C Programming: From Basic Syntax to Advanced Concepts
All about C Programming Language
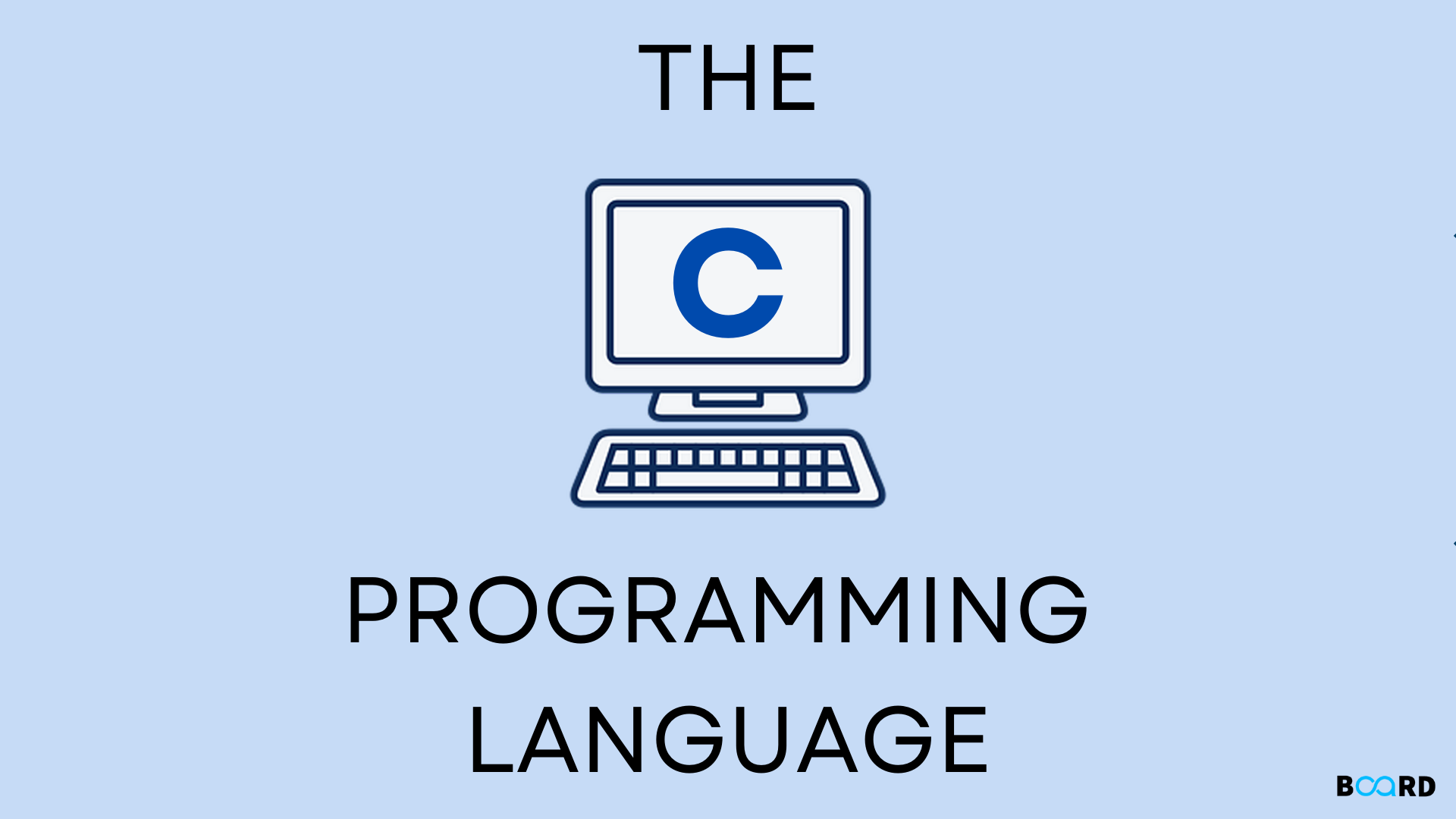
Introduction
C is one of the most commonly used programming language.C is a procedural programming language.Dennis Ritchi developed this language to write program for operating system.C is also used in compiler development.
Now you must be thinking that why you should learn C Programming. Here are the reasons:
- C helps you to understand the internal architecture of a computer, how computer stores and retrieves information.
- After learning C, it will be much easier to learn other programming languages like Java, Python, etc.
- Opportunity to work on open source projects. Some of the largest open-source projects such as Linux kernel, Python interpreter, SQLite database, etc. are written in C programming.
Keywords in C
Keywords are predefined, reserved words used in programming that have special meanings to the compiler. Keywords are part of the syntax and they cannot be used as an identifier.
A list of 32 keywords in the c language is given below:
Identifiers in C
Identifier refers to name given to entities such as variables, functions, structures etc.Identifiers must be unique. They are created to give a unique name to an entity to identify it during the execution of the program.
int n = 10;
Here n is the identifier.
Let’s see some programs in C to understand this language better:
C Program to find factorial of a number
Output:
Let’s see a sorting algorithm in C Language:
Insertion sort program in C
So this is how we write programs in C Language.Do visit our other blogs on Board Infinity to know more about C Language.Do share this blog and keep Learning.