C Programming: From Basic Syntax to Advanced Concepts
Nested Structures in C
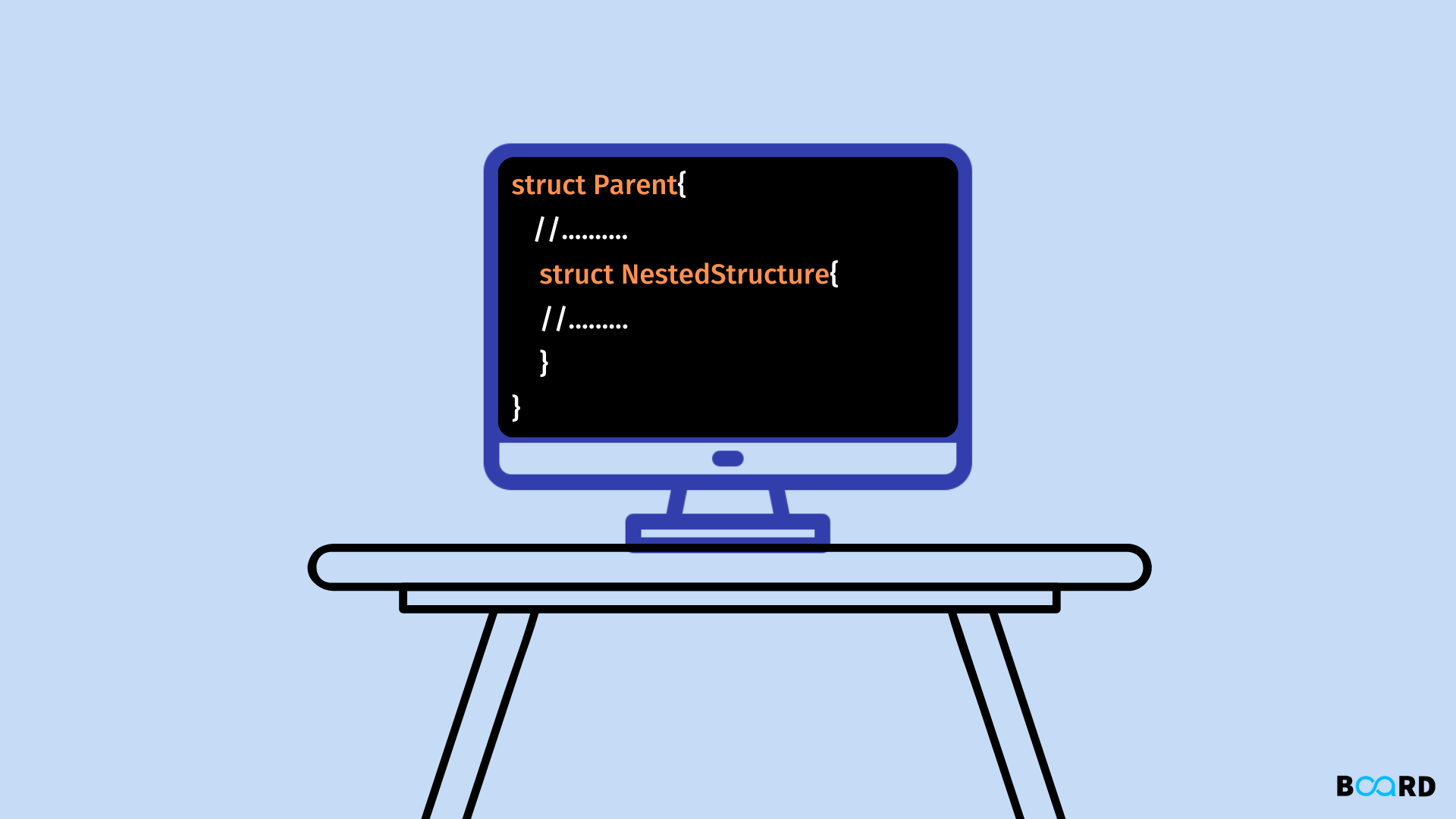
Introduction
A nested structure in C is a structure that contains one or more members that are themselves structures. Nested structures are useful for organizing complex data and can help improve a program's readability and maintainability.
To define a nested structure in C, we use the following syntax:
In this example, outer_structure is the name of the outer structure, and inner_structure is the name of the inner structure. inner is a member of the outer structure, which is a structure of type inner_structure.
To access the members of a nested structure, we use the dot operator (.). For example, to access inner_member1 in the nested structure above, we would use the following syntax:
We can also define a pointer to a nested structure using the following syntax:
In this example, ptr is a pointer to a structure of type outer_structure, and we use the arrow operator (->) to access the members of the nested structure.
Nested structures can be nested to any depth and can be used in conjunction with arrays and other data types to create complex data structures.
One common use for nested structures is to represent records in a database. For example, consider a database that stores information about employees. We might define a structure to represent an employee as follows:
In this example, the employee structure contains an id member, a name member, and an addr member that is itself a structure of type address. The address structure contains four members: street, city, state, and zip.
To create an array of employees, we can use the following syntax:
To access the members of a particular employee in the array, we can use the following syntax:
In this example, i is the index of the employee in the array, and we use the dot operator to access the members of the nested structure.
Nested structures can also be used with other data types, such as pointers and arrays, to create more complex data structures. For example, we could define a linked list of employees using the following syntax:
In this example, we have added a `next` member to the `employee` structure, which is a pointer to another `employee` structure. This allows us to create a linked list of employees, where each employee is linked to the next employee in the list.
To create a linked list of employees, we can use the following code:
In this code, we create two employee structures and link them together to form a linked list. The `head` variable points to the first employee in the list, and the `next` member of each employee points to the next employee in the list.
Conclusion
In conclusion, nested structures in C are a useful tool for organizing complex data and can help improve a program's readability and maintainability. They can be nested to any depth and used with other data types to create complex data structures, such as linked lists and databases.