C++ Fundamentals: Understanding the Basics
Learn about Strings in C++
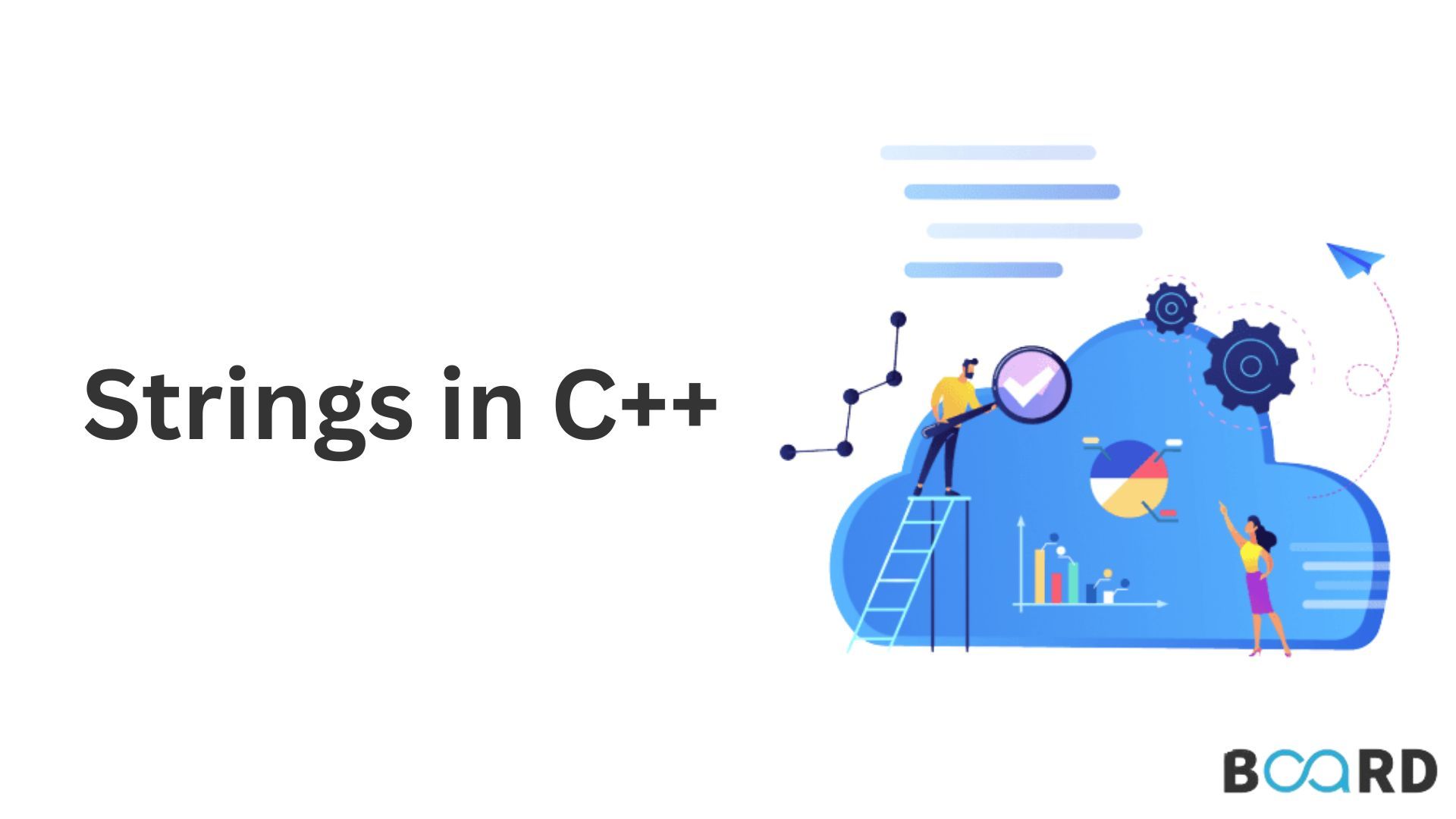
Introduction
An orderly series of characters is referred to as a string. In C++, a class object can be used to represent a string of characters. A String class is the type of class that offers such a definition. The single-byte character may be accessed thanks to the capabilities provided by the String class, which saves characters as a series of bytes. Double quotes are used as enclosing delimiters in C++.
String Class and Character Array Difference
Declare and Initialize Strings in C++
In C++, initializing a string is rather easy.
using namespace std;
string std_string; Or std::string std_string;
Both the initialization methods for the string class (st and std st) and the character array (ch) have been demonstrated in this example. As a starting point, we applied the character array technique by creating the character array ch[12], which has 12 entries and ends with a nil character. We applied a string class method in the second section.
Operations on Strings in C++
There are numerous built-in methods in C++ to modify strings, which is a benefit of utilizing the string class. Programming is now simple and efficient. Let's examine a few crucial string manipulation methods and learn about them through various examples.
String Size: The size of the object may be determined using the length() and size() methods.
String Concatenation: Concatenating strings is as simple as applying the + operator between two or more strings.
Appending Strings: A string can be concatenated and added to at a precise character place by using the class member function.append(string). If a programmer uses the syntax str.append(str1, p, n), then the string str will have n characters from position p added to the end.
Searching strings: The find() member function can be used to locate the first instance of a string inside another. find() will start searching for a string needle inside a string haystack at position pos and return the location of the needle's first appearance. Similar to find(), rfind() only returns the final instance of the provided string.
The index of the first instance of the letter "o" in the haystack string is "4", which will be printed by the first cout command. If we need the "o" in "World," we must change "pos" such that it points beyond the very first instance. The results of haystack.find(needle, 4) and haystack.find(needle, 5) were both 4 and 7, respectively. Find() returns std::string::npos if the substring cannot be located. Npos is a unique value that is equal to the largest value the type size type may represent. It is 4294967295 here. It is often used by functions that anticipate a string index as the end of the string indicator or by functions which return a string index as the error indicator.
This little code snippet finds every instance of "C++" in str2 and reports their locations:
Conclusion
This marks the conclusion of our discussion of strings in C++.