C Programming: From Basic Syntax to Advanced Concepts
Decision Making in C
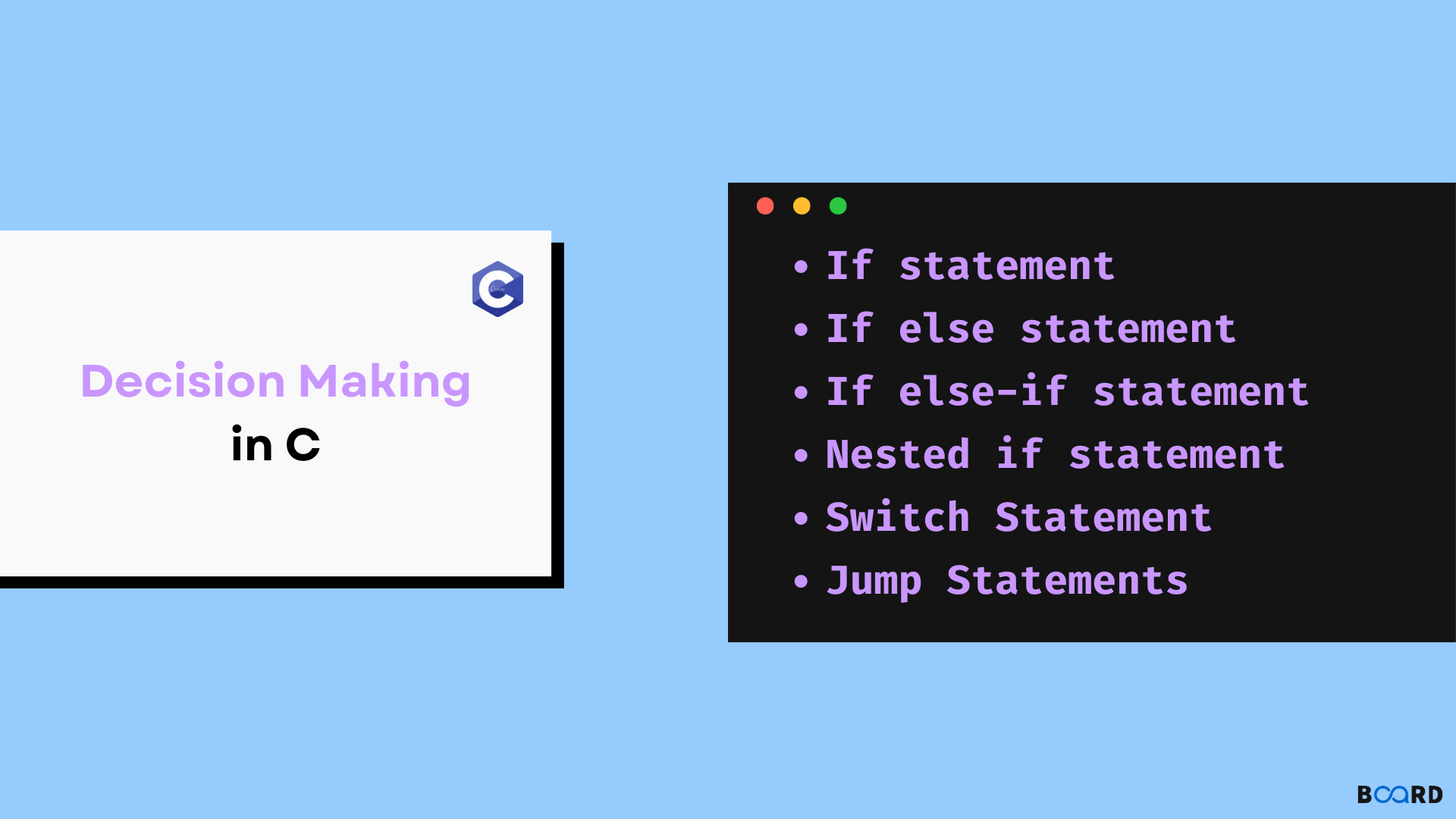
What is a Decision-Making statement?
Decision-making statements determine the program's arc and flow. Because they provide conditions using boolean expressions that are evaluated to a true or false boolean value, they are sometimes referred to as conditional statements. A particular piece of code will run if the condition is true; if the state is false, the block will not run.
The statements below in C can be used to make decisions.
- If Statement
- If..else statement
- if..else-if statement
- Nested if statement
- Switch statement
- Jump Statement
If Statement
The if statement is the most basic and direct expression for making a choice. It is used to decide whether or not to run a specific code section. A block of statements is only performed if a particular condition is met; otherwise, it is not.
Syntax:
The if statement is the most basic and direct expression for making a choice. It is used to decide whether or not to run a specific code section. A block of statements is only performed if a particular condition is met; otherwise, it is not.
Example:
If the condition is true, the previous snippet will be considered inside if and executed.
Example:
Output:
The statements included inside the if statement is carried out if the condition present in the if statement evaluates to true.
If else
A block of statements will be performed if a condition is true, and they won't be executed if the condition is false, according to the if statement.
What happens, though, if the condition is false and we wish to take a different action? The if-else phrase is used in this situation. We may use the otherwise statement in combination with the if statement to execute a code block when the condition is false.
Syntax:
Example: Program to check if a given number is even or odd
Output:
If-else-If ladder
In C, if-else-if is used to make decisions when several possibilities are available. The "if" statements are carried out bottom-up. The statement related to that if is performed when one of the conditions governing it is met, and the rest of the ladder is skipped. The last else statement is carried out if none of the requirements are met.
Syntax:
Example: Check whether an integer is positive, negative, or zero.
If we input -11, the first condition is verified since the value is less than zero. The statement within the else-if is now executed since the following else-if is examined, and the number is found to be less than 0.
Nested if
Suppose statements that are nesting are included within another if statement. We may use an if statement within another if statement in C and when we need to make several judgments, and nested if statements in C are helpful.
Syntax:
Example: In this example, we would first determine whether a number was more than 10 before deciding whether it was greater than 20.
Nested if else
In C, nested if else may also be utilized to make decisions. In C, nested if else can be used when a sequence of decisions is necessary.
Syntax:
For Example: in this program, we will determine whether a number is divisible by 10 and whether it is equal to 10 or not.
Output:
Switch Case Statement
As a condensed version of the Nested If-Else statement, often used in C to make decisions, a switch case statement helps programmers avoid creating lengthy chains of if-else-if. A switch-case statement determines the block of code that should be performed by comparing an expression to several circumstances.
A single evaluation of the expression, which must result in a "constant" value, is performed before it is compared to the values of each case label (constant 1, constant 2, .., constant n).
If a match is discovered for a case label, the code after that label is executed until a break statement is reached or the control flow reaches the switch block's conclusion.
In the absence of a match, the code following default is run.
Example: Program to identify numbers between 1-5
Jump Statements
Jump statements cause unconditional jumps to other statements elsewhere in the code. They are mainly employed to break loops and switch statements.
In C, there are four different forms of jump statements.
- Break
- Continue
- Goto
- Return
The part that follows will go through these leap statements for C decision-making.
Break Statement
When the break statement is used in C, the loop or switch statement is terminated, and control is instantly transferred to the statement before the loop.
Syntax:
Break statements are typically used when we don't know how many times a loop will run and wish to end the loop depending on specific criteria.
Example: Identify any negative values in an array.
Output:
The C decision-making statement "continue" is the exact reverse of the "break" statement in that it compels the loop to run through its remaining iterations rather than ending it.
The code that follows the continue statement is skipped when the continue statement is run, and controls advance to the next iteration.
Syntax:
Example: Print out all array values that are not negative.
Output:
GOTO Statement
By shifting control to another area of the programme, the goto statement may change the typical flow of programme execution. Within a function, you may hop from one place to another by using the goto statement.
Syntax:
Example: Using the goto statement, determine if a number is even or odd and report the result appropriately.
Output:
Return Statement
The return statement ends the execution of a function and returns control to the caller function. It can also indicate the value the function should return. There might be one or more return statements in a function.
Syntax:
Output: