JavaScript Fundamentals: Learning the Language of the Web
Array iteration in JavaScript
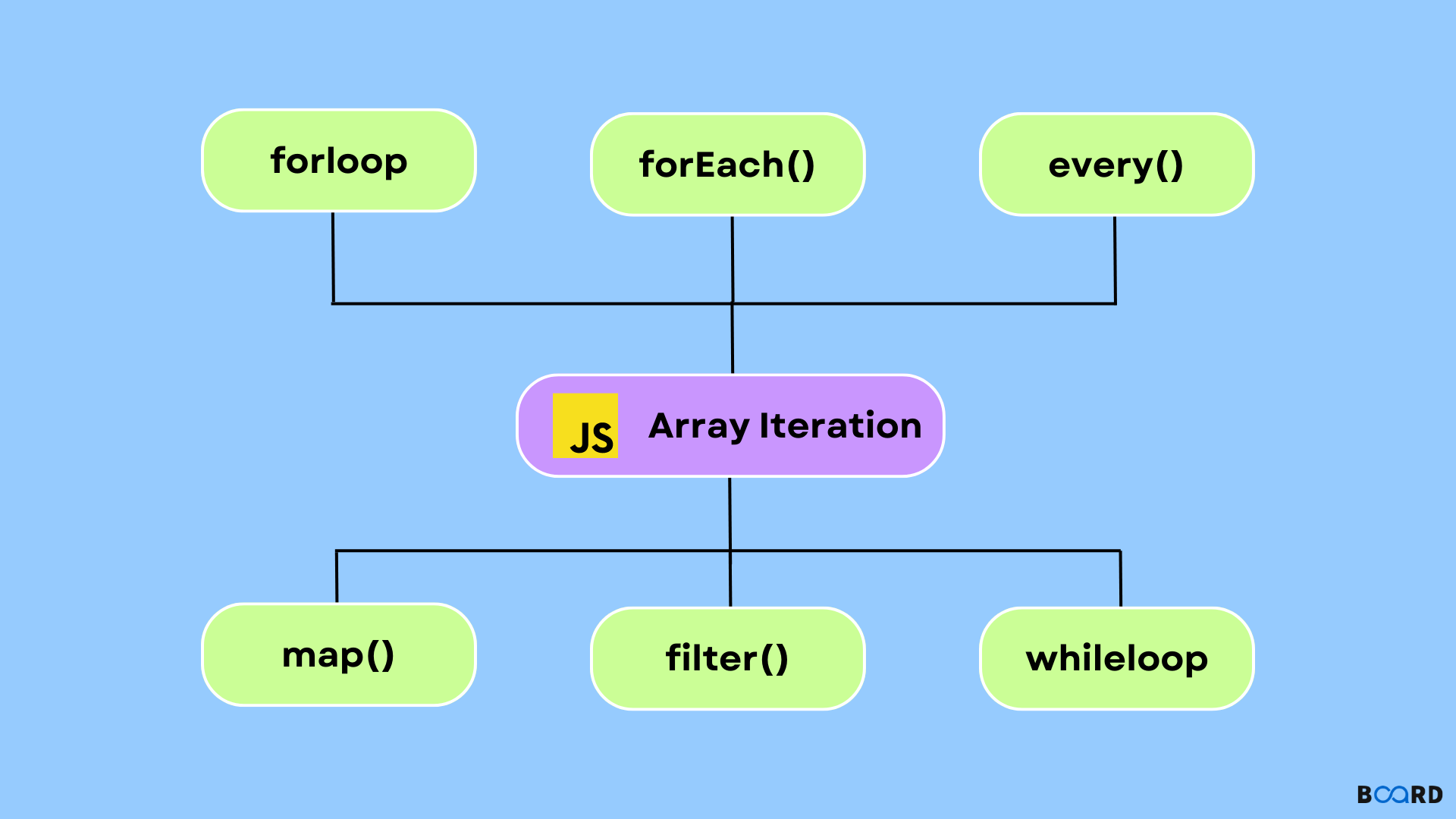
Introduction
Array iteration in JavaScript can be done by either for loops or with the help of the built-in array methods like map() and filter(). Array iteration is a way to loop through an array and apply a function to each element in the array.
To iterate over an array, you need to first create that array. To do this, you'll use the Array method's init() method. This method takes two arguments: the size of the array, and another argument for how many times to repeat the operation (which defaults to 1).
The code below uses this method to create an empty array called myArray:
myArray = [];
myArray[0] = 42; // Set the first element in myArray to 42
myArray[1] = "hello"; // Set the second element in myArray to "hello"
myArray[2] = 3.1415; // Set the third element in myArray to 3.1415
Example:
Output:
Let's take a look at an  example of how array iteration works in JavaScript.
We'll start with an array containing two numbers:
var A = [1, 2];
Then we'll iterate through the array and print out each value by itself:
for (var i = 0; i < A.length; i++) { console.log(A[i]); }
This method is called an array iteration because it loops through each element of the array. The variable i will refer to each element in the array and be equal to its index value in that element.
There are multiple ways one can iterate over an array in Javascript.
Using for loop
Output:
Using while loop
Output:
using forEach method
Output:
Using every method
Output:
Using map
Output:
Using Filter
Output:
Conclusion
The array iteration in JavaScript is a way to iterate through an array, which means to enumerate the elements of that array. Array iteration is a common operation in JavaScript that allows you to iterate through an array in a loop. Each time you call the function, it will return a new element from the array.
Array iteration is a method of accessing the elements of an array. The first step is to create a new array, which will be used as the source of our iterating data. We then set up variables that will hold each element in our new array. Then we loop over the new array, calling the function on each element.