C Programming: From Basic Syntax to Advanced Concepts
Storage Classes in C
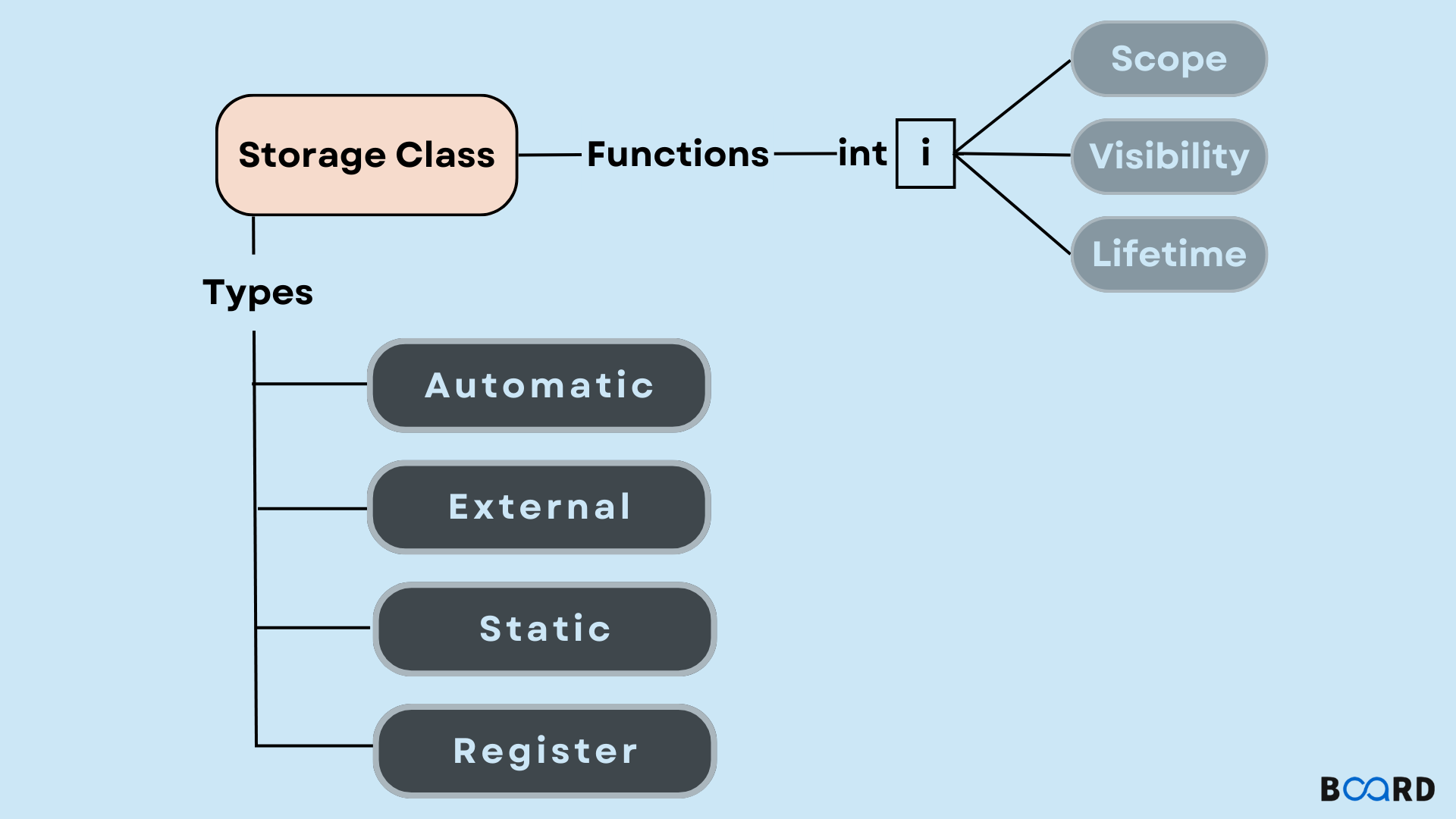
What are Storage Classes in C?
Computer Programming is all about storing and processing information to generate some results. Therefore, the concept of Variables plays a critical role. Variables allow us to store information in some memory location and manipulate them using some reference.
So, it’s important to describe some features of those variables like their scope, default values, lifetime, etc. This is done using Storage Classes, which determine the visibility, lifetime, default values, memory location, and scope of the variables within a C Program. Thus, Storage Classes in C help us to trace the existence of the variables during Program execution.
In this article, we will discuss this. But before diving into storage classes in C, it is important to know the difference between the scope and lifetime of a variable in C. The scope of a variable is the region or space in code up to which the variable is available to use. Whereas, the lifetime of a variable is the time for which the variable occupies a valid space in the system memory.
Types of Storage Class
Now, let’s see the storage classes in C with examples. C Language provides us with four types of Storage Classes which are named below and summarized in the diagram:
- Automatic (auto)
- External (extern)
- Static
- Register
The auto Storage Class
This is the default storage class for all the variables in a C Program. This means if variables are not associated with any particular storage class, they are assigned an ‘auto’ storage class. Variables of auto storage class have a default value as garbage and their scope is local, which means they can be accessed only in the function in which they have been declared.
Their lifetime is within the function which means memory assigned to auto variables becomes free after the code is executed. Let’s understand this using a simple C Program.The following code shows the default value of auto variables.
Also, the following code snippet shows the scope of auto variables.
External Storage Class
Out of all Storage Classes in C, only this storage class allows variables to be declared with the global scope. It can be said that an External Variable is a global variable declared within the function. The default values of variables of the extern class are 0 or null and their lifetime is till the end of the main function. An important point is that the declaration and initialization of external variables cannot be done at the same time. The following programs show the implementation of the external storage class.
Now, in the following code, we have accessed the external variable i locally and globally.
Output:
Similarly, we will see the working of other Storage Classes in C.
Static Storage Class
Static Storage Class is used to declare the static variables in C, which are frequently used in Programs. Static Variables are those variables that can hold values between multiple function calls.
Variables of this storage class are initialized only once and exist till the termination of the program. They are visible only to the block in which these are defined and initialized with 0 as the default value. The working of the Static storage class can be understood using the below code:
The variable i is non-static while j is static. Thus, j is incremented to 1 in the first call and its value is preserved for the next call. Hence, this is the most frequently used class among the various storage classes in C as it can be used to preserve the values of variables till the main program terminates.
Output:
Register Storage Class
If you are familiar with the concept of Register, it will be easier for you to understand this storage class. The variables of this class are similar to that of the auto class. The difference is that the compiler tries to store these variables in the register of the microprocessor. Since the accessing of a register is faster than the variables stored in the memory, register variables work faster.
The main use of the Register is to access frequently used data. So, if some variables have to be accessed many times, then they can be declared as register variables. The initial default value of register variables is garbage, their scope is within the block and their lifetime is till the end of the block. The following code demonstrates the working of register variables:
An important point to note is we cannot obtain the address of the register variables using a pointer. Also, if register memory is not sufficient, these variables are stored in the system memory. This was the whole concept of Storage Classes in C which helps to use the variables in a better way.