C Programming: From Basic Syntax to Advanced Concepts
Dangling Pointer in C
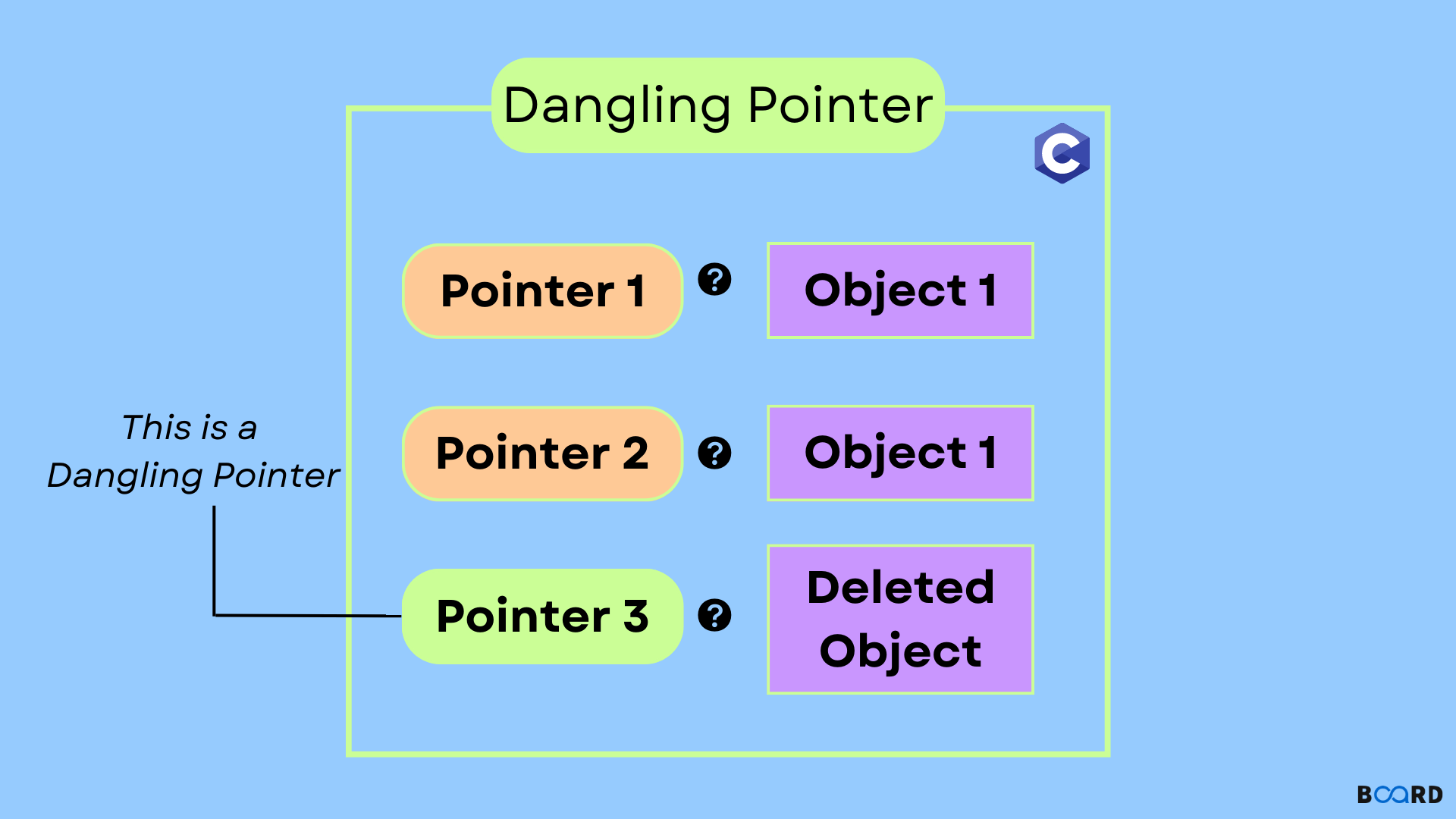
Introduction
A dangling pointer in C is a pointer that points to a memory location that has been deallocated or is no longer valid. Dangling pointers can cause various problems in a program, including segmentation faults, memory leaks, and unpredictable behavior.
One common cause of dangling pointers is using the free function to deallocate memory that was previously allocated using the malloc function. When the free function is called on a pointer, it deallocates the memory pointed to by the pointer, making it available for reuse. However, if the pointer is not set to NULL or reassigned to a different memory location after the memory is deallocated, it becomes a dangling pointer.
Example 1
Here is an example of how a dangling pointer can be created:
In this example, we allocate memory for an integer using the malloc function and assign the allocated memory address to the pointer ptr. We then deallocate the memory using the free function and assign a new value to the memory location pointed to by ptr. However, since the memory has been deallocated, ptr is now a dangling pointer, and accessing the memory location pointed to by ptr can lead to unpredictable behavior.
Another common cause of dangling pointers is using pointers to memory that is automatically deallocated when a function returns or an object goes out of scope. For example, consider the following code:
In this example, we allocate memory for an integer inside the foo function and assign the allocated memory address to the pointer ptr. When the foo function returns, the memory is automatically deallocated, and ptr becomes a dangling pointer. If we try to access the memory location pointed to by ptr after the foo function returns, it can lead to undefined behavior.
Example 2
Here is another example of how a dangling pointer can be created in C:
In this example, we define a pointer ptr and a function get_string that reads a string from the user and assigns it to the buffer array. The function then assigns the address of the buffer array to the ptr pointer and returns it. However, since the buffer array is a local variable and is deallocated when the get_string function returns, ptr becomes a dangling pointer. If we try to access the memory location pointed to by ptr after the get_string function returns, it can lead to undefined behavior.
To avoid this problem, we can allocate memory dynamically using the malloc function or pass a pointer to the caller as an argument. For example:
In this example, we allocate memory for the string using the malloc function and pass the allocated memory address to the get_string function as an argument. The get_string function reads the string from the user and assigns it to the memory location pointed to by the argument. Since the memory is dynamically allocated, it is not deallocated when the get_string function returns, and the pointer str is not a dangling pointer.
To avoid dangling pointers, it is important to ensure they are properly initialized and deinitialized. This can be done by setting pointers to NULL after they have been deallocated and ensuring that pointers are not used after the memory they point to has been deallocated. It is also a good practice to use the free function only on pointers that have been initialized using the malloc function, and to use the calloc or realloc functions instead of the malloc function when necessary.
Conclusion
In conclusion, dangling pointers in C pointers point to memory locations that have been deallocated or are no longer valid. They can cause various problems in a program, including segmentation faults, memory leaks, and unpredictable behavior. To avoid dangling pointers, it is important to ensure that pointers are properly initialized and deinitialized and to use the free function only on pointers that have been initialized using the malloc function.