Selection Sort In Python
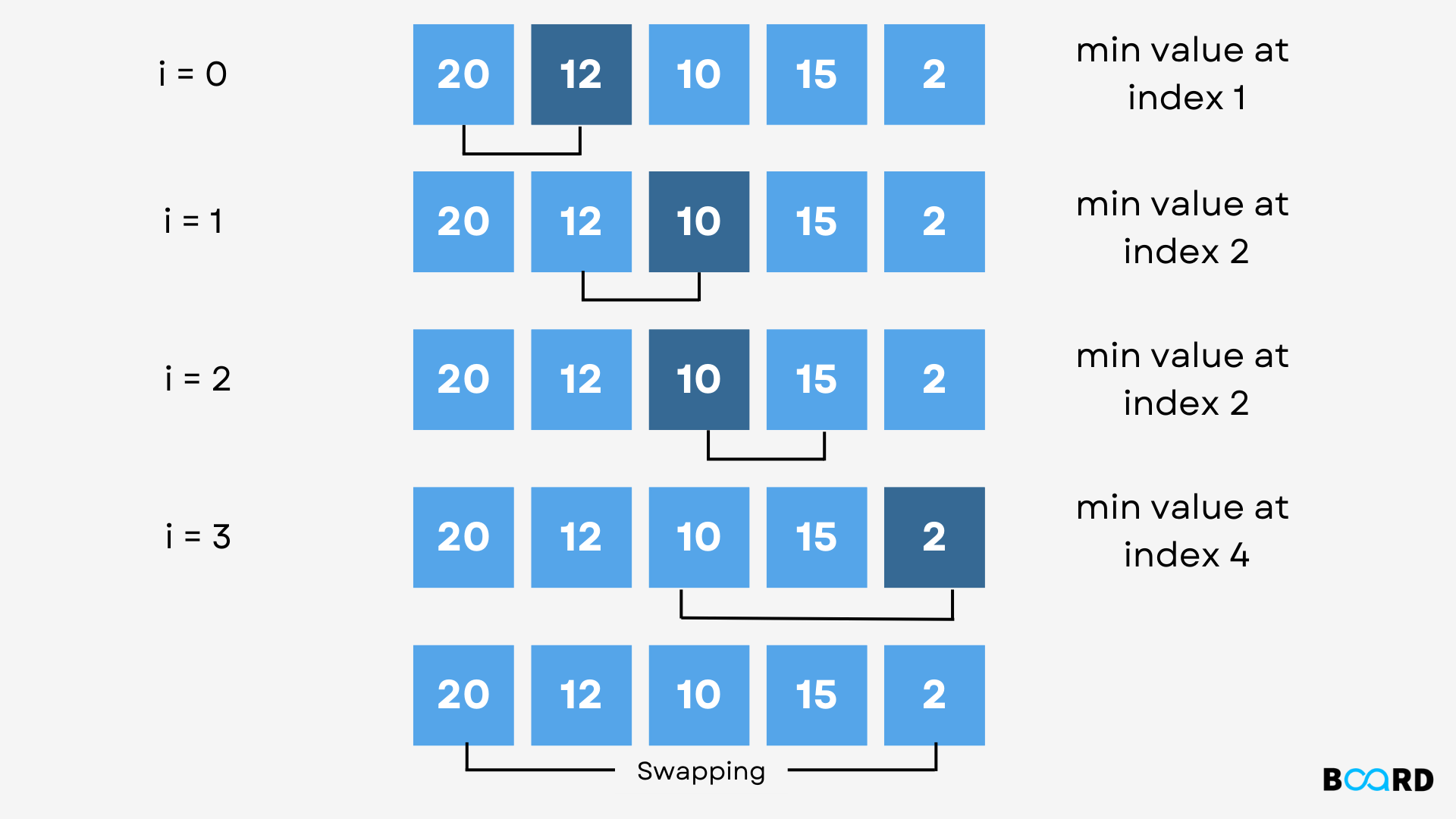
Overview
In this article, we shall learn about the selection sorting algorithm in Python. Selection sorting is an algorithm that sorts a given array by repeatedly searching for the smallest/largest element (depending upon the order of sorting) in an unsorted portion of the array, and then placing the found element at the start.
Scope
- The concept of selection sorting.
- Implementing selection sort in Python.
- Time complexity of selection sort.
- Space complexity of selection sort.
Concept
The selection sort algorithm operates by maintaining a partition of the given array into the following two arrays, at any point:
- The subarray which is already sorted.
- The remaining subarray, which has yet not been sorted.
In each of its iterations, the selection sort finds the smallest/largest element in the unsorted subarray and moves it to the sorted subarray.

Image Courtesy: technologystrive
Implementation
Output
write your code here: Coding Playground
Time Complexity
O(n2)
Space Complexity
O(1)