Advanced JavaScript: A Deep Dive into the Core Concepts
Promise in JavaScript
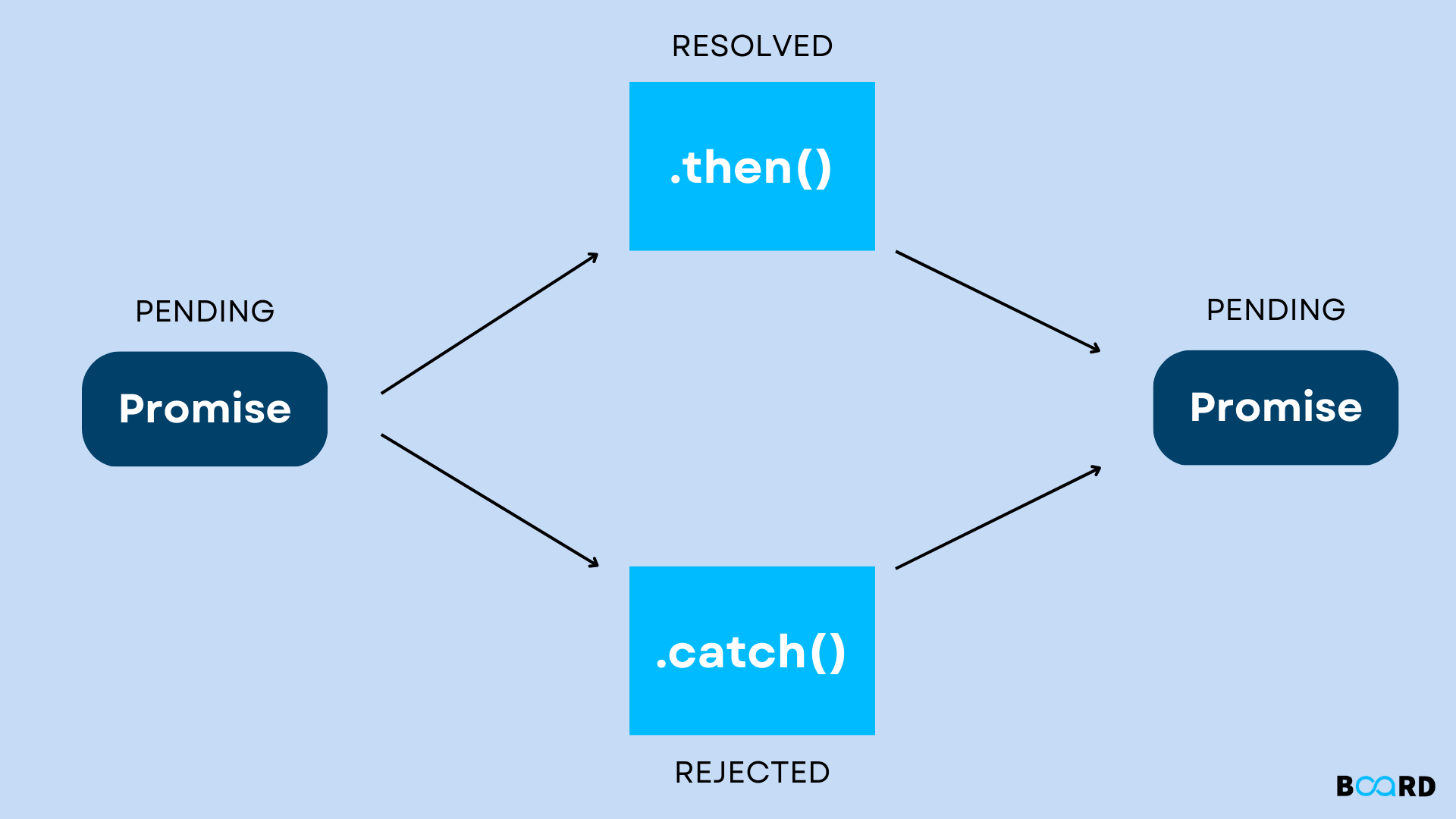
Introduction
Simply said a promise is a function that returns an Object to which callbacks can be attached.
Only when the operation is finished will a promise object's callbacks be activated. Callbacks must wait till the procedure is finished or declined:
A promise must pass through several phases before it settles (it either is fulfilled or is rejected):
A promise's initial state when it is established is pending. The promise is then either fulfilled or denied based on the operation's outcome.
The callback that will be made based on each promise state is clearly displayed in the table above:
How to Write JavaScript Promises?
JavaScript's promise function Object() { [native code] } is used to create promises. A function with two parameters, resolve and reject, is the only argument required by the function Object() { [native code] }.
Then, by adding the callbacks, we can use our new_promise:
Rejected promises in JavaScript
A promise can also be rejected. Rejections typically happen because JS ran into a problem when executing the asynchronous code. In this case, it makes use of the refuse() method.
Here is a short and fabricated illustration of how a promise may be turned down:
Ways to Connect Promises with then()
You normally want to perform something with the return value when the promise eventually delivers a value ().
For instance, you would want to obtain the value and show it to the user on the website if you were sending a network request.
Two callback methods that you wish to be invoked when a promise is accepted or refused can be defined. These routines are defined within a then() method that is nested:
When this code is executed, the following fulfillment message will appear in the console after three seconds:
You should be aware that you can nest as many promises as you like. Following the preceding step, each subsequent step will be carried out, incorporating the result of the preceding phase:
However, we have overlooked something crucial.
Always remember that a then() function must accept both a rejection handler and a fulfillment handler. In this way, if the promise is kept, the first party is called, and if the promise is broken due to an error, the second party is called.
A second handler is not present in the promises in code examples 4 and 5. Therefore, if an error were to occur, there would be no rejection handler to deal with it.
It is necessary to nest a catch() method at the bottom of the promise chain if you are only going to declare one callback function (also known as a fulfillment handler) in then() in order to capture any potential failures.
What is the catch() Method in JS Used For?
When an error occurs anywhere along the promise chain, the catch() function is always invoked:
The method specified in the nested then() will not be used since myPromise will ultimately resolve to a rejection. Instead, the catch(error )'s handler will be activated, and it should output the subsequent error message to the console:
Conclusion
The extremely potent feature of JavaScript promises makes it possible to execute asynchronous programming in JavaScript. Your interviewer will undoubtedly inquire about promises in most, if not all, interviews for positions requiring JavaScript.