Advanced JavaScript: A Deep Dive into the Core Concepts
A quick guide to async/await in Javascript
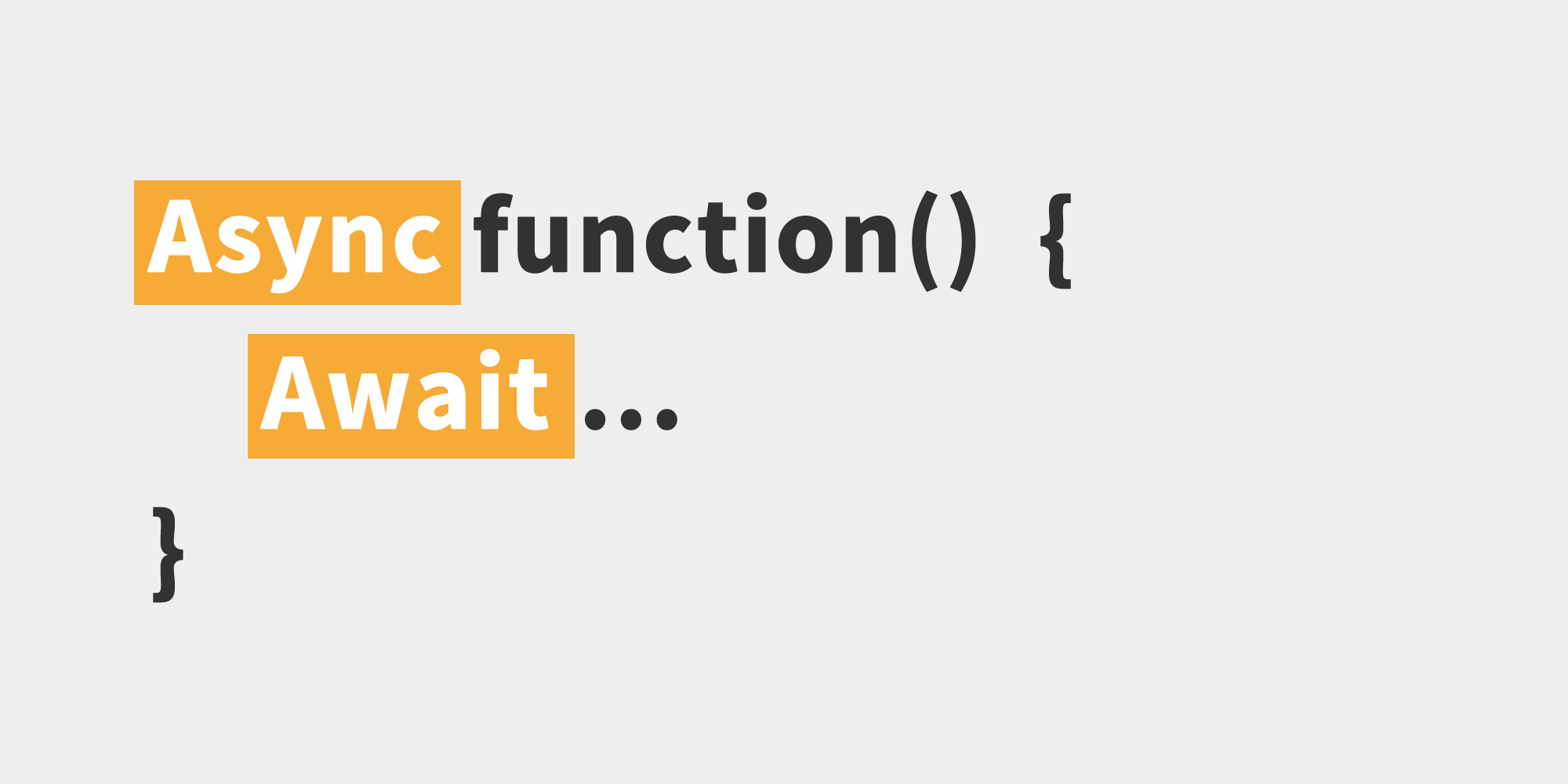
Introduction
To indicate that a function is an asynchronous function, we use the async keyword with the function. The promise that the async function returns.
The Async function syntax is as follows:
Here,
- name - the function's name
- parameters - Information provided to the function via parameters
Async Function
Output:
The async keyword is used before the function in the aforementioned program to indicate that the function is asynchronous.
Since this function produces a promise, the following example shows how to utilize the chaining technique then():
Output:
The then() procedure is carried out in the aforementioned programme once the f() function has been resolved.
Await Keyword in JavaScript:
The async function uses the await keyword to wait for the asynchronous action.
The appropriate syntax for await is:
When await is used, the async function is suspended until the promise's outcome (a resolve or reject value) is returned. For instance,
Output:
A Promise object is generated in the programme above, and it is resolved 4000 milliseconds later. Here, the async function is used to create the asyncFunc() function.
The await keyword watches for the fulfillment of the promise (resolve or reject).
Hello is therefore only shown once the promise value has been made accessible to the result variable.
If await is not used in the application above, hello is shown before the Promise is resolved.
Note: Only async methods may contain await.
The async function enables the execution of the asynchronous method in a manner that seems synchronous. Despite being asynchronous, the operation seems to be carried out synchronously.
If the program has several promises, this may be helpful. For instance,
Advantages of Async Functions:
- Compared to calling a callback or a promise, the code is easier to read.
- Handling errors is easier.
- Testing is simpler.
Note: The more recent version of JavaScript added the terms async and await (ES8). The usage of async/await may not be supported by some older browsers. Visit JavaScript async/await browser support for additional information.