Mastering DBMS: Learn Basics to Advanced Technique
Natural Join in DBMS SQL
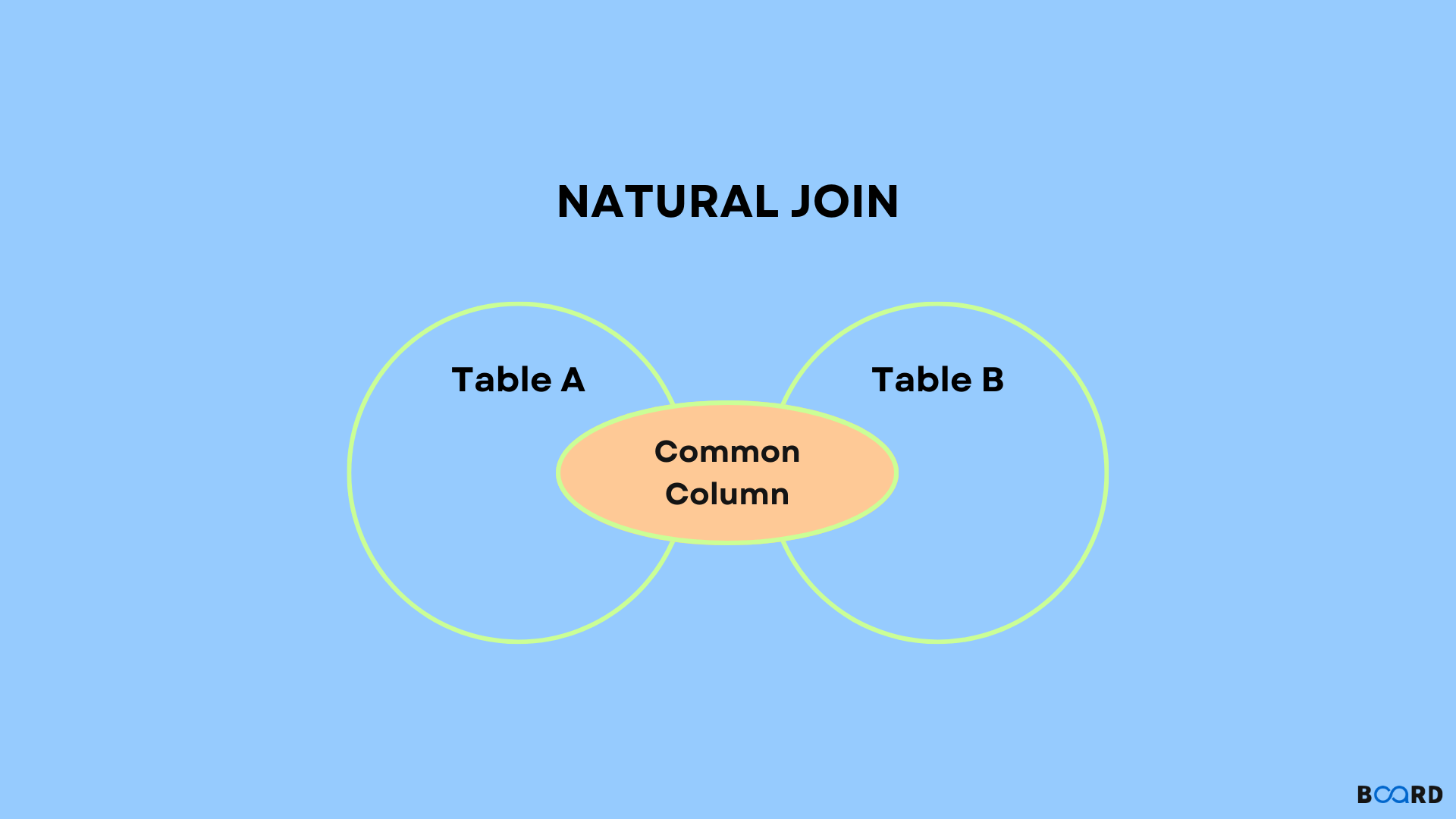
Introduction
In DBMS SQL, a natural join is a join operation that combines tables based on matching column names and their data types. It returns a result set that contains only the standard columns between the two tables, without including duplicate columns.
The primary functions of Natural Join are as follows:
- Combines data from two tables.
- Eliminates duplicate columns.
- Performs Cartesian product.
Syntax:
The syntax for combining 2 or more tables with the Natural Join technique is as follows,
SELECT column_name(s)
FROM table1
NATURAL JOIN table2;
In this syntax, table1 and table2 are the names of the tables you want to join, and column_name(s) are the columns you want to select from the joined table. When you use the NATURAL JOIN clause, SQL will automatically match the columns with the same name and data type in both tables.
Example:
Here is an example code in SQL for creating a database with two tables and performing a natural join operation. The code illustrates creating a database called “Board_Infinity”, a user table and orders table. Both the “user” and “orders” tables are joined by natural join.
Code Implementation
Output:
The NATURAL JOIN operation will join the two tables based on the columns with the same name and data type, which in this case is the "id" column of the "users" table and the "user_id" column of the "orders" table.
Conclusion
Natural joins can be less efficient than other types of joins, especially if the tables being joined have many columns or if the matching columns have many duplicates. In some cases, it may be more efficient to use an explicit join condition using the ON or USING clause, which allows you to specify the columns to join explicitly.