HashMap in Python
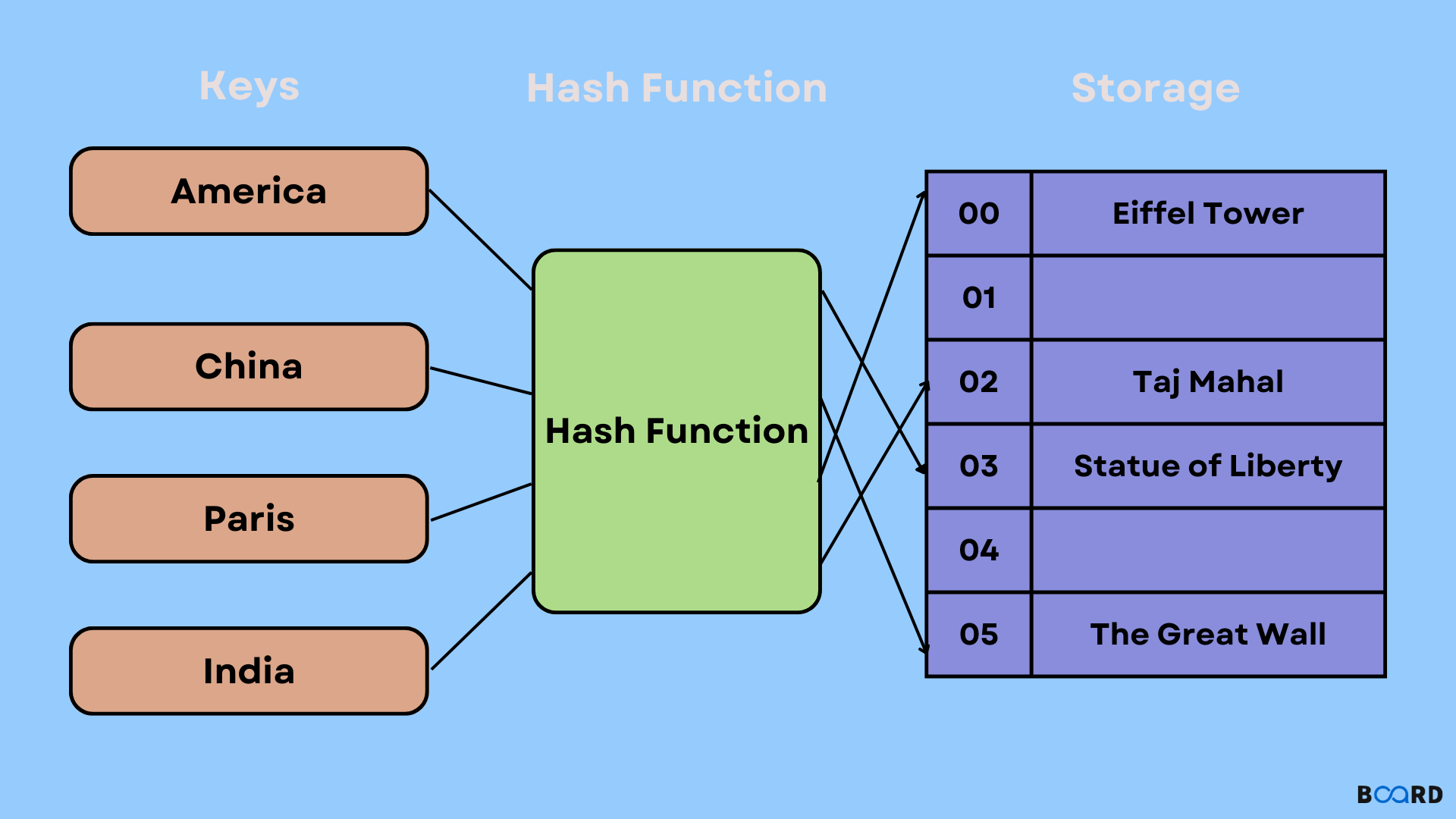
Introduction
Hash maps are the indexed Data Strutures. When creating an index with a key into an array of buckets or slots, a hash map uses a hash function. The bucket with the corresponding index is linked to its value. The key is distinct and unchangeable. Imagine a hash map as a cabinet with drawers that are labelled for the items inside of them. For instance, when storing user data, we can map values for the user, such as their first and last names, to a bucket by using their email address as the key.
Implementing a hash map relies primarily on hash functions. It accepts the key and converts it to the bucket index from the key. A different index should be generated by ideal hashing for each key. But collisions can happen. We can easily use a bucket for multiple values by appending a list or by rehashing when hashing yields an existing index.
Dictionaries are an illustration of hash maps in Python. To learn how to create and modify such data structures for search optimization, we'll see a hash map implemented from scratch.
Functions
The Hash Map we will be designing will have the Following functions:
- set(key, value): This will Insert a key-value pair into the hash map. If the value already exists in the hash map, this will update the value.
- get(key): This will returns the value to which the specified key is mapped, or “No record found” if this map contains no mapping for the key.
- delete(key): This will remove the mapping for the specific key if the hash map contains the mapping for the key.
Code Implementation
Let's Implement hashmap using dictionary in python.
Example 1:
Output:
Example 2:
Output:
Time Complexity
Hashing and memory index access both take a fixed amount of time. As a result, a hash map's search complexity is also constant time, or O(1).