C Programming: From Basic Syntax to Advanced Concepts
Operator Precedence and Associativity in C
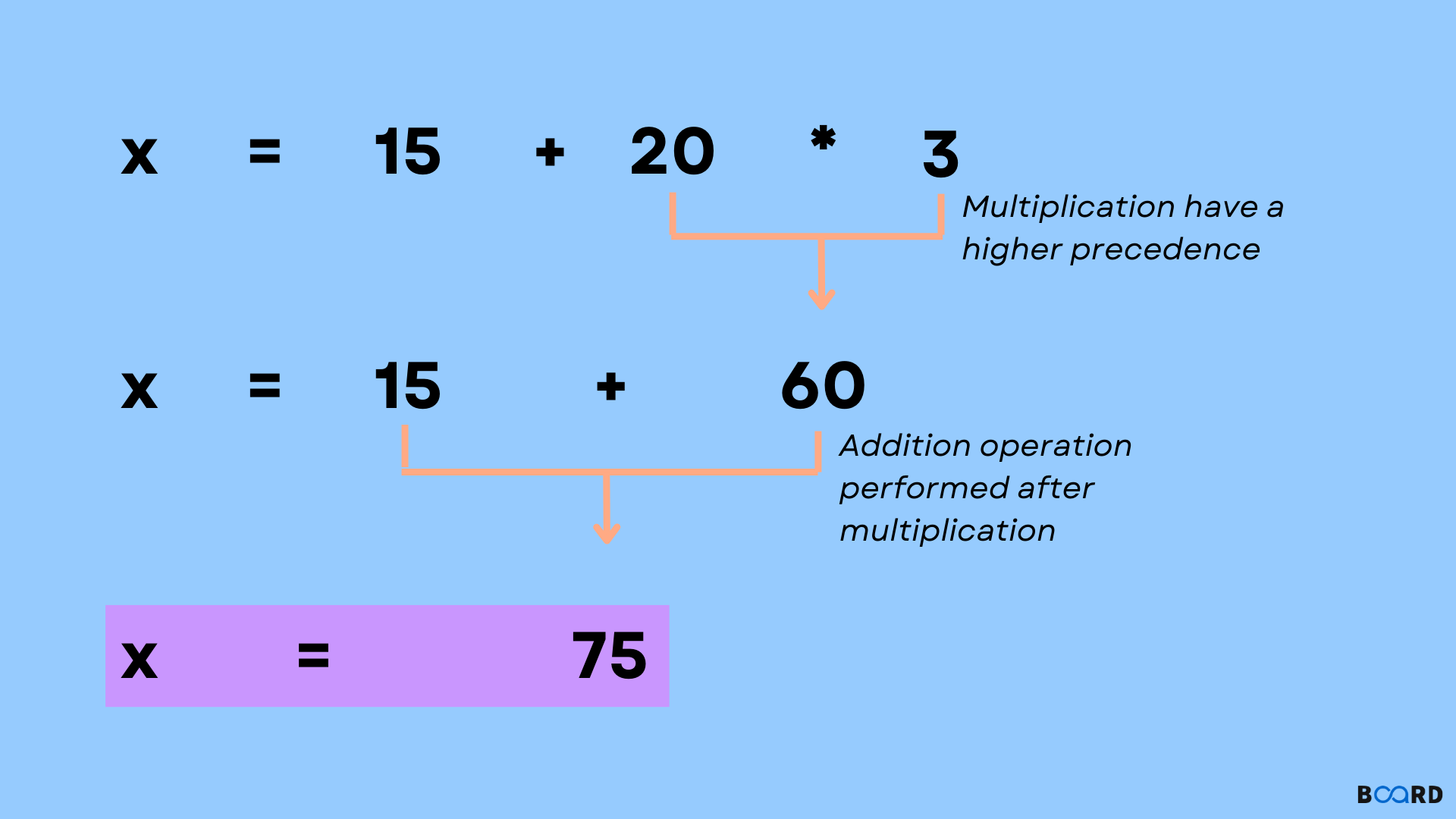
Introduction
The precedence of operators in C determines the order in which the operators in an expression are evolved. Associativity, on the other hand, specifies the order in which operators with the same precedence in an expression are evaluated. Associativity can also occur from right to left or left to right.
When there are no brackets, the letters precedence and associativity are utilized for operators in an expression to determine the order of sub-expressions.
In the event of an expression with more than one operator, operator precedence lets us determine which of the operators in the expression must be evaluated first.
The precedence of * in C is greater than that of - and =. As a result, 17 * 6 is examined first. The phrase using - is then evaluated since the precedence of - is greater than that of = operators Precedence & Associativity Table
Associativity of operators
When two or more operators with the same precedence appear in the same phrase, we employ associativity.
In this case, the value of an is allocated to b rather than the other way around. It's because the = operator's associativity is from right to left.
Furthermore, if two operators with the same precedence (priority) are present, associativity determines which direction they execute.
Consider the following example:
In this case, the operators == and!= have the same priority. As a result, 1 == 2 is executed first.
The preceding expression is equivalent to:
Points to Remember
- Associativity is only used when two or more operators in an expression have the same precedence. It is important to note that associativity does not apply when defining the order of evaluation of operands with varying levels of precedence.
- All operators with a comparable amount of precedence have the same associativity. It is critical because otherwise, the compiler will be unable to determine what order of execution an expression must follow when it contains two operators with the same precedence but differing associativity. For example, the associativity of - and + is the same.
- The associativity and precedence of the prefix ++ and postfix ++ are extremely different. In this case, the prefix ++ has less precedence than the postfix ++. As a result, their associativity is likewise distinct. In this case, prefix ++ associativity is from right to left, while postfix ++ associativity is from left to right.
- We must employ commas (,) with caution since they have the lowest level of priority among all the operators in an expression.
- The C programming language does not provide comparison chaining. Python interprets expressions such as x > y > z as x > y and y > z. In the C program, no such chaining occurs.
Conclusion
We hope you found our post interesting and learned about the precedence and Associativity of Operators in your own code.