Advanced JavaScript: A Deep Dive into the Core Concepts
Understanding Array Slice() in JavaScript
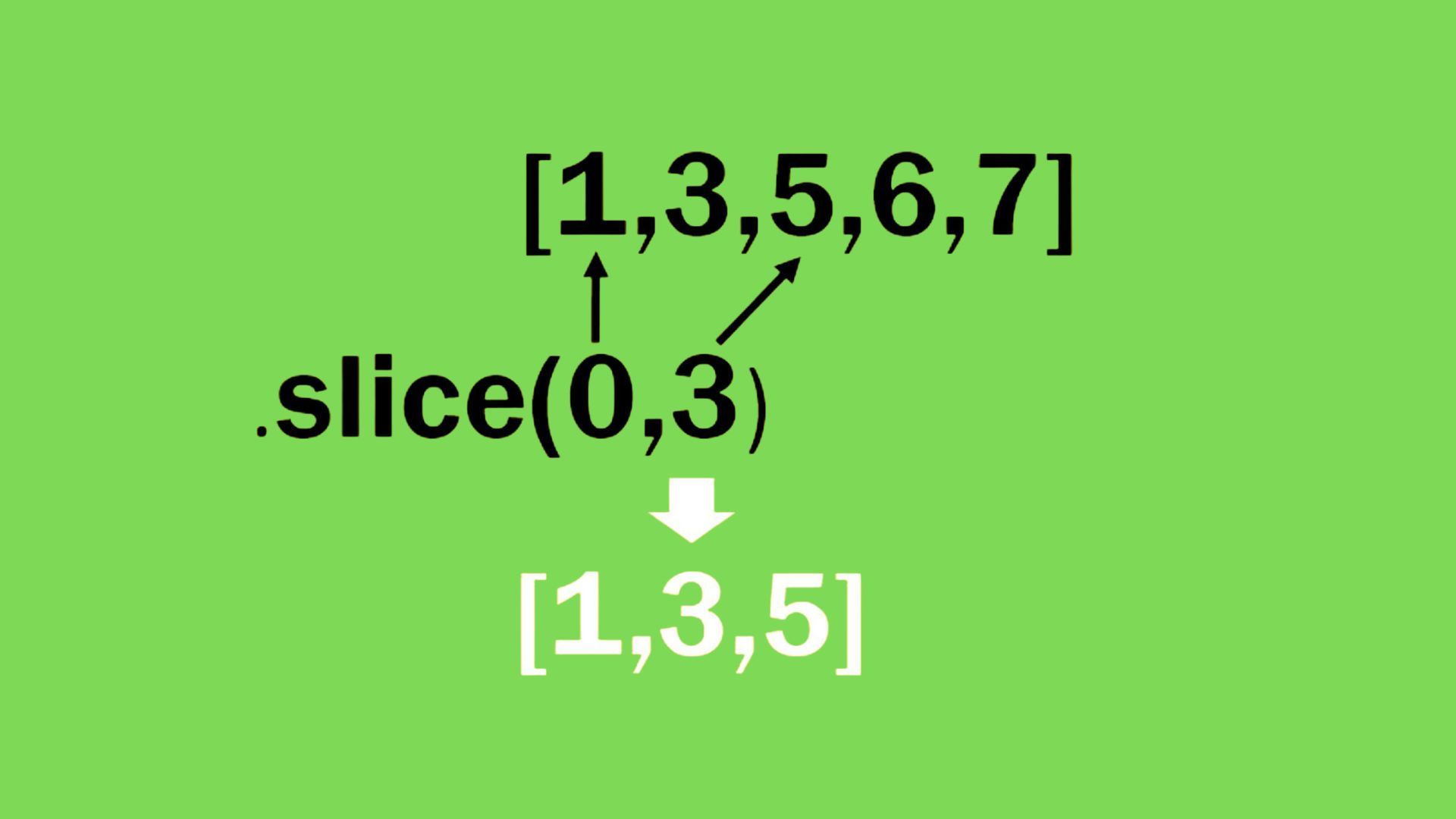
Introduction
With the assistance of examples, we will learn about the JavaScript Array slice() technique in this article.
Slice() creates a new array object by making a shallow duplicate of a specific area of an array.
Example:
Output:
slice() Syntax
The slice() method's syntax is as follows:
arr.slice(start, end)
The array arr is used here.
slice() Constraints:
Slice() function includes:
- start (optional) - The selection's initial index. Unless otherwise specified, the selection begins at start 0.
- end (optional) - The selection's final index (exclusive). The selection stops at the final element's index if it is not given.
Return value for slice():
Returns a new array that contains the elements that were removed.
Examples
Example 1: The slice() function in JavaScript.
Output:
Example 2: Negative index slice() in JavaScript
You may also use negative start and end indices with JavaScript. The final element's index is -1, the next-to-last element's index is -2, and so on.
Output:
Example 3: Objects as Array Elements in JavaScript's slice()
The array's elements are shallowly copied using the slice() function as follows:
- References to objects are copied to the new array. (For instance, a nested array) As a result, any modifications to the referenced object are reflected in the newly returned array.
- It duplicates the value of strings and numbers to the new array.
Output:
Hope this gave you a clear understanding of the concept. To check out more topics in Javascript, visit our Discussion Forum.