vectors in C++.