C++ Fundamentals: Understanding the Basics
rand() Function in C++
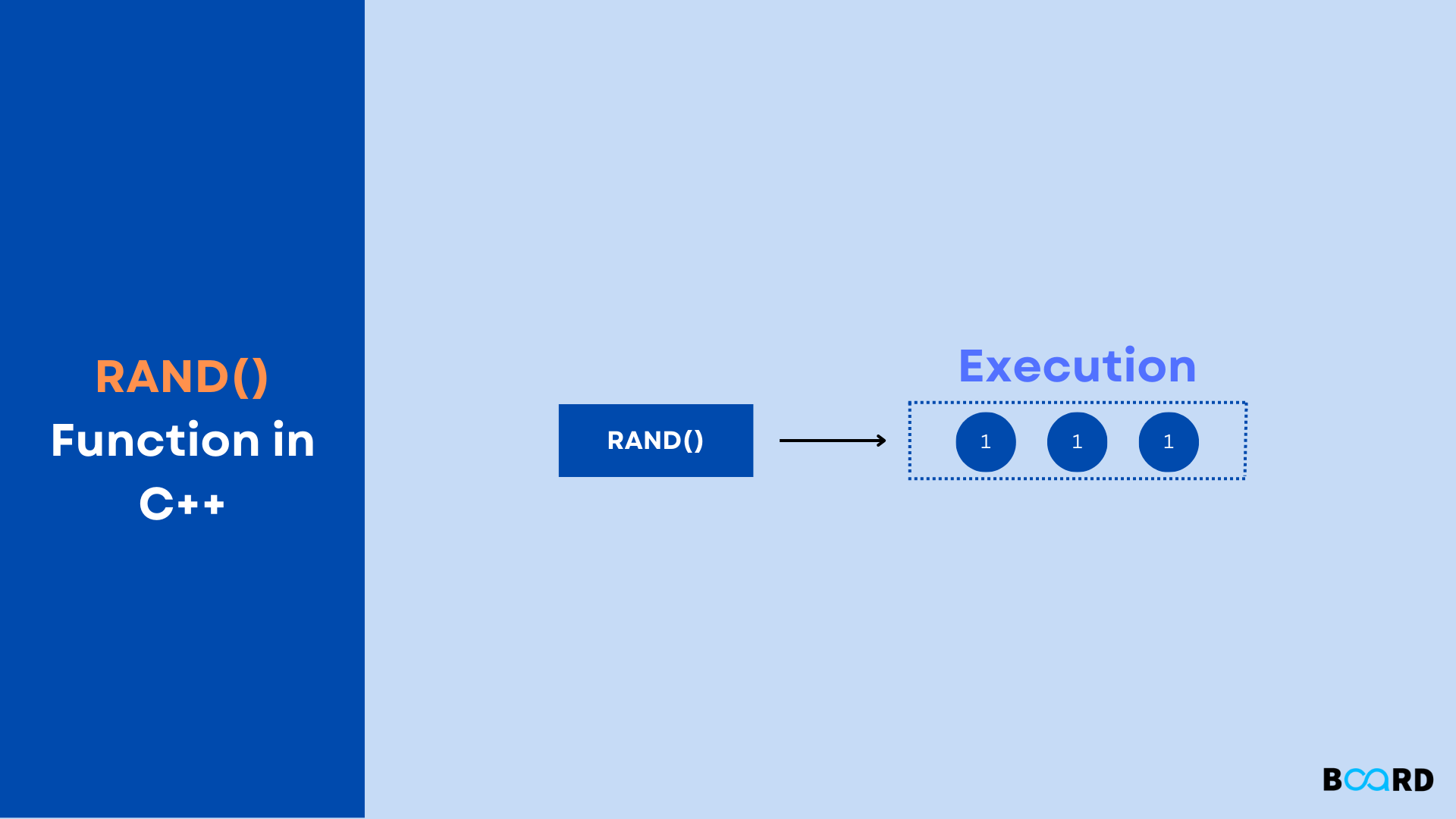
Introduction
This tutorial explains in detail how to use the rand() function in C++ to generate random numbers. In order to create simulations, games, and other applications that call for random events, we frequently need to use random numbers in our code. For instance, if there are no random events in a dice game, we will always get the same side when we roll the dice, which will produce unfavorable outcomes.
As a result, it becomes essential that we have access to a random number generator. We can generate random events in the physical world, but this is not possible with computers. In this tutorial, we will go into great detail about how to produce random numbers in C++.
Understanding Random Number Generation
A pseudo-random number generator is a computer programme that uses mathematical operations to transform a seed or starting number into a different number (PRNG). The most recent generated number is used each time as we repeat this process. Every time, the generated number is different from the previous numbers. This programme can therefore generate a series of numbers that appear random. A built-in pseudo-random number generator is available in the C++ language's rand () function, which can be used to generate random numbers.
rand ()
Function prototype: int rand (void);
Parameters: none
Return value: INT val ranging from RAND_MAX & 0
Description: The subsequent random number in the series is produced by the rand () function. The result is an integer that is pseudo-random and falls between 0 and RAND MAX. The default setting for the constant RAND MAX in the cstdlib header is 32767.
By using the system clock as the seed for the srand function in the aforementioned software, we were able to produce the first 100 random numbers. We have utilized both the srand and rand functions in our software. Be aware that each time the programme is run, a different output will be produced because the system clock serves as a seed.
C++ Generating Random number between 0 and 1
To create random numbers ranging from 0 and 1, we can use the srand () and rand () functions. Keep in mind that the output of the rand () function must be converted to a decimal value using either a float or double. To display fractional random integers between 0 and 1, the rand () function's integer default return value is insufficient.
The following C++ software displays the first five randomly generated numbers between 0 and 1.
Output:
As can be seen, the software generates fractional random numbers between 0 and 1. We will receive 0 as the random number if we don't cast the return result of the rand () function to a float or double.
In this tutorial, we went over Random Number Generation in great detail. Computers, or programming languages in particular, do not produce random numbers because their output is intended to be predicative. As a result, we must imitate unpredictability. We utilize the built-in C++ pseudo-random number generator (PRNG) to imitate randomness. Thus, we may produce random numbers in C++ using the rand() function in C++