C Programming: From Basic Syntax to Advanced Concepts
Malloc vs Calloc - Quick Note
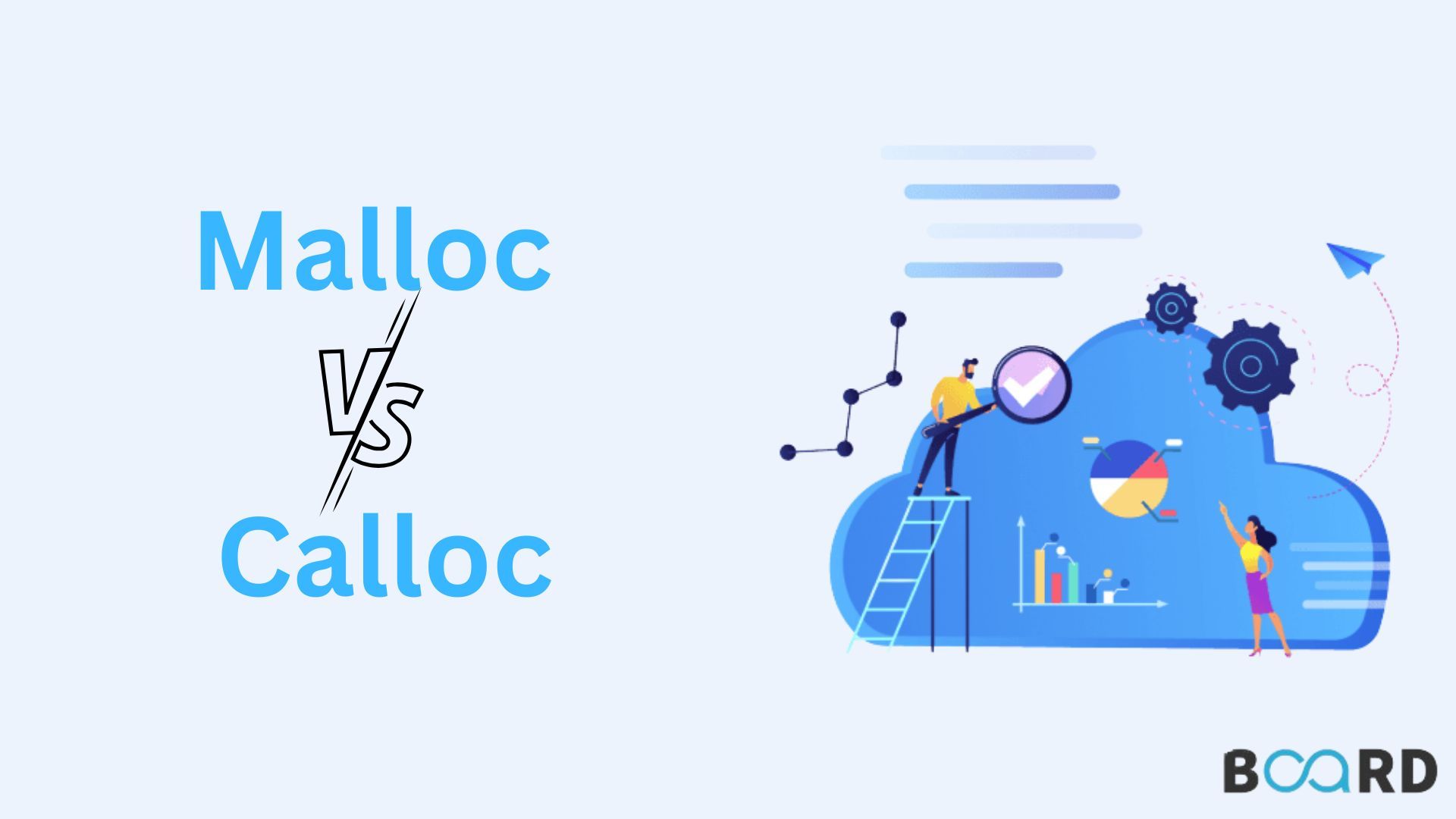
Introduction
The primary distinction between the calloc() function and malloc is that the latter requires two parameters, while the former just requires one (). The C programming language offers the methods malloc() and calloc() for dynamic memory allocation and deallocation during runtime. Let's first grasp the definition of dynamic memory allocation before learning how to use the malloc() and calloc() routines. The process of allocating computer memory for the use of applications and processes is known as memory allocation. When the amount of memory a program or process will use is unknown in advance, we employ dynamic allocation strategies. Due to issues with static memory allocation, such as what happens if fewer elements are saved than expected with the rest of the RAM, dynamic memory allocation was created. As a result, it solves the issues with static memory allocation, where memory is only allocated as needed.
Comparison Chart
Definition of malloc()
A block of memory is assigned in bytes using the malloc function. The block size that is needed for use must be specified expressly by the user. The program uses the malloc function to request memory allocation from the system's RAM, and if the request is granted (i.e., the malloc function reports that memory allocation was successful), it provides a reference to the first block of memory. Any kind of pointer can be assigned because it returns a void type of pointer. However, if the malloc function is unable to allocate the necessary amount of memory, it returns a NULL. The header file alloc.h or stdlib.h contains access information for the malloc function.
SYNTAX
malloc(total elements *size of individual element);
Eg.
int *ptr;
ptr=malloc(10*sizeof (int))
where size denotes the amount of RAM needed in bytes. The aforementioned declaration might be expressed in the following form: But as was previously explained, the method malloc returns a void pointer, thus a cast operator is needed to convert the resulting pointer type to suit our needs.
ptr_var=(type_cast* ) malloc (size) where,
ptr_var = the pointer's name, which stores the allocated memory block's initial address,
type_cast =the data type that the provided pointer (or type void) should be converted to, and size gives the size in bytes of the memory block that was allotted.
Eg.
int *ptr;
ptr=(int*) malloc (10 * size of (int));
The malloc function allocates memory using junk data. Be aware that you should check if the matching request made by malloc to allocate memory from the system RAM was accepted or denied. We may take advantage of the fact that the malloc method returns a NULL when the required amount of memory is not provided.
Definition of calloc()
With the exception of the fact that malloc() only takes one parameter, the calloc method functions exactly the same as malloc().
Eg.
int*ptr;
ptr = (int*)calloc(10,2);
In this scenario, the size of the data type in bytes that we want the allocation to be done for is specified by the number 2, which in this case is 2 for integers. Additionally, 10 is the total number of items for which allocation is required. Do not get confused since numerous arguments are usually separated by commas; instead, keep in mind that the parameter supplied to the method malloc was (n*10). There are no commas in the argument (n*10). Hence it is a single argument, though not a simple one but an expression.
A 20 byte memory block is allocated to the requesting application once the aforementioned statement has been executed. The requesting programme is then given the address of the first block, and the pointer ptr is given the address of the first block. All the zeros in the memory that the calloc function allotted. Additionally, the calloc function may be found in the header files stdlib.h or alloc.h.
Key Differences
The following are the main variations between the calloc and malloc functions:
- Malloc assigns a single block of requested memory, whereas calloc allocates several blocks of requested memory.
- The malloc method initializes the memory that has been allocated without clearing it. It is filled with junk, and the allocated memory's contents cannot be changed. Calloc, on the other hand, starts the memory allocated at zero.
- Malloc is quicker than calloc since calloc needs to go through more setup stages, although the speed difference is small.
- Another distinction between the two is that calloc is a malloc+memset, whereas malloc simply assigns memory from the heap inside this virtual address, and memset also allocates the physical pages in memory.
Conclusion
Both the malloc and calloc functions, which are used to allocate memory, have their advantages and disadvantages. For example, malloc is faster than calloc. Malloc is also simpler to use since it only requires one parameter, unlike calloc, which allocates memory and initializes the memory space with ZERO. However, when variable initialization is more crucial to you, you would choose to utilize calloc.