C Programming: From Basic Syntax to Advanced Concepts
Function Pointers in C
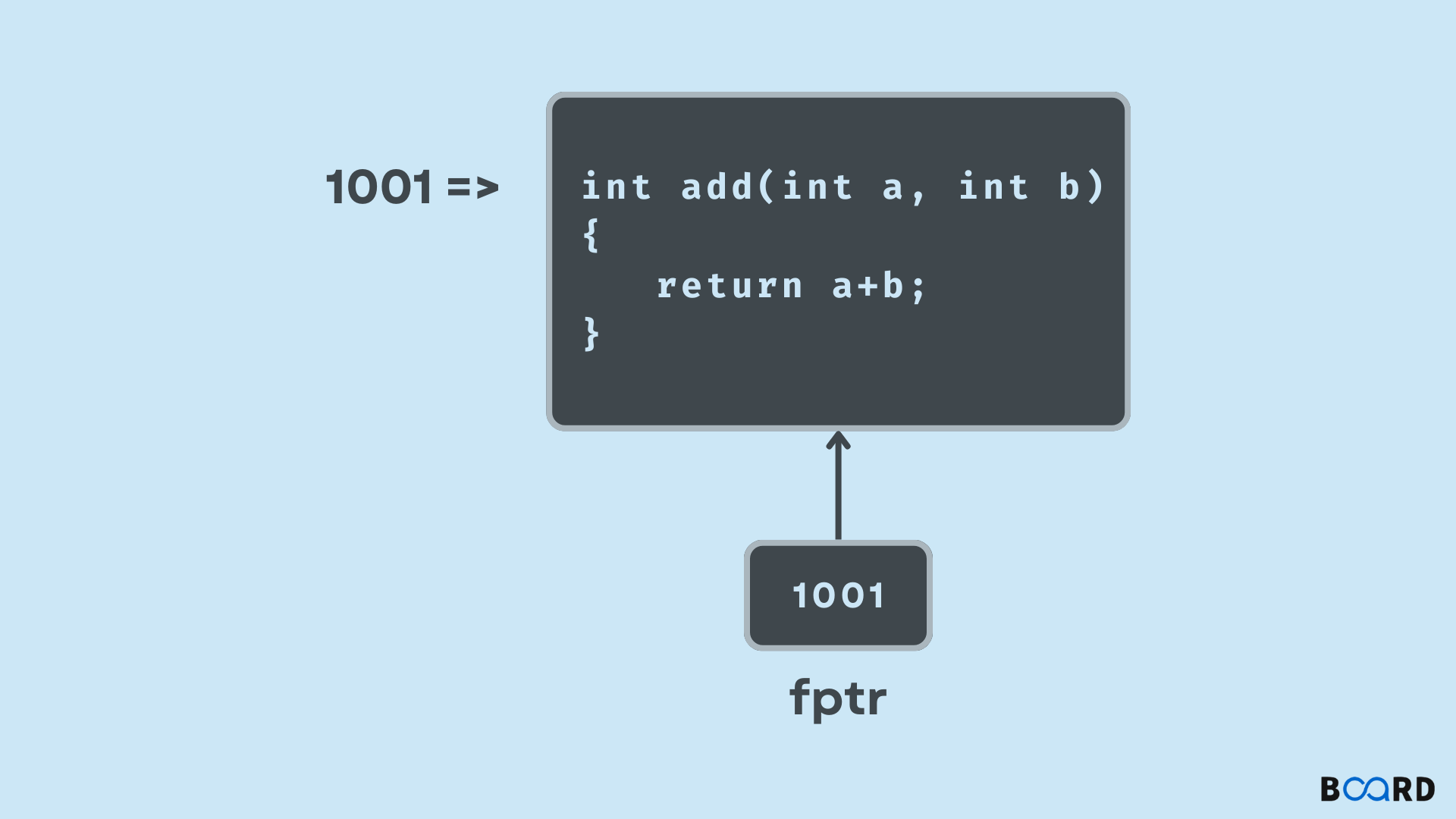
Introduction
C provides us with the concept of Pointers which are variables that can store the address of other variables. It means that a variable that has some valid space in the memory and stores some data can be accessed using its address in the memory.
C also supports the facility of a pointer to a function. In this article, we will learn about the Function Pointers in C. But, before that, you have to be well-versed in the concept of variable pointers. So, we will see how pointers work in C. The following code shows the implementation of a pointer variable.
Output:
Here, the variable ‘pointer’ stores the address of variable ‘a’ and can be used to access the value. You may get confused with “int* pointer” and “int *pointer.” Both are the same, but it is good practice to use an asterisk with the data type of the pointer variable. The reason is that “int*” means a pointer of type ‘int.’ So, an asterisk (*) is not part of the variable, it’s a part of the data- type of the pointer declared.
You can also change the value of a variable without using it using its pointer variable. The following code shows how to change the value of a variable without using it.
Output:
Hence, the value of the variable has been changed from 10 to 11 without using it. This is the utility of pointers. Now, it will be simpler for us to understand the concept of Function Pointers in C.
When you declare a function, it is allocated some space in the stack memory. Thus, each function has a valid address. For example, in the code below, the address of the main function and display function is printed.
Output:
Thus, Function Pointers in C can be used to store the address of a function that is defined in the program. Now, let’s see how to declare and define pointers to function in C.
The syntax of Function Pointers is as follows:
Suppose, there is a function that adds two numbers. Its address can be stored in the following way.
Here, ptrToFunc is the pointer variable that returns ‘int’ and accepts two arguments of type ‘int.’ The output of the code is:
The main point is that a function pointer is a pointer to a block of code, unlike the normal pointers which point to some data. Therefore, a function pointer signifies the starting of a block of code. Also, we cannot allocate and deallocate memory using the pointer to function in C.
Passing address of a function using Function Pointers in C
You can also pass the address of a function as an argument to another function using pointers. In the code given below, there are two functions, function1 and function2. The pointer to function1 and function2 is called with a pointer to function as an argument. Then, the passed pointer is used to call the function1 and after function1 is executed, control is passed to function2 (from where it was called) and finally to the main function.
The output of the above code is given below:
The Array of Pointers to Functions in C
Like the array of pointers, we can also have an array of Function Pointers in C. The syntax to declare an array of pointers to functions is:
The elements of an array of pointers to functions are the same as that of an array, where the array elements are accessed using the index of elements. Below is the C implementation of an array of Function Pointers in C.
The output of the above code is:
As shown in the output, the functions have been called using the array of function pointers in C. Therefore, using pointers you cannot only access the variables, but also the functions which are defined in the program.