C Programming: From Basic Syntax to Advanced Concepts
Type Conversion in C
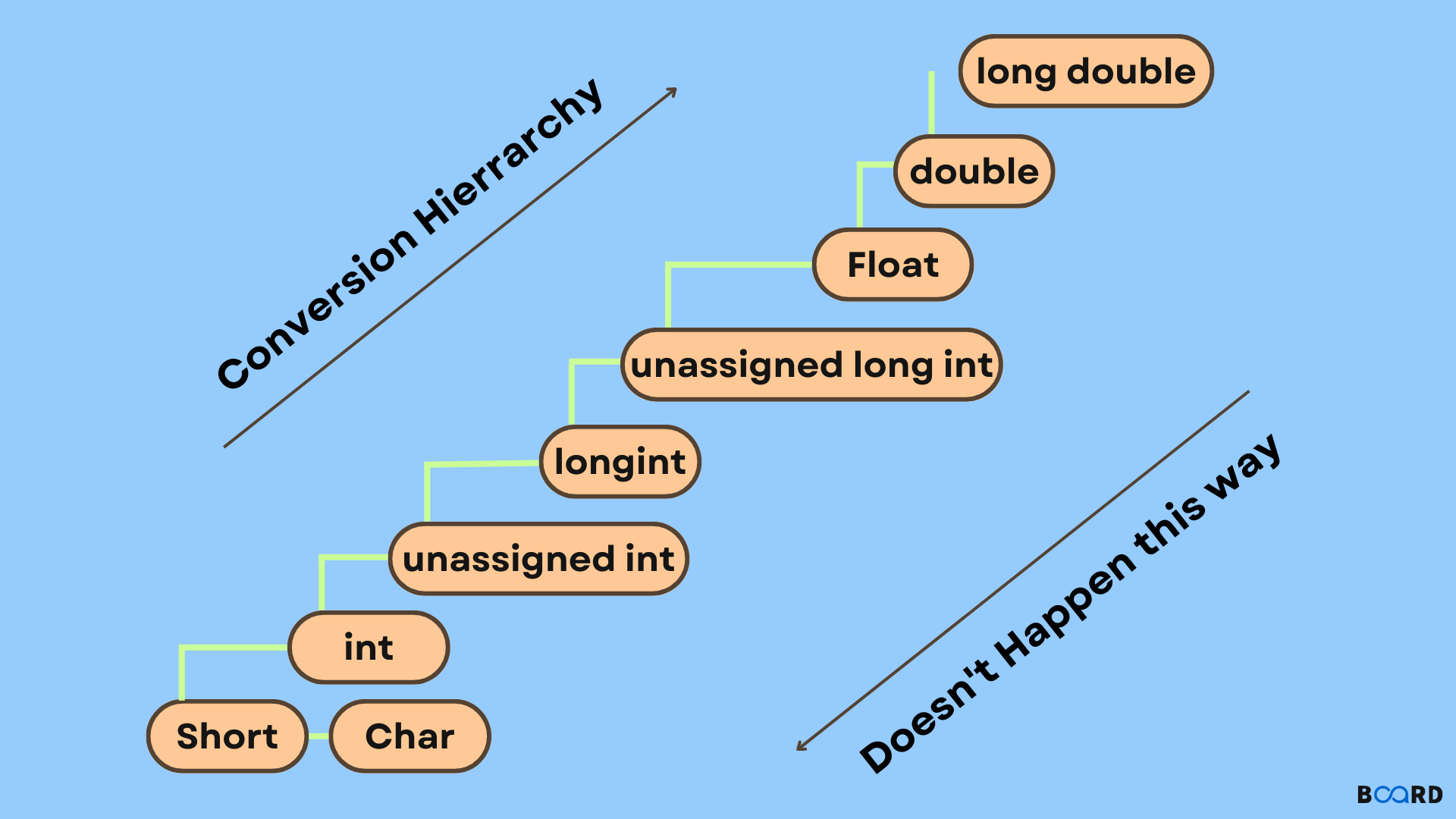
Introduction
The expression in a computer language contains data values of the same or various data kinds. When the expression contains similar datatype values, it is evaluated correctly. However, if the phrase contains two or more different datatype values, they must be transformed to the destination datatype's single datatype. The destination in this case is the location where the final result of that expression is saved. For instance, multiplying an integer data value by a float data value and storing the result in a float variable. In this scenario, the integer value must be transformed to a float value, resulting in a float datatype value.
The integer variable number is given the double value 34.78 in this case. That a double value is converted back to integer value 34 in this case.
Types of Conversion
Implicit type conversion is the name given to this type of conversion. There are 2 kinds of type conversion in C:
- Implicit type Conversion
- Explicit Type conversion
Implicit Type Conversion
As previously stated, implicit type conversion automatically converts the value of one type to the value of some other type. As an example,
Output
A double variable with the value 4150.12 is used in the preceding example. It's worth noting that we've allotted the double value to an integer variable.
The C compiler automatically changes the double value 4150.12 to the integer value 4150 in this case.
Because the conversion occurs automatically, this type of converting is known as implicit type conversion.
Example:
Output
The preceding code generated a character variable alphabet with value 'a.' It's worth noting that we're attributing alphabet to an integer variable.
The C compiler automatically changes the character 'a' to integer 97 in this case. This is because characters in C programming are internally saved as integer values known as ASCII Values.
ASCII is a set of characters used in computers to encode text. Because the character 'a' has the integer value 97 in ASCII code, it is easily transformed to integer 97.
Explicit type Conversion In C
We manually convert value systems of one type of data to another in explicit type conversion.
Example
Output
In the preceding programme, we declared an integer variable named number with the value 35. Take note of the code.
(double) - the data type to which the number is to be converted number
number - the value to be converted to double type
Example:
Output
In the code above, we created a variable with the value 97. It's worth noting that we're converting this integer to a character.
(char) - explicitly converts a number to a character value number
number - the value to be converted to char type
Data loss In Type Conversion
When we transformed a double type value to an integer type in previous examples, the data after the decimal point was lost.
Output
4150 is the data here. 12 is equal to 4150. Data after the decimal point,.12, is lost in this conversion.
This happens because double is a bigger data data type (8 bytes) than data type int(4 bytes), and data loss occurs when we convert data from one type to another.
Similarly, there's a data type hierarchy in C programming. According to the hierarchy, data is lost when a higher data type is transformed to a lower data type, but no data is lost when a lower data type is transformed to a higher data type.
Here,
- Data loss occurs when a long double type is transformed to a double type.
- There is no data loss if char is transformed to int.
Conclusion
We hope you found our post interesting and learned about Type Conversion in C in your own code.