JavaScript Fundamentals: Learning the Language of the Web
String to Array conversion in JavaScript
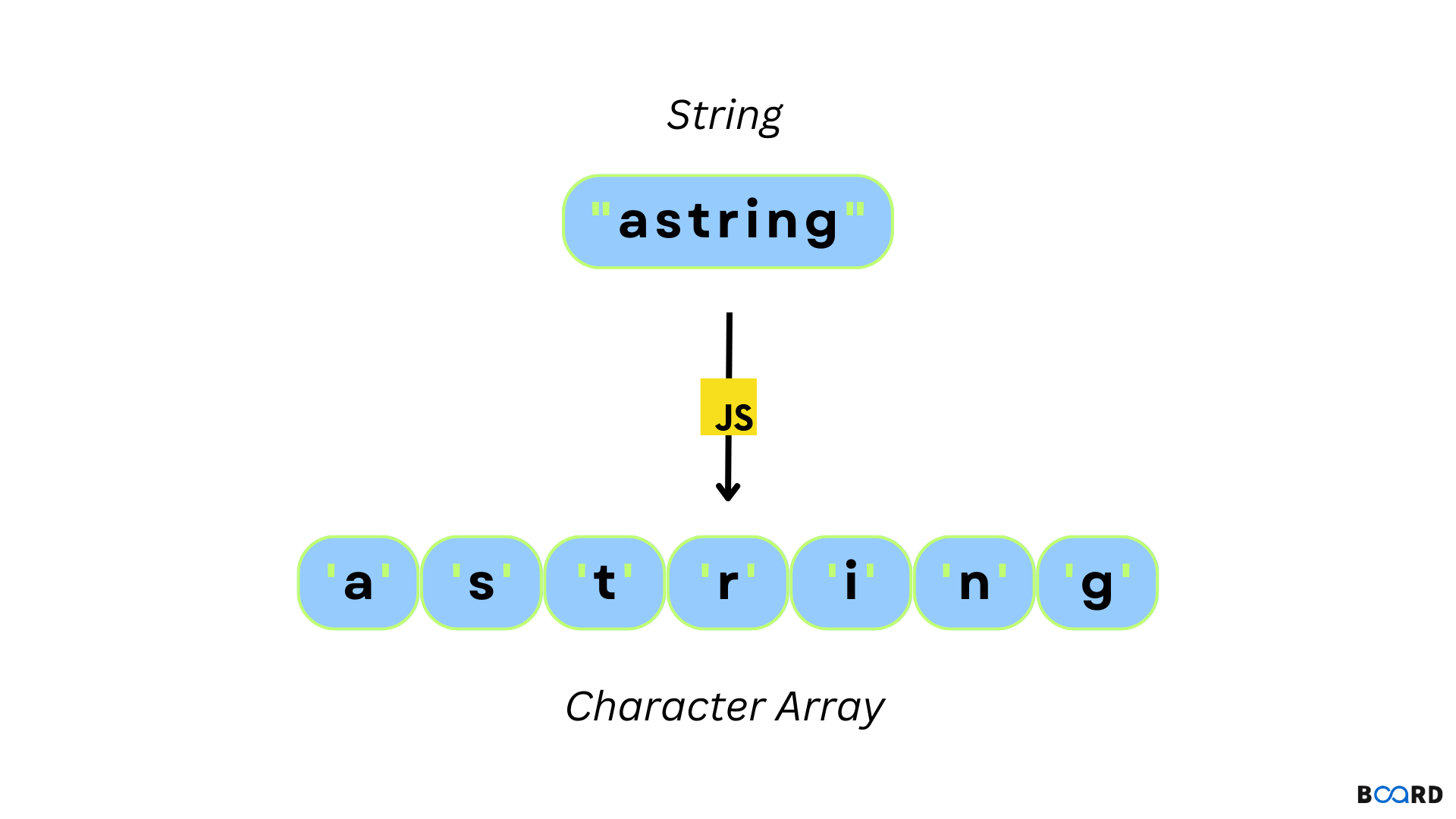
Introduction
we can use the split() and Array.from() functions in JavaScript to convert string into a character array
String split() Function in JavaScript
String.split() is a built-in function in JavaScript that lets you split a string into an array of strings, with each item representing one word in the original string. The delimiter can be any character or sequence of characters that separates words in the input string, such as whitespace or punctuation. The String.split() method is used to break a string into an array of strings by splitting it on a given delimiter, which is specified as the argument to the method.
Syntax
The split() function is a useful method that splits strings into an array of substrings. It takes one argument, a string to be split, and returns an array of the substrings.
Working
This is how it works:
1. A string is passed as the first argument to the split() function.
2. The string is then split on whitespace (spaces, tabs, newlines) using a regular expression defined by the second argument.
3. If there are more than two matches for this regular expression, then only the first two matches are used in splitting the string; any additional matches are ignored and do not contribute to the result of that call to split().
The following example uses the String.split() method to break up a sentence into words and store them in an array:
Output:
This would result in an array with two elements, containing the words " Hello" and " World”.
Example:
Output:
Array.from() function
The Array.from() function in JavaScript is a new way to create arrays from other types of data. It is not the same as the array literal syntax which creates an array with a set of comma-separated values.The Array.from() function can be used to convert an existing object or value into an array, or it can be used to create a new array with a given set of values. Array.from() is a static method of the Array class and accepts an iterable as its argument. It converts the iterable into an array, without changing the original object. The result of this conversion will have an identical shape to the original iterable and have all of its elements converted to values in the appropriate positions of the array according to their type properties.
Syntax
The Array.from() function in JavaScript takes a list of values and returns a new array with the same number of elements as the original list, but with the values replaced by their index within the original list.
If you have a list of integers and want to return an array whose elements are those integer values, you can use this function:
Array.from( [1, 2, 3] )
This will give you:
[1, 2, 3]
The returned array has the same length as the original one and contains each entry exactly once--there are no duplicates!
Example:
Output:
Conclusion
String split() is a string method that splits a string into an array of strings. The method returns an array of strings, each containing a substring of the original string and the Array.from() function can take any iterable object, such as an array, generator, or string of space-separated values. It converts the object into a new array and returns new array.