JavaScript Fundamentals: Learning the Language of the Web
Understanding Anonymous Function in Javascript
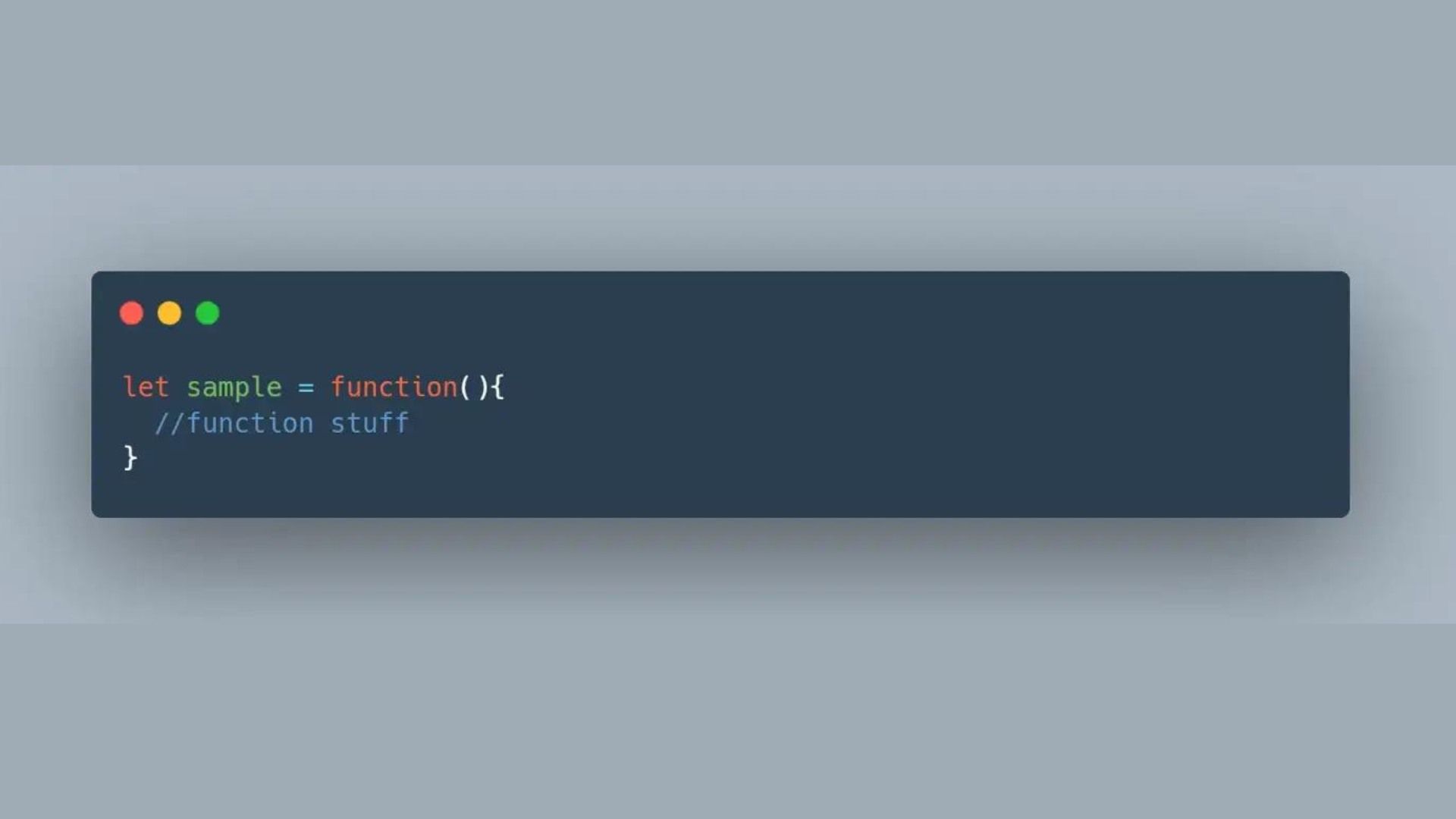
Introduction
The definition of the term "anonymous" is "unknown or without identification." An anonymous function in JavaScript is a function that has no name, or more precisely, one that lacks a name. An anonymous function has no identification when it is created. The distinction between a regular function and an anonymous function is this. Not just in JavaScript but in various other computer languages as well. An anonymous function serves the same purpose.
Every time a function is called, a series of instructions are carried out, and the function responds by returning a specific result. The specific syntax is used to define functions; for instance, the function keyword is followed by the function name and parentheses in JavaScript. The syntax is the only difference between Anonymous and similar functions.
The anonymous function in JavaScript is not named when declared and is typically supplied as an argument to other higher-order functions. The anonymous function is created and utilized as a parameter for immediate function execution within a function.
Code Example
Output -
Welcome to Board Infinity!
Code Explanation
Here, a normal function called greet() has been constructed. It describes the distinction between a regular function and an anonymous function. We have finally called the newly generated function. Thus, the actual implementation of an anonymous function is completed in this manner.
Use of Anonymous Functions in JavaScript
The anonymous function in JavaScript has a variety of uses. Below are a few of them:
- Supplying an anonymous function as a parameter to another function
- An anonymous function may also be used as a parameter for another function. Let's write some code to provide the anonymous function as a value for an argument to another function to understand better:
Key Takeaways
- Functions that are anonymous lack a name.
- We may do so when writing an anonymous function by utilising the function keyword and parentheses ().
- Inside the curly parenthesis, we can write the function statements exactly like we would for any other javascript function.
- The output of an anonymous function is saved in variables.
How to reuse the Anonymous Function
Anonymous functions in JavaScript can be utilized again in the future. Make them reusable by assigning them to a variable and then calling them wherever you want. Let's look at the example below to grasp how we might utilize anonymous functions in the future.
Output -
This is an anonymous function
Since there is no name between the function keyword and parenthesis in the example above, it is entirely anonymous. However, we are assigning this anonymous function to the AnonymousFunc variable because we want to be able to call it in the future.
Immediately Invoked Function Execution
To avoid invoking a function, we sometimes need to run it immediately after declaring it. Since they start working as soon as they are stated, anonymous functions are a fantastic choice.
An instantaneously invoked JavaScript function is called an IIFE (Immediately Invoked Function Expression). Simply put, it begins to operate as soon as it is defined. It allows functions to be instantly executed when they are generated.
Syntax
Conclusion
When an anonymous function is created, it has no name and can be called anonymously. The anonymous function can execute a function or give another function a parameter. This article has covered how to construct anonymous functions and save them for use at a later time.