JavaScript Fundamentals: Learning the Language of the Web
Object.keys() in JavaScript
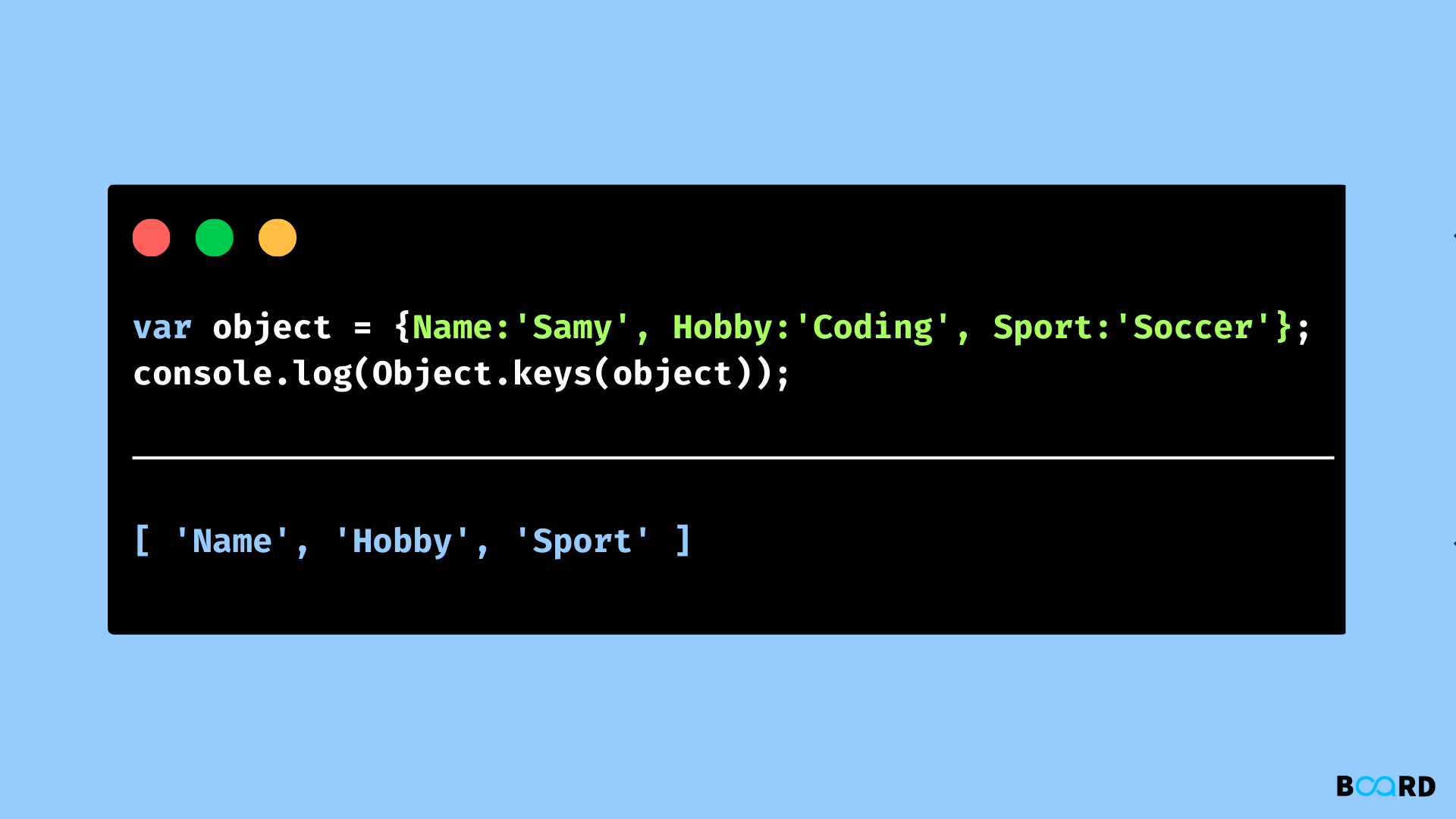
Object and object constructors in javascript
Comparable to other programming languages, JavaScript features the concept of objects and constructors that function much in the same way and may carry out similar kinds of actions. An object in JavaScript is a collection of unrelated data in the form of "key: value" pairs, either of primitive or reference types. In the context of an object, these keys, which can be variables or functions, are referred to as properties and methods, respectively.
There are two sorts of constructors: built-in constructors (such as array and object constructors), which are used with the new keyword, and custom constructors (defined properties and methods for specific objects). By employing the Object Constructor function, constructors can be used to generate an object "type" that can be reused repeatedly without having to redefine the object each time. Constructor names are typically capitalised to set them apart from conventional functions.
Object.keys() Method
The enumerable properties located directly on an object are represented as strings in an array that is returned by the Object.keys() method. The properties are applied in a loop with the same ordering as that provided by the object manually. An array of strings representing every one of the enumerable properties of the provided object is returned by the function Object.keys(), which accepts the object as an argument whose enumerable properties are to be returned.
The array returned by Object.keys() contains strings that correspond to the enumerable attributes that may be found directly on the object. The order of the properties is the same as what would result from manually looping over the object's properties.
Syntax
Parameter
Obj: The object whose enumerable properties are to be returned is referred to as obj.
Return Value: It provides an array of strings that represent each of the given object's enumerable attributes.
Applications include returning enumerable attributes of a basic array, an object that resembles an array, and an object that resembles an array with random key ordering.
Examples of Object.keys()
Example 1
In this example, an array called "check" contains the object as well as three property values ('a', 'b', and 'c'). The array's enumerable properties are returned using the keys() method. The ordering of the properties is the same as what was manually specified for the object.
Output:
Example 2
In this example, an object called "check" that resembles an array has three property values: "a," "b," and "c," along with the object itself. The array's enumerable properties are returned using the keys() method. The ordering of the properties is the same as what was manually specified for the object.
Output
Example 3
In this illustration, an object called "check" that resembles an array with three randomly ordered property values: 79: "a," 34: "b," and 89: "c." The array's enumerable attributes are returned by the keys() method in ascending order according to the index value.
Output:
Object Values in JavaScript
In contrast, a value may be any data type, such as an array, an integer, or a boolean. String, integer, boolean, and array are the types of values in the aforementioned example.
A function can even be used as a value, in which case it is referred to as a method. An example is the object's sounds() method.
Conclusion
In this article, we understood Object.keys() function with examples.