C Programming: From Basic Syntax to Advanced Concepts
In-Depth Analysis of Struct in C
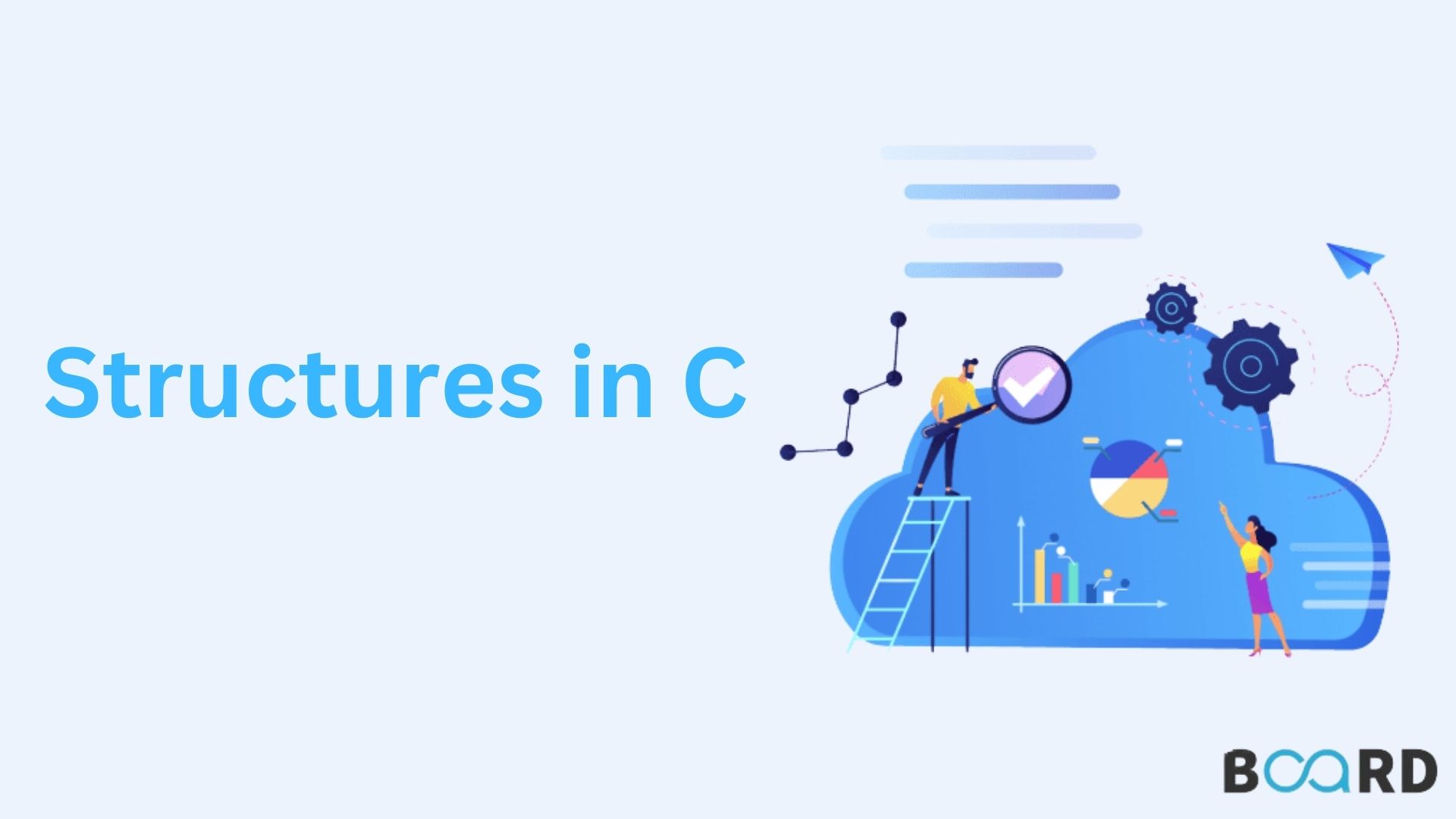
Introduction
Structures (also called structs) are a way to group several related variables into one place. Each variable in the structure is known as a member of the structure. Unlike an array, a structure can contain many different data types (int, float, char, etc.).
Now you must be thinking that how can we make a structure.So, let’s see:
Declaring Structure Variable
A structure variable can either be declared with a structure declaration or as a separate declaration like basic types.
Now you must be thinking that how can we initialize a structure variable. So here is the answer:
The reason for the above error is simple when a datatype is declared, no memory is allocated for it. Memory is allocated only when variables are created.
Accessing Members of the Structure
There are two ways to access structure members:
- By . (member or dot operator)
- By -> (structure pointer operator)
Let’s see one example:
Limitations of Structures
- The C structure does not allow the struct data type to be treated like built-in data types.
- We cannot use operators like +,- etc. on Structure variables.
- No Data Hiding: C Structures do not permit data hiding. Structure members can be accessed by any function, anywhere in the scope of the Structure.
- Functions inside Structure: C structures do not permit functions inside the Structure
- Static Members: C Structures cannot have static members inside their body
- Access Modifiers: C Programming language does not support access modifiers. So they cannot be used in C Structures.
- Construction creation in Structure: Structures in C cannot have a constructor inside Structures.