JavaScript Fundamentals: Learning the Language of the Web
Map in JavaScript
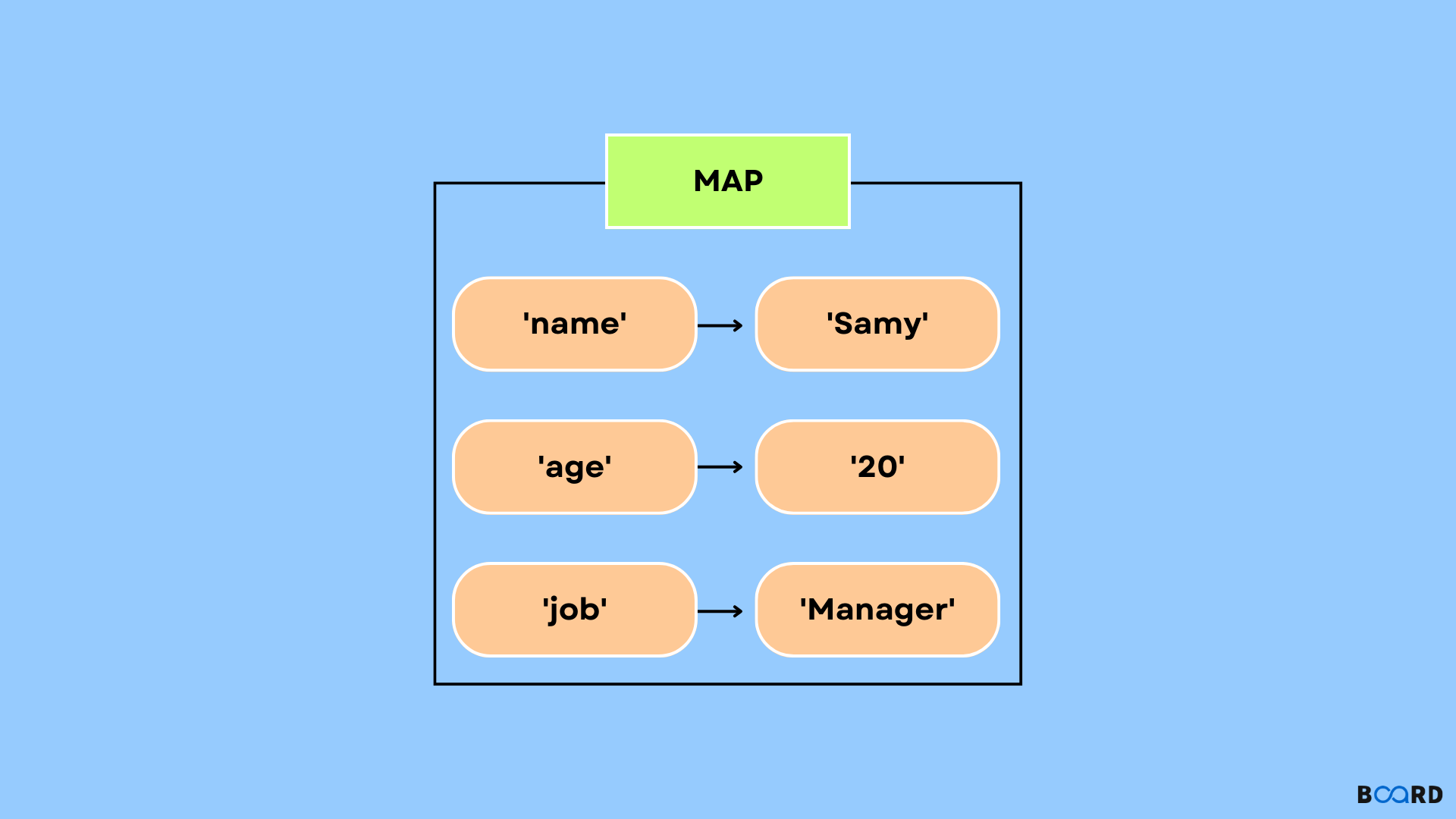
Introduction
Map is a collection of key data items, Same as Object. A map is a data structure that stores key-value pairs and allows you to look up the value associated with a given key. Each key belongs to a data value, and the values are stored in an array. You can access a value by using its associated key as a string, or by using array syntax to index it.
Important:
The keys are not necessarily unique, so you can have multiple maps with the same key.
Map is an associative array, which means that it can be indexed by either the key or the value. The values in a map can be of any type, including other maps or sets. Unlike arrays, there is no limit on how many items can be stored in a map, as long as you have enough memory available to accommodate all of them. Maps also allow more than one item with the same key in them - this is called "chaining".
Syntax:
Parameter:
N -- Iterable object whose values are stored as key, value pair, If the parameter is not specified then a new map is created is Empty.
Properties:
map.set(key, value) - set the value for the key in the map object.
map.get(key) - returns the value associated with the key, undefined if key doesn’t exist in map.
map.has(key) - returns true if value associated with the key exists, false otherwise.
map.delete(key) - removes the value by the key.
map.clear() - removes all elements from the map.
map.size - returns the count of the elements .
map.values() - returns a new iterator object that contain values for each element in insertion order.
map.keys() - returns a new iterator that contain the keys for element in insertion order.
Example 1
Output:
Example 2: using map object
Output:
Conclusion
A map is an object with a set of key-value pairs. The keys are strings, and the values can be any type. Keys are unique, meaning that no two keys should have the same value within the map. The values can be any type, but they must match the corresponding key's type for example, if you have a string as your key and an integer as your value, you will need to convert the integer to a string before inserting it into the map.