What are Collections in Python?
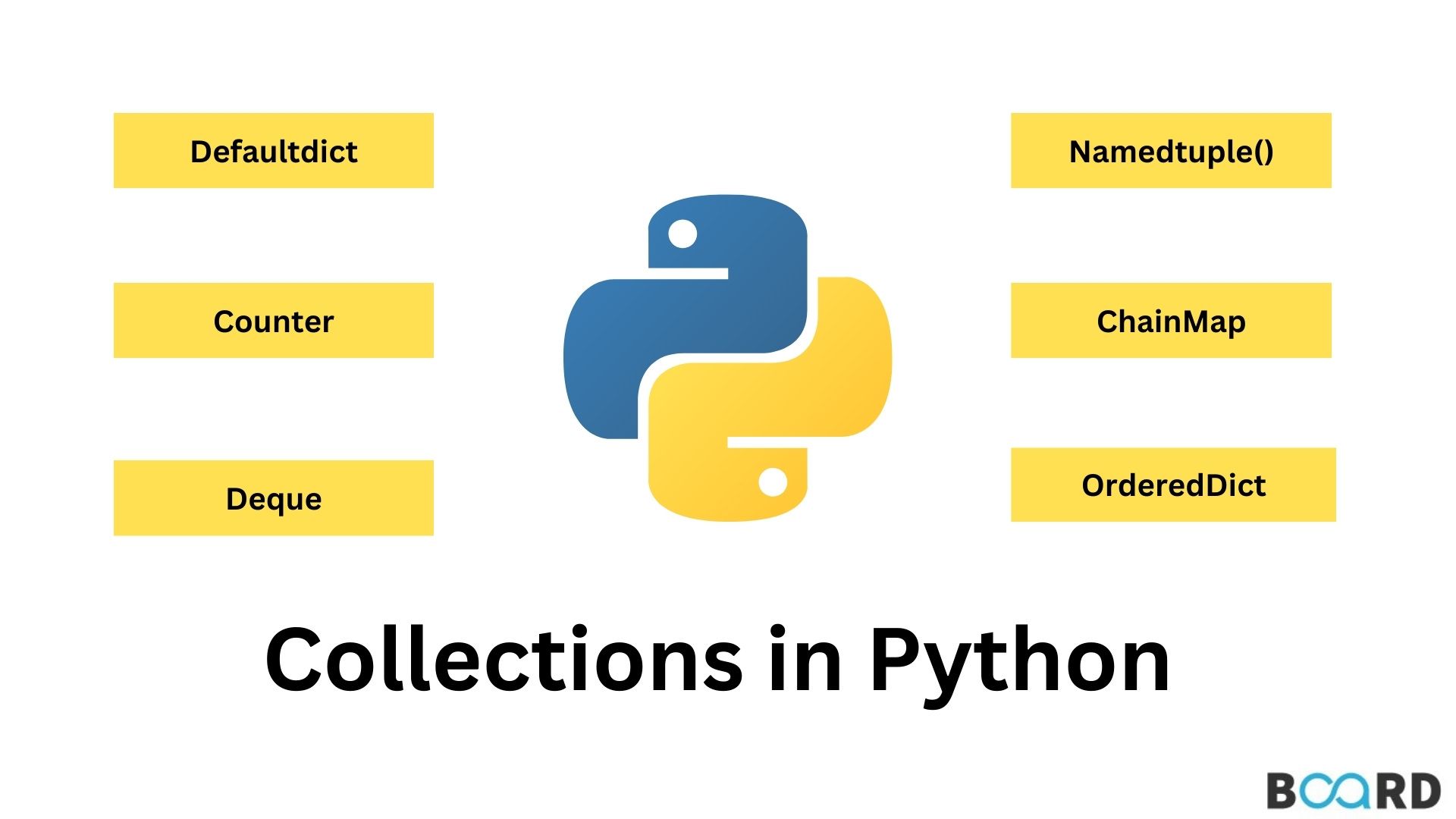
Introduction
Data structures like lists, tuples, arrays, dictionaries, etc. are examples of data structures that are usually referred to as collections in Python.
A built-in collections module in Python offers extra data structures for data collections.
The six most popular data structures are included in the collection modules.
Defaultdict
A dictionary in Python is just like Defaultdict. The only distinction is that when you attempt to access a key that doesn't exist, it doesn't throw an exception or a key error.
Even though the 4th index in the following code wasn't initialized, the compiler still gives us a value of 0 when we try to access it.
EXAMPLE :
OUTPUT:
Counter
Counter A built-in data structure known as a counter is used to track the frequency of each value in an array or list.
The following code counts how many times the value 2 appears in the provided list.
EXAMPLE:
OUTPUT :
Deque
Deque is the best form of a list for adding and removing items. It can add/remove items from either start or the end of the list.
The supplied list is being added to at the conclusion of the following code, and g is at the beginning of the same list.
EXAMPLE:
OUTPUT:
Namedtuple ()
A very significant issue in the world of computer science is resolved by the Namedtuple() function. Unlike namedtuple(), which returns names for each place in the tuple, regular tuples don't need to remember the index of each field of a tuple object.
In the code below, passing an attribute is sufficient to produce the desired output and does not require an index to print a student's name.
EXAMPLE
OUTPUT
ChainMap
ChainMap creates a list of dictionaries by combining numerous dictionaries. With no limitations on the number of dictionaries, ChainMaps essentially condenses several dictionaries into a single unit.
The next application, ChainMap, will give you back two dictionaries.
EXAMPLE
OUTPUT
OrderedDict
OrderedDict A dictionary that keeps its order is called OrderedDict. For instance, the order is maintained if the keys are put in a particular order. The location will not change even if you later modify the key's value.
EXAMPLE
OUTPUT