JavaScript Fundamentals: Learning the Language of the Web
A Quick Guide to VAR, LET and CONST
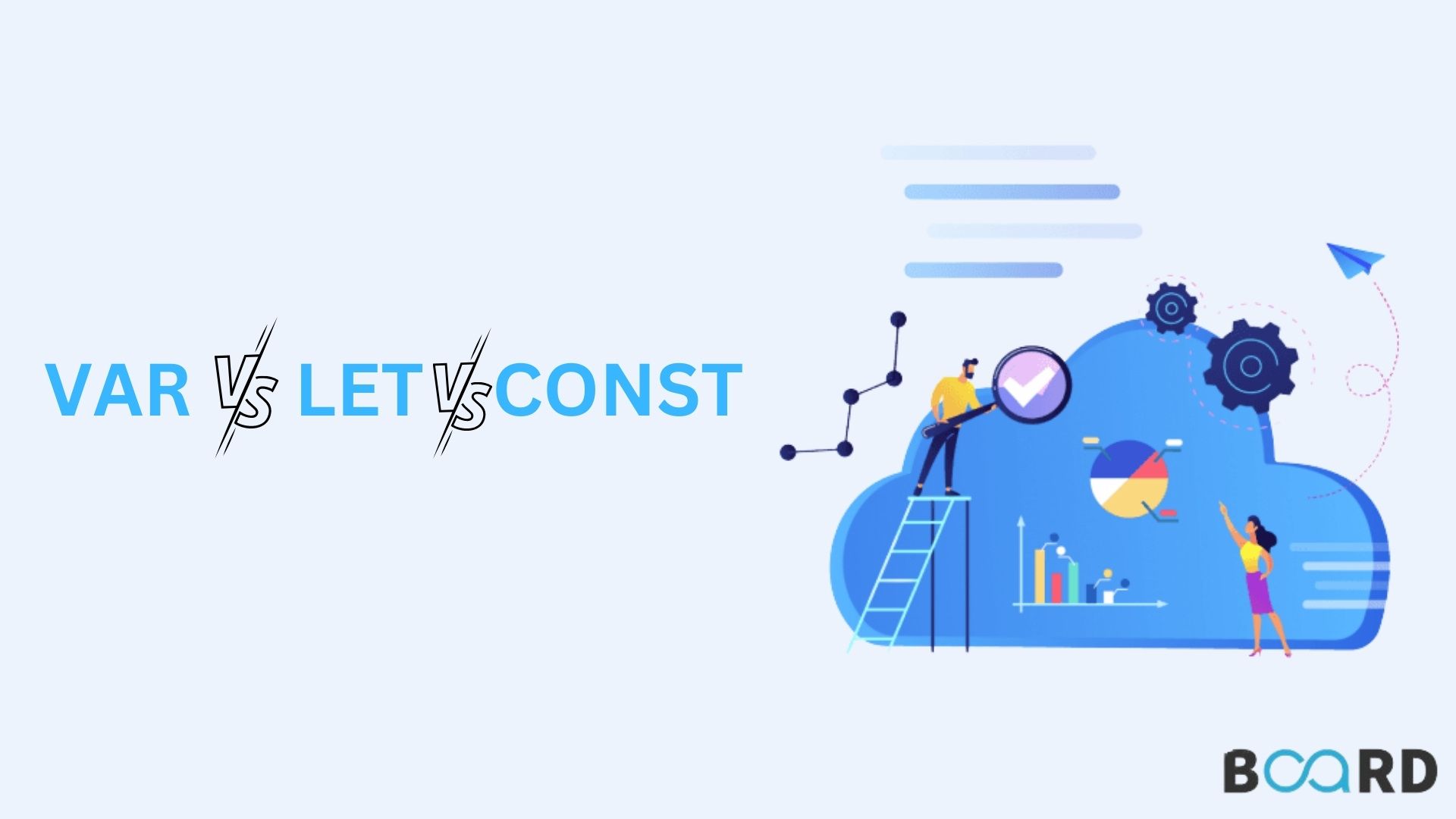
INTRODUCTION
The keywords for declaring variables in javascript are var, let, and const. A variable can be declared in javascript using the venerable Var variable method. We utilize the let and const variables in the current javascript, which was included in the ES2015(ES6) update; it is now used more commonly in modern javascript than the var variable.
VAR
Scope of Var
Scope basically refers to the area in which these variables can be used. Var declarations can be function/locally or globally scoped.
When a var variable is declared outside of a function, the scope is global. This means that any variable declared outside of a function block using the var keyword is accessible throughout the entire window.
When a variable is declared inside a function, it has function scope. This indicates that it is accessible and limited to that function.
Look at the example below for a better understanding.
Since greeter occurs outside of a function, it is globally scoped in this instance, whereas hello is function scoped. Therefore, we are unable to access the hello variable outside of a function. In that case, if we:
Because hello isn't accessible outside of the function, we'll receive an error.
Var variables may be modified and re-declared.
This indicates that we can accomplish this within the same scope without encountering a problem.
Even this:
Hoisting of var:
Before code is executed, variables and function declarations are hoisted to the top of their scope in JavaScript. Thus, if we carry out the following:
It can be interpreted as:
As a result, var variables are initialized with the value undefined and raised to the top of their scope.
LET
Let is now the preferred syntax for declaring variables. It shouldn't come as a surprise because it enhances var declarations. Additionally, it resolves the issue we just discussed with var. Let's analyze the reasons for this.
Scope of let
A block is a section of code that is bounded by. Curly braces house a block. A block is anything contained in curly brackets.
Consequently, a variable declared in a block with let can only be used in that block. Here's an illustration to help me explain.
We can see that using hello outside of its definition's block (the set of curly brackets) results in an error. Let variables be block-scoped, which explains why.
Unlike re-declaration, let can be changed.
A variable declared with let can have its scope modified, just like var can. A let variable, unlike var, is immutable within its scope. While doing so will work:
It gives an error.
However, there won't be an error if the same variable is specified in many scopes:
Hoisting of Let
Why is there not a mistake? This is so that the different scopes of the two instances can be regarded as separate variables.
Let is a superior option to var due to this feature. If you've used a name for a variable before, you don't need to worry while using let because a variable only exists within its scope.
Additionally, the earlier noted issue with var does not occur because a variable can only be declared once within a scope.
Let declarations be elevated to the top, just like var. The let keyword is not initialized, in contrast to var, which is initialized as undefined. Therefore, you will get a Reference Error if you attempt to utilize a let variable before the declaration.
CONST
Constant values are kept for variables specified using the const keyword. Let declarations and const declarations have some similarities.
The scope of const declarations is blocked.
Const declarations, like let declarations are only accessible within the block in which they were made.
It is impossible to modify or re-declare const.
This indicates that within the scope of a variable declared with const, its value will not change. It cannot be changed or declared again. As a result, if we declare a variable with const, we cannot:
Not even this:
Therefore, each and every const declaration must be initialized at the moment of declaration.
Hoisting of const
Const declarations are raised to the top, similar to let, but they are not initialized.
So, in case you missed them, here are the distinctions:
- While let and const are block-scoped, var declarations are either globally scoped or function-scoped.
- Let variables can be updated but not re-declared, const variables cannot be updated or re-declared, and var variables may both be updated and re-declared inside their scope.
- Each of them is raised to the very peak of its scope. However, let and const variables are not initialized, but var variables are initialized with undefined.
- Contrary to const, which needs to be initialized upon declaration, var and let can be defined without being initialized.
Conclusion
The keywords var, let, and const allow us to declare variables. The scope of a variable indicates where in our code we may and cannot access it. It is one of the key factors determining how let, var, and const vary in javascript. Before code execution, hoisting capabilities allow us to lift our variable and function declarations to the top of the scope. A variable can be declared with the sweet and innocent suffix var, which elevates it to the top of the scope. The current methods of declaring variables, which also get hoisted but weren't initialized, are let and const.