Advanced JavaScript: A Deep Dive into the Core Concepts
What is Promise.all in JavaScript?
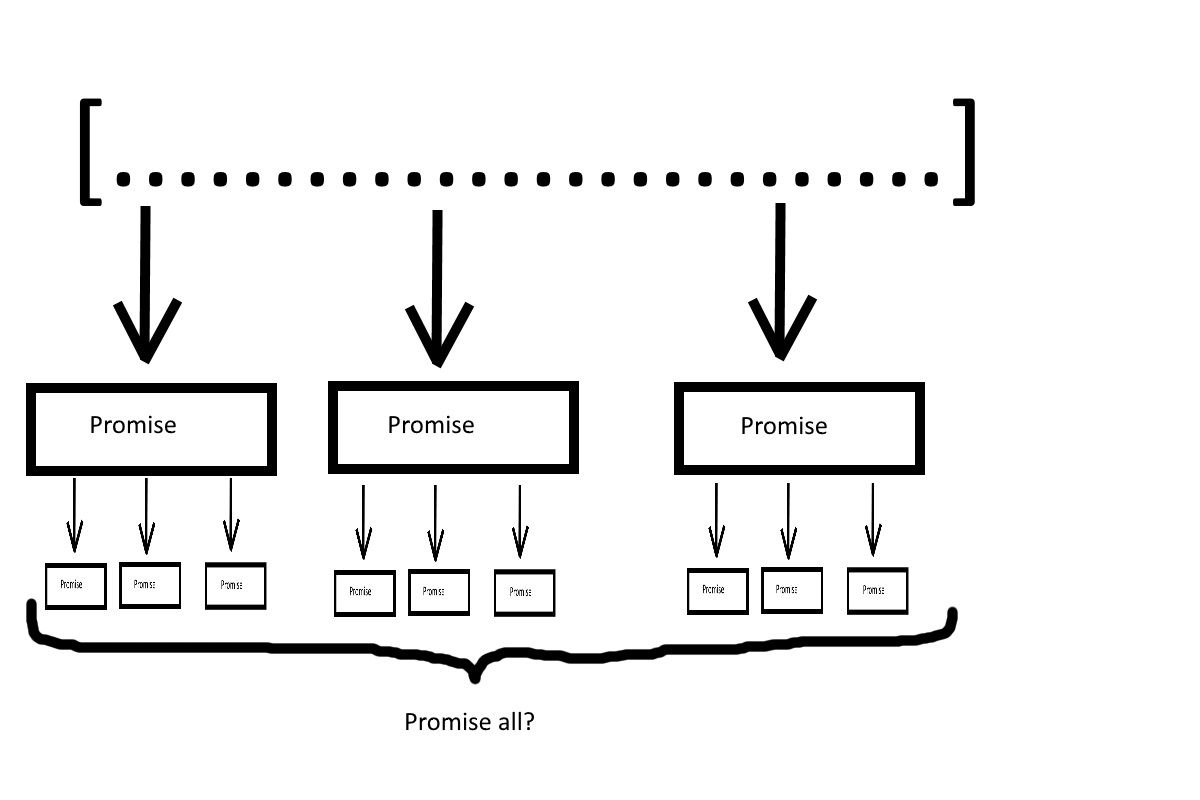
Introduction
An iterable of promises is sent into the Promise.all() function, which produces a single Promise that resolves to an array of the promises' outcomes. When every promise in the input has been completed or if there are no promises in the input iterable, the promise that was returned will be fulfilled. Upon any of the input promises being rejected or upon a non-promise throwing an error, it rejects right away and will reject with this first rejection message/error.
Syntax
promise.all(iterable)
Parameters:
- Iterable - an item with iterations, such as an array.
Return value:
If the iterable passed is empty, a Promise has already been fulfilled.
If the iterable supplied has no promises, then an asynchronously fulfilled Promise will be returned. Notably, Google Chrome 58 in this instance returns a promise that has already been met.
In all other instances, a pending Promise. When every promise in the returned iterable has been fulfilled or rejected, or if any of the promises reject, this returned promise is then fulfilled or rejected asynchronously (as soon as the queue is empty). Please refer to the example below regarding "Asynchronicity or synchronicity of Promise.all." Regardless of the complete order, returned values will be in the order of the Promises supplied.
Description
This technique may be helpful for combining the outcomes of many promises. It is often utilized when there are several interconnected asynchronous activities that must all be completed before the code execution can move on in order for the entire program to function correctly.
If any of the supplied promises are rejected, Promise.all() will instantly return false. In contrast, regardless of whether any of the input promises are rejected, the promise delivered by Promise.allSettled() will wait for all of them to finish. The end result of each promise and function from the input iterable will thus always be returned.
When this procedure is finished, the promise array's order is maintained.
Examples
Using Promise.all:
Promise.all waits for all fulfillments (or the first rejection).
Non-promise values will be disregarded if they are included in the iterable, but they will still be included in the value of the returned promise array (if the promise is kept):
Promise.all - synchronicity or asynchronicity:
The following illustration shows how Promise.all may be asynchronous (or synchronic, if the iterable provided is empty):
However, the provided iterable must be empty for Promise.all to resolve synchronously:
Promise.all fail-fast behavior:
If any of the parts are refused, Promise.all is also rejected. For instance, Promise.all will reject right away if you feed it five promises that resolve after a timeout and one promise that rejects right away.
This habit can be altered by how you respond to potential rejections:
Hope this gave you a clear understanding of the concept.