Advanced JavaScript: A Deep Dive into the Core Concepts
JavaScript: Array Filter() Method
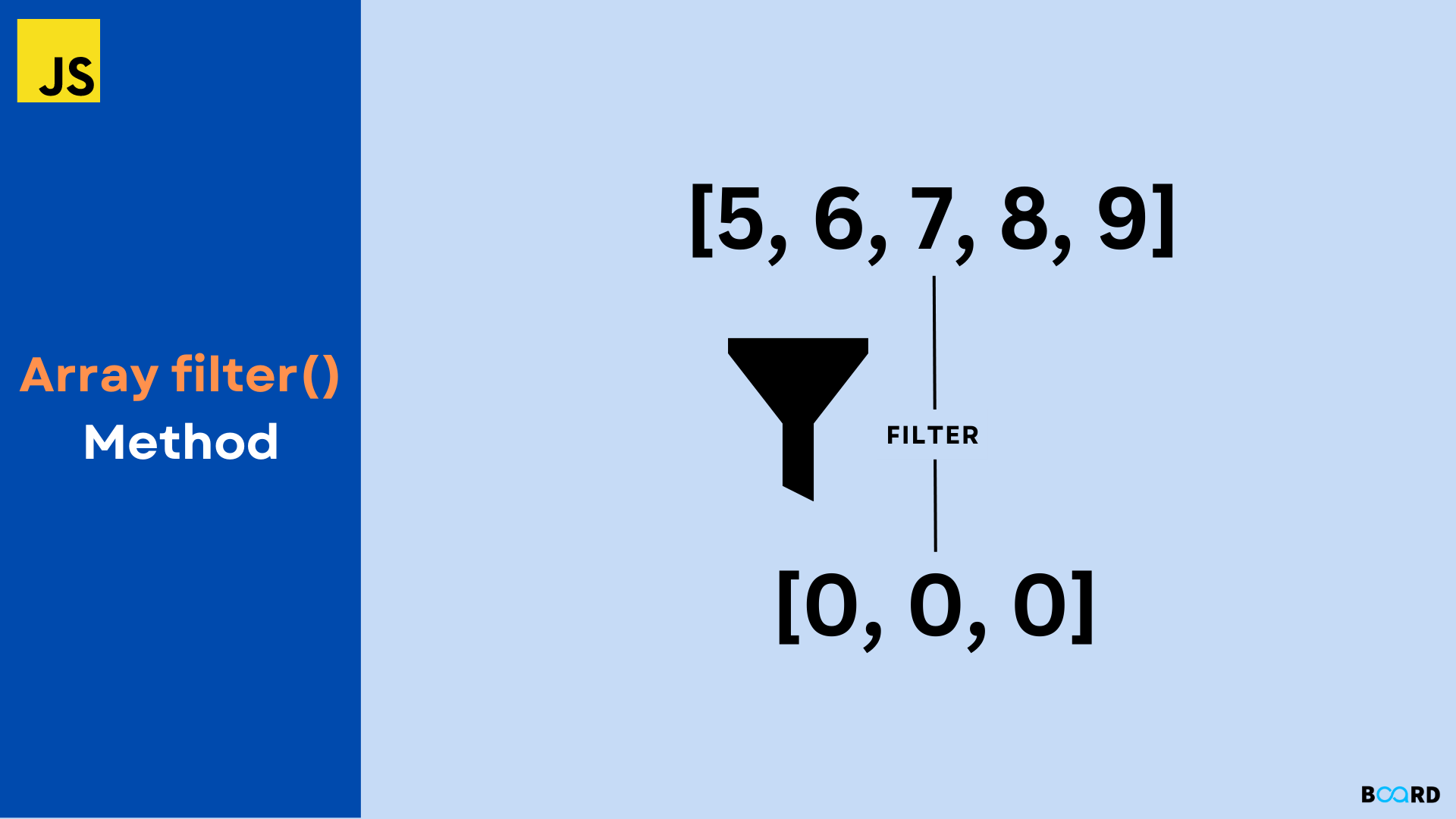
Introduction
The arr.filter() method is used to create a new array from a given array consisting of only those elements from the given array which satisfy a condition set by the argument method. If no condition is specified, it applies no filtering and simply returns an array with all elements from the passed input array. The array filter() method is a powerful and flexible way to slice and dice your data. It's useful for finding specific values in an array based on the criteria you specify. The JavaScript array filter() method lets you filter an array of values based on a condition.
Points to Remember
The Array.filter() method returns a new array that contains all of the elements in the original array for which the specified condition is true, discarding any elements for which the specified condition isn't true.
The filter() method creates a new array filled with elements that pass a test provided by a function.
Array filter() takes five parameters:
Index :(Optional) The index of the current value element in the array starting from 0.
Arr : (Optional) it holds the complete array on which Array. every is called.
Element: The parameter holds the value of the elements being processed currently.
thisValue : (Optional) Default undefined A value passed to the function as its this value.
Callback: This parameter holds the function to be called for each element of the array.
The callback function is called with element, index, arr, thisValue arguments: the value you want to return if the condition is true, or undefined if it isn't. The array argument contains your original array of values.
The syntax for using filter() looks like this:
where obj1, obj2, ..., objN are your original values.
OR
Examples:
Example 1:
Output:
First element and query both are converted to lowercase and the indexOf() method is used to check if query is present inside element. Those elements that do not pass this condition are filtered out.
Example 2:
Output:
The numbers less than 200, and all the non numeric values are filtered out.
Conclusion:
This method simply takes a function as an argument, runs it over each element of the array you wish to filter, and returns the resulting subset. The arr.filter() method creates a new array from scratch based on the filters you provide. The filter() method of an array is a way to remove items from the array based on a condition.