JavaScript Fundamentals: Learning the Language of the Web
JavaScript Split()
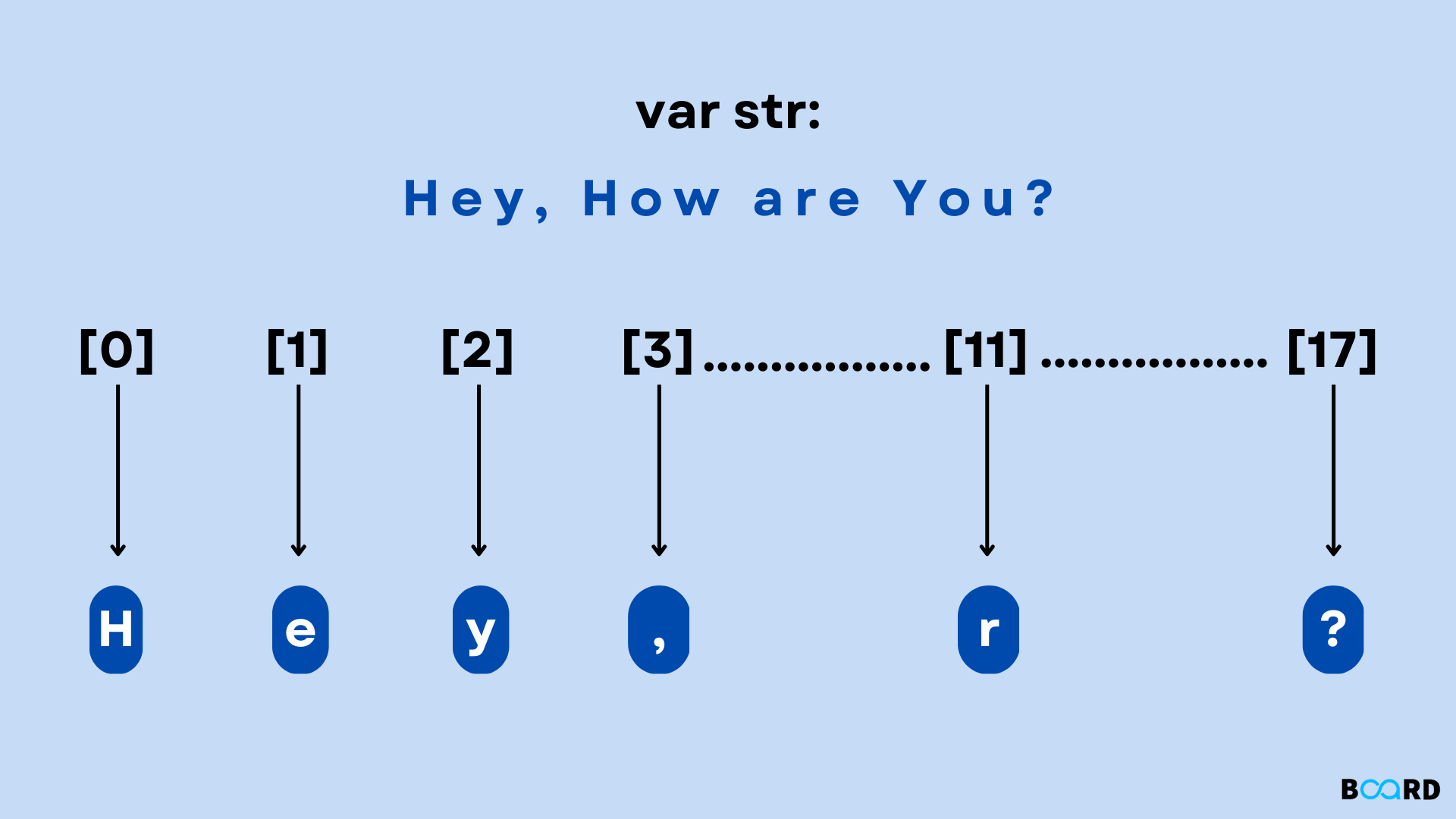
Introduction
The given string is divided into substrings using the defined separator provided in the argument when it is split using the JavaScript str.split() method. JavaScript offers us numerous string methods to change our existing string in accordance with our needs. One of them is the split() method. A string can be divided using JavaScript's Split function. In order to give us a newly formed array, it splits the string into an array of substrings. In other words, we can state that a separator must be given as an argument when using the split function to split a string. If we use the split method in JavaScript to modify a string value, it won't change the original string; instead, it will always give us the newly formed array or substring. If we don't pass anything in the split method argument, the string will be divided into its individual characters.
Syntax
Separator
It specifies the character or regular expression to be used to split the string. The entire string becomes one single array entry if the separator is left out. When the separator is absent from the string, the same thing likewise occurs. Every character in the string is split if the separator is an empty string ("").
limit: Defines the maximum number of splits that can be identified in the given text. The string is not reported in the array if it is left unchecked after the limit has been reached.
Return value: The function splits the given string at each place where the separator appears to create an array of separated strings.
How to does split() work in JavaScript?
Javascript's split() function requires two arguments, a separator and a limit. Both of these arguments are optional. When we provide the separator a value, it will first search the string for the value we give it before splitting the string when it finds a match. Additionally, regular expression can be passed to it. Regarding the second option, limit, it determines how many times we want our string to be spit out. It will choose how many times the string is iterated. Separator accepts a string as its first argument, from which we can choose to divide our original text. If the separator is missing from the string, only one element will be returned.
Example 1
Output:
Example 2
Output:
Example 3
In this illustration, the function split() splits str wherever the character "" appears to produce an array of strings. The second argument (2) only allows for two such divisions.
Output:
Conclusion
The JavaScript split() method is used to split up a string into an array of substrings.
The optional separator is a pattern that instructs the computer where each split should occur.A positive number serves as the optional limit argument, which instructs the computer how many substrings should be included in the array value returned.
So long as both of the parameters it requires are optional, we can split a string using a variety of symbols and regular expressions. As a result, their use must be based on necessity. Remember that it will always return as the new array, leaving the original string's value unchanged.