Advanced JavaScript: A Deep Dive into the Core Concepts
JavaScript array map() method
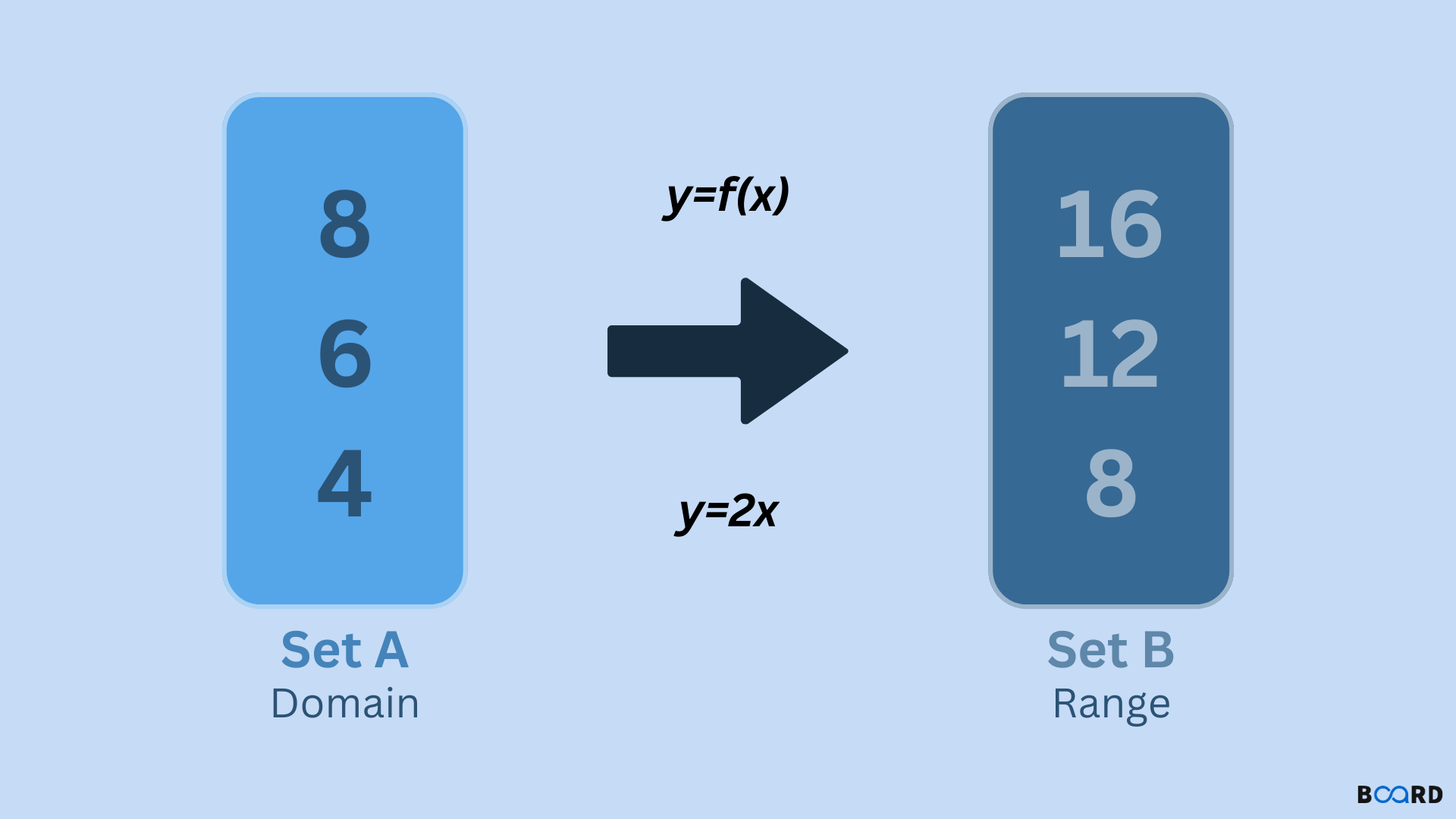
Introduction
The array map() method transforms an array into a new array with each element mapped to the value of its corresponding key. The result is usually not an entirely new array, but only a flattened version of the original one.The Array.map() method allows you to iterate over the items of an array, transforming each item in turn. The array map() method aggregates elements of an array and applies a function to those values. The map method returns a new array with an updated collection of the result.
It accepts two parameters:
Function: It is required parameter and it runs on each element of array.
thisValue: It is optional parameter and used to hold the value of passed to the function.
The map() method can be used to execute a function once for each item in the array. The function is executed with three arguments: the value of the current item, the index of that item in the array, and the whole array itself.
currentValue: (required)it holds the value of current element. index: (optional) It holds the index of current element.
Arr: (optional) parameter and it holds the array.
Map() return value:
Return a new array with elements as the return value .
Syntax
Here's how it works:
First, we pass our function as a string. That string will be run once for each element in our original array. The result of this mapping is then passed back into our function and returned as a new array with all the results of where we mapped them.
Examples
Example 1:
Output:
Example 2:
Output:
Example 3:
Output:
The map() method is used to iterate over a JavaScript object's properties and create new arrays. Since JavaScript does not support polymorphic behavior, the result of this iteration is limited to being a single array value.
Conclusion
The JavaScript array map() method creates a new array with the result of applying a function to each element of an existing array. This method is commonly used as an iterative processing step, where many elements are processed in parallel in a computationally efficient way.