JavaScript Fundamentals: Learning the Language of the Web
Array Join() in JavaScript
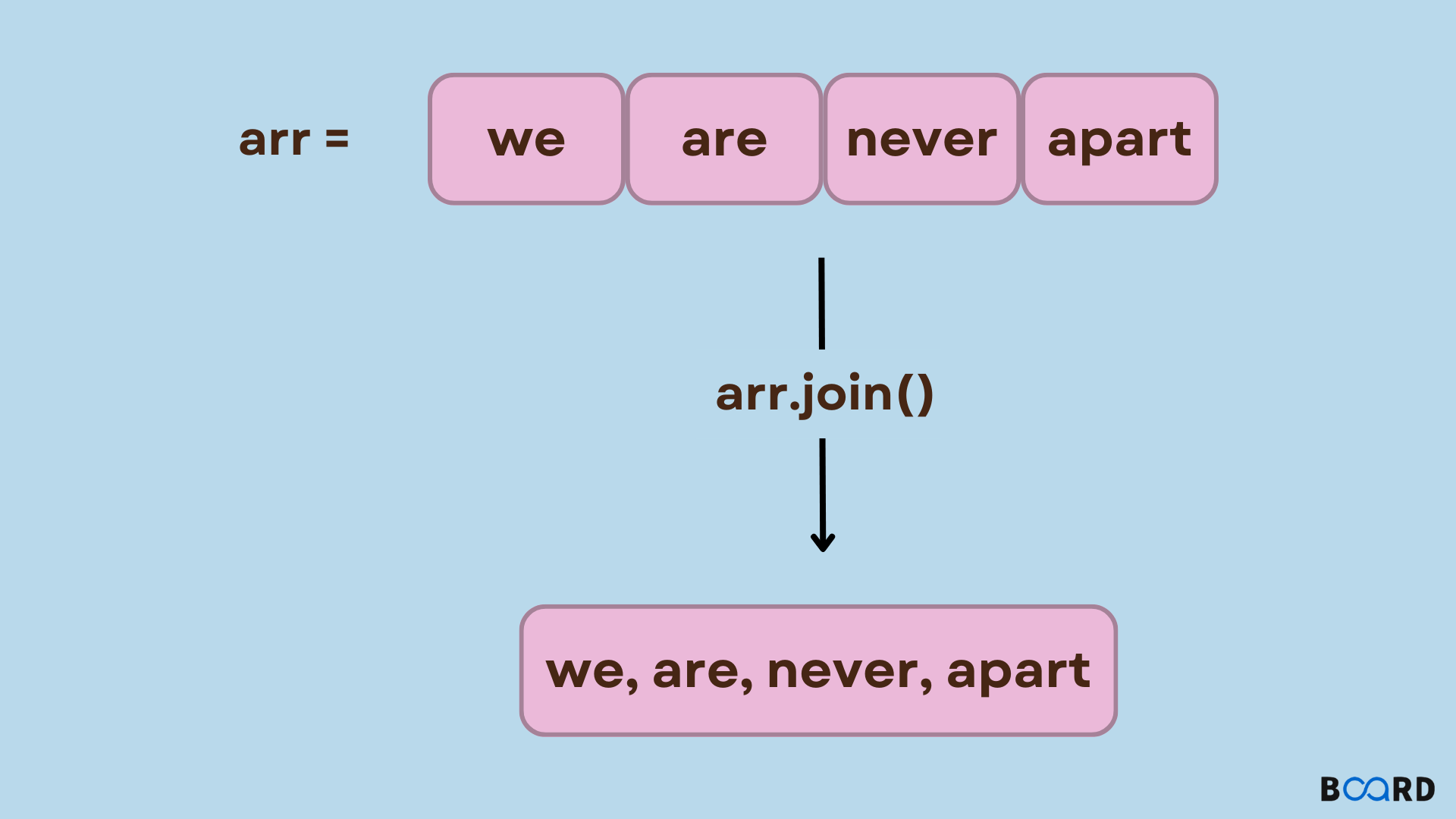
Introduction
The array elements in an array, each separated by a specific separator, are concatenated together using the join() method to produce a new string. The join() method concatenates all of the elements in an array (or an array-like object), separated by commas or a specified separator string, and returns a new string. If there is only one element in the array, the separator will not be used when returning the element.
Syntax:
Parameters: This method accept single parameter as mentioned above and described below:
separator: It is optional, meaning that it may or may not be used as a parameter. The comma is its default value (, ). specifies a string to be used to divide up each pair of adjacent array members. If required, the separator is changed into a string. The array's elements are separated by commas if it is left out (","). All items are put together without any characters in between them if separator is an empty string.
Return Value: The collection of elements from the array is returned as a String. VBy employing the separator to link each element of the array, this function returns a string. The comma (,), which is this function's default separator, is used to combine the array items if no separator is specified. The elements are connected directly with no characters in between them if the separator is an empty string. This function returns an empty string if the array is empty.
Note that the join() method converts undefined, null, and empty array [] to an empty string.
Description
All array items' string conversions are combined into a single string. The string "null" or "undefined" is replaced with an empty string if an element is undefined or null.
Internally, Array.prototype.toString() with no arguments calls the join function. An array instance's function toString() { [native code] } behaviour will also be overridden if join is overridden.
The join() method iterates empty spaces as though they have the value undefined when applied to sparse arrays.
The generic join() method is used. Only the length and integer-keyed characteristics are required for this value.
Examples
Example 1: In this case, the function join() uses the symbol “|” to unite the array’s items into a string.
Output:
Example 2: Using the symbol “,” the function join() unites the array’s members into a string (empty string).
Output:
Example 3: The functions joins array elements using ‘and’
Output:
Using four distinct methods to join an array
The example that follows creates an array called a with three members before joining it four times with the default separator, a comma, a space, a plus sign, and an empty string.
Using join() on sparse arrays
join() generates an additional separator and treats empty slots as if they were undefined.
Calling join() on non-array objects
The join() method first accesses each integer index and then reads the length property of this.
Conclusion
We can use the JavaScript Array join() method to concatenate all elements of an array into a string separated by a separator.The output is a string and the input is an array. The initial array is unaltered.If a separator has been specified, it is used to divide the array's elements; otherwise, JavaScript uses a comma as the separator by default for join.