Counter in Python
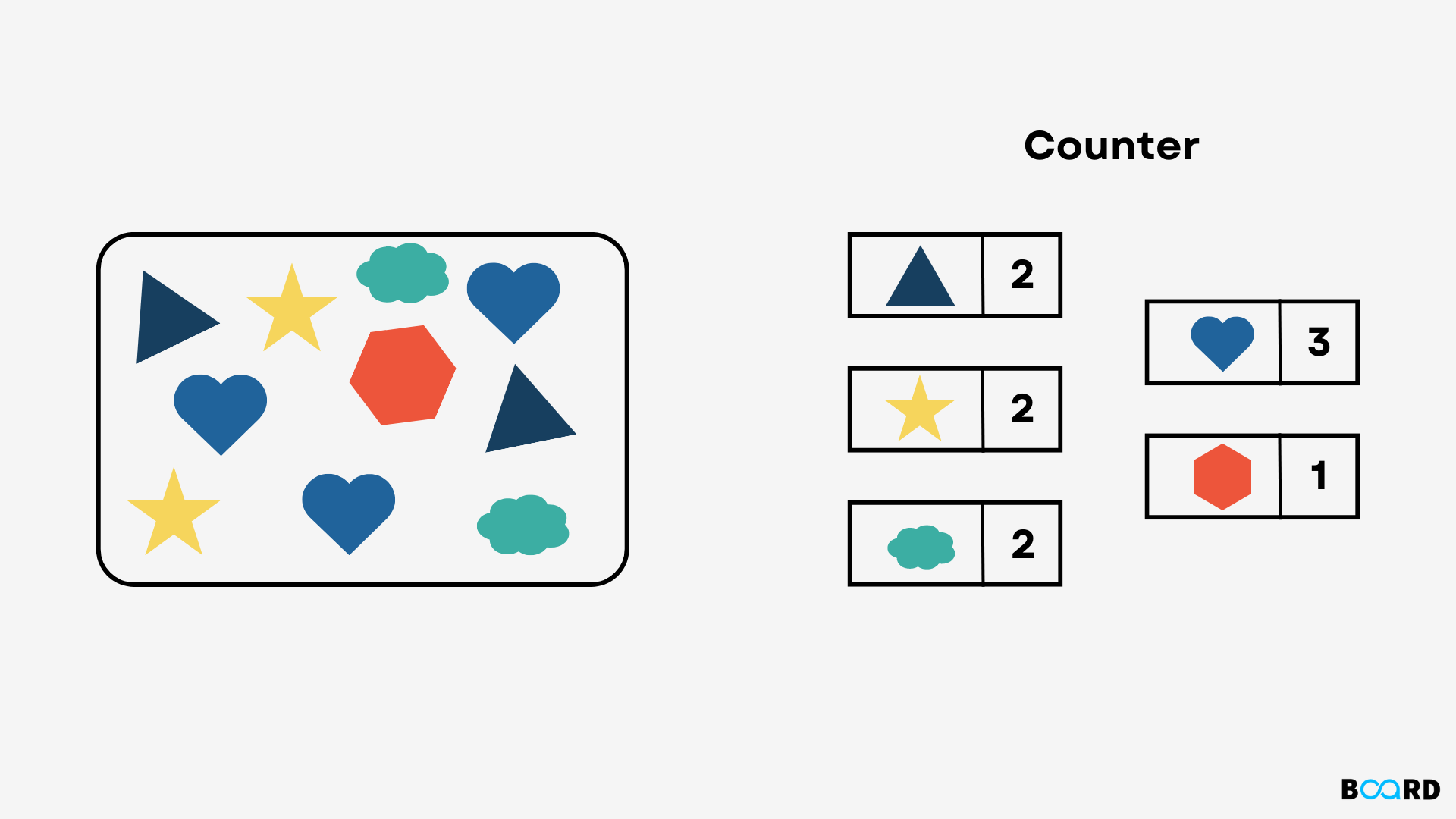
Introduction
In Python, when we use a container to store elements then the count of each element is stored in a counter. Counter helps us to access the object that is stored. The dictionary holds the Counter as a sub-class. It provides us with a way to count the key-value pairs in an object. Also known as Hash table objects. Some built-in containers are lists, tuples, dictionaries etc. They are included in the collection module in Python. It is a multiset of other languages.
Syntax
Initialization
In Python, we can call counters constructors and this can be done by any method below:
- By calling it with the sequence of items
- With Dictionary containing keys and values.
- With String
- With Keyword arguments mapping String names to counts.
Features of Counter
- In the unordered collection, the counter is used to store the data, similar to hashtable objects. The elements are represented as keys and the count as values.
- In an iterable list, it helps to count the number of elements.
- On the counter, we can do some arithmetic tasks such as addition, subtraction union etc.
- A counter can also count elements of another counter.
Implementation of Counters
In Python, it takes the iterable objects as arguments such as list, tuple etc and returns the count of elements in it.
For example, let us see the list below:
l = [1,2,3,4,5,5,3,2,1]
Now this list contains duplicate items. we will make use of a counter to count the number of elements. Let us see the code below.
Code 1
Output
Code 2
Output
Arithmetic Operation on Python Counter
As mentioned earlier, we can do arithmetic operations such as addition, subtraction union etc, on the container. Let us see the following example below:
Code
Output
Conclusion
- In Python, when we use a container to store elements then the count of each element is stored in a counter.
- Counter helps us to access the object that is stored.
- In the unordered collection, the counter is used to store the data, similar to hashtable objects. The elements are represented as keys and the count as values.
- Python takes the iterable objects as arguments such as list, tuple etc and returns the count of elements in it.