Class Methods in Python
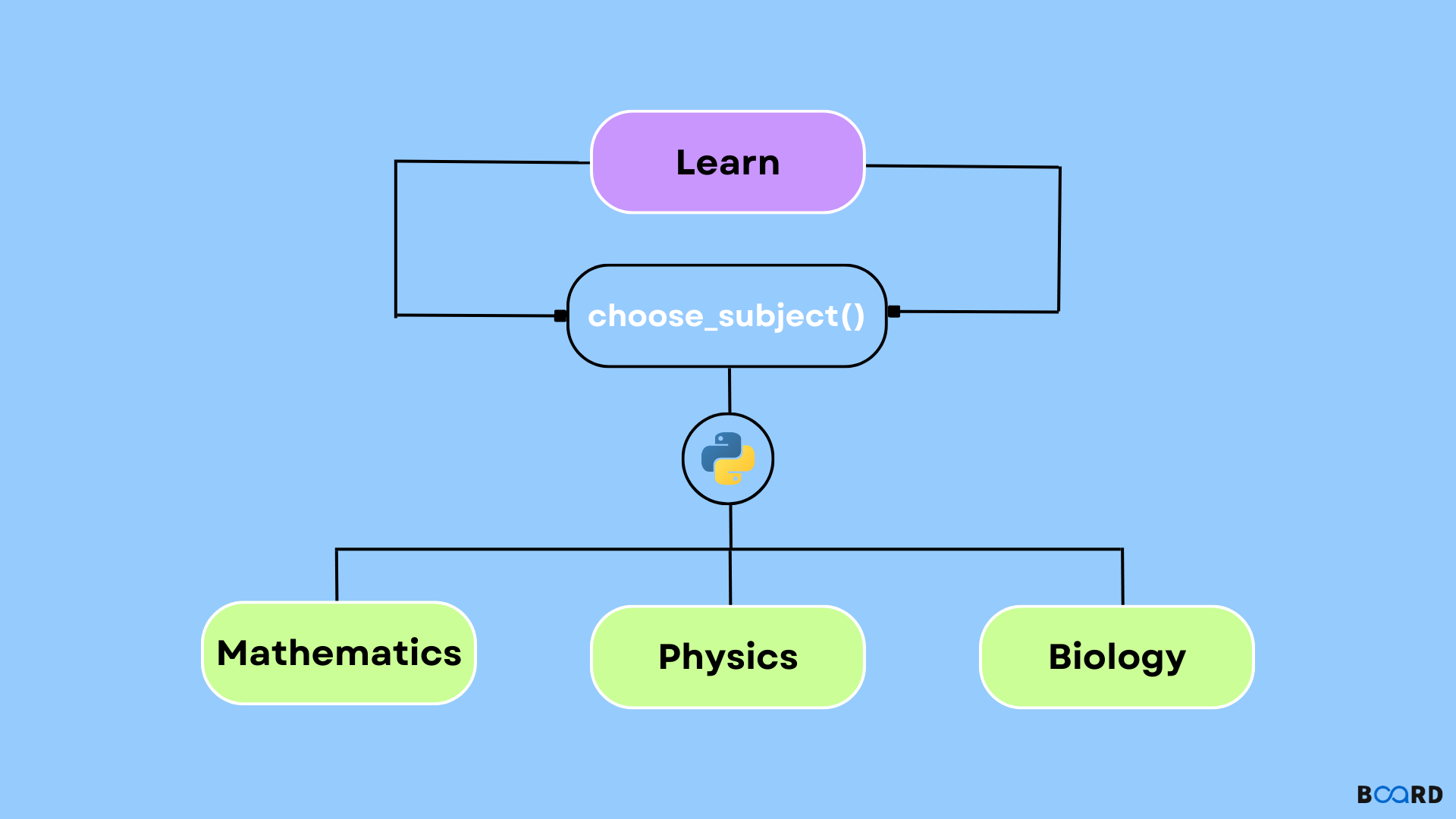
Introduction
In Python, usually we use class-specific methods called instance methods that are connected to instance variables. Within the same class, instance methods have access to instance variables. You must first build a class instance in order to call instance methods. The Person class is described as follows:
Three instance methods, including __init__(), get full name(), and introduce, are available for the Person class (). Consider adding a function to the Individual class that produces an anonymous person. To do this, you would create the following code:
An anonymous person is returned by the instance function create_anonymous(). However, creating an instance is required in order to use the create anonymous() function, which is unnecessary in this situation. Python class methods are useful in this situation. A class method has no instance-specific restrictions. It is restricted to the class alone.
Definition of a class method
- Prior to the method declaration, insert the @classmethod decorator. For the time being, all you need to know is that an instance method will become a class method when the @classmethod decorator is used.
- The self parameter should then be renamed to cls. The cls means class. Class, however, cannot be used as a parameter because it is a keyword.
The updated Person class is displayed in the following way:
The create anonymous() method does not have access to instance attributes. However, it can access class attributes thanks to the cls variable.
Calling Python class methods
You must use the class name, a dot, and the method name as shown in the example below to call a class method:
The create anonymous() class function of the Person class is demonstrated by the following example:
Class methods vs Instance methods
The distinctions between instance methods and class methods are shown in the table below:
When to use Python class methods
Any methods that aren't tied to a single instance but rather the class can be implemented using class methods. For methods that produce a class instance in real life, you frequently utilize class methods. A method is referred to be a factory method when it makes an instance of a class and returns it. For instance, the create anonymous() function, which creates a new instance of the Person class and returns it, is a factory method.
Summary
- Python class methods are connected to classes rather than any particular instance.
- To convert an instance method to a class method, use the @classmethod decorator.
- Additionally, give the class method the cls as its first parameter. For factory methods, use class methods.