Advanced JavaScript: A Deep Dive into the Core Concepts
A quick guide to Debounce in JavaScript
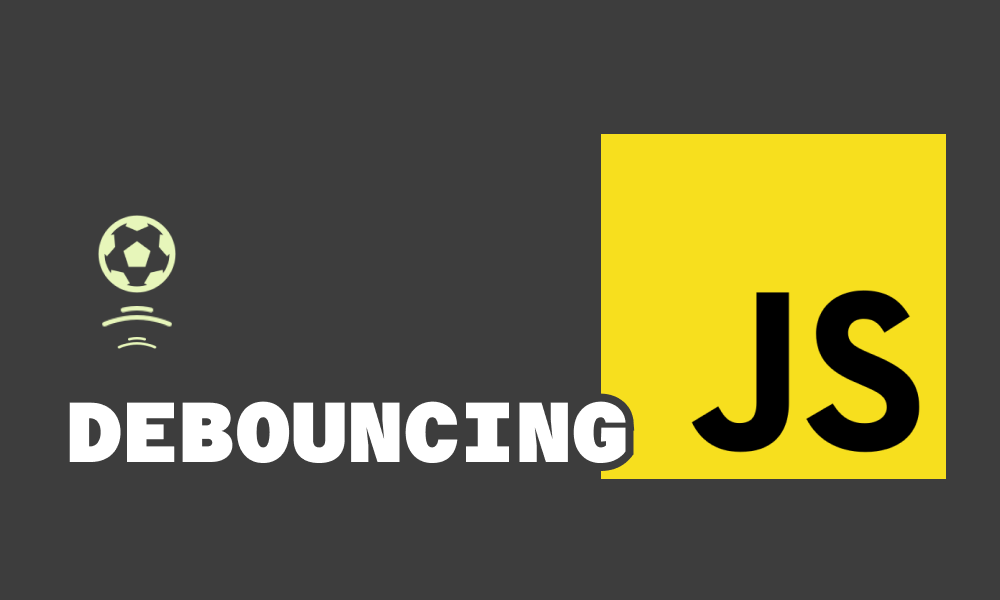
Introduction
JavaScript uses the debouncing technique to speed up browser performance. Some features on a web page could require laborious computations. The fact that JavaScript is a single-threaded language means that if this type of technique is used frequently, it could have a significant impact on the browser's performance. Debouncing is a programming method that ensures time-consuming tasks do not result in performance drops for the website. In other words, when called, the Debounce methods are not executed. Instead, they delay execution for a set amount of time. The previous process is stopped and the timer is reset when we call the same process again.
Example:
Output
Explanation
The debounce function is called by an event listener that is connected to the button. There are two parameters given to the debounce function: a function and a number. Create a variable named debounce timer, which purpose is to call the method after a delay of 'delay' milliseconds.The debounce function is called after the delay if the debounce button is only clicked once. The first delay is cleared, though, and a new delay timer is begun if the debounce button is clicked once, then again just before the delay expires. To do this, the clearTimeout function is employed.
Debouncing's fundamental principle is to:
1. Start with a timeout of 0.
2. Reset the timer to the desired delay in case the debounced function is called once more.
3. Call the debounced function in the event of a timeout.
Every time a debounce function is called, the timer is reset and the call is delayed.
Application:
Debouncing can be used to implement suggestive text, in this case the text is proposed after we wait a brief period for the user to cease typing. As a result, we give each keystroke a few seconds before offering a suggestion. On websites that load content while the user scrolls, like Facebook and Twitter, debouncing is frequently employed. If the scroll event is fired too frequently, it will affect performance because there are so many movies and photographs. Debouncing is therefore required in the scroll situation.
Conclusion:
We learned about debouncing ideas in this post, along with how they are applied in the real world. JavaScript and HTML were also used to put the ideas into practice.